将yolov5检测运行结果显示在pyqt界面上
时间: 2024-03-04 19:48:00 浏览: 212
要将 YOLOv5 的检测运行结果显示在 PyQt 界面上,可以按照以下步骤进行:
1. 在 Pytorch 中加载 YOLOv5 模型,并使用该模型进行图像检测。
2. 将检测结果转换为 PyQt 中的图像格式,例如 QImage。
3. 在 PyQt 界面上添加一个图像显示控件,例如 QLabel。
4. 将转换后的图像显示在 QLabel 控件中,实现检测结果的显示。
下面是一个简单的实现示例:
```python
import sys
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QLabel, QMainWindow
import torch
import cv2
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords, plot_one_box
from utils.datasets import letterbox
from utils.torch_utils import select_device
class YOLOv5Detector:
def __init__(self, weights, device='cpu'):
self.device = select_device(device)
self.model = attempt_load(weights, map_location=self.device)
self.img_size = self.model.img_size
self.conf_thres = 0.4
self.iou_thres = 0.5
def detect(self, img):
# Preprocess input image
img0 = img.copy()
img = letterbox(img, new_shape=self.img_size)[0]
img = img[:, :, ::-1].transpose(2, 0, 1)
img = torch.from_numpy(img).float().div(255.0).unsqueeze(0).to(self.device)
# Run YOLOv5 model
pred = self.model(img)[0]
pred = non_max_suppression(pred, self.conf_thres, self.iou_thres)[0]
# Postprocess detection results
if pred is not None and len(pred):
pred[:, :4] = scale_coords(img.shape[2:], pred[:, :4], img0.shape).round()
for *xyxy, conf, cls in reversed(pred):
label = f'{self.model.names[int(cls)]} {conf:.2f}'
plot_one_box(xyxy, img0, label=label, color=(0, 255, 0), line_thickness=3)
# Convert output image to QImage
height, width, channel = img0.shape
bytes_per_line = width * 3
qimg = QImage(img0.data, width, height, bytes_per_line, QImage.Format_RGB888)
return qimg
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# Create detector instance
self.detector = YOLOv5Detector('yolov5s.pt')
# Create QLabel for image display
self.image_label = QLabel(self)
self.setCentralWidget(self.image_label)
# Load input image and run detection
self.image = cv2.imread('input.jpg')
self.detected_image = self.detector.detect(self.image)
# Display detection result
self.image_label.setPixmap(QPixmap.fromImage(self.detected_image))
self.setWindowTitle('YOLOv5 Detector')
self.setGeometry(100, 100, self.detected_image.width(), self.detected_image.height())
if __name__ == '__main__':
app = QApplication(sys.argv)
mainWindow = MainWindow()
mainWindow.show()
sys.exit(app.exec_())
```
在上面的代码中,我们首先定义了一个 `YOLOv5Detector` 类,用于加载 YOLOv5 模型并进行图像检测。在 `detect` 方法中,我们首先对输入图像进行预处理,然后运行 YOLOv5 模型,最后对检测结果进行后处理,并将结果转换为 QImage 格式。
在 `MainWindow` 类中,我们创建了一个 QLabel 控件,将检测结果显示在该控件中。在构造函数中,我们首先创建了一个 YOLOv5Detector 实例,然后加载输入图像并运行检测,最后显示检测结果。注意,在设置 QLabel 控件的大小时,我们使用了检测结果的宽度和高度。
运行上面的代码,可以看到 YOLOv5 的检测结果已经显示在 PyQt 界面上了。
阅读全文
相关推荐
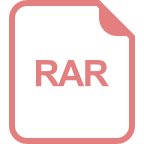
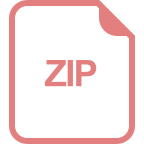
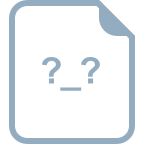
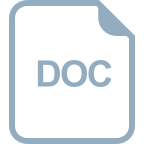
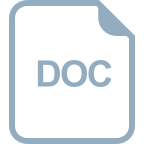
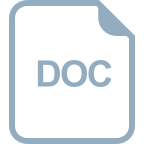
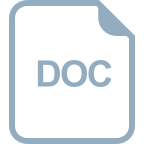
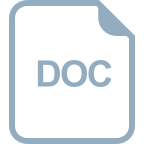
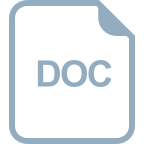
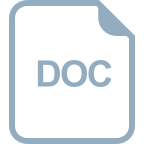
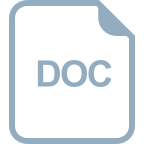
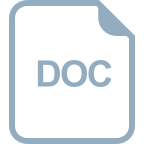
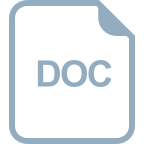
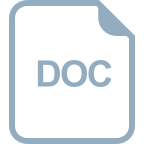
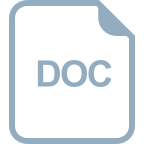
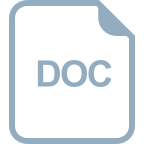
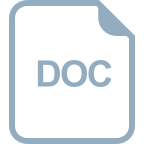
