如何在编程中为复数类型定义并实现加法 (+) 和减法 (-) 运算符,以便正确执行例如 (6+7i) + 7, (6+7i) - 7, 7 + (6+7i), 和 7 - (6+7i) 的操作?用C++
时间: 2024-12-25 11:23:59 浏览: 5
在C++中,我们可以使用类来模拟复数,并为其自定义加法和减法运算符。首先,我们定义一个名为`Complex`的类,包含两个成员变量表示实部和虚部。然后,我们将加法和减法定义为成员函数:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 加法运算符 overload
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 减法运算符 overload
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
// 打印复数
void print() const {
cout << real << " + " << imag << "i" << endl;
}
};
int main() {
Complex c1(6, 7); // 6 + 7i
Complex c2(7, 0); // 7 + 0i
// 示例计算
Complex sum = c1 + c2; // (6+7i) + 7
Complex difference = c1 - c2; // (6+7i) - 7
cout << "Sum: ";
sum.print();
cout << "\nDifference: ";
difference.print();
Complex d1 = 7; // 7 + 0i
Complex subtrahend = c1; // 6 + 7i
Complex subtraction = d1 - subtrahend; // 7 - (6+7i)
cout << "\nSubtraction: ";
subtraction.print();
return 0;
}
```
在这个例子中,我们通过`operator+`和`operator-`重载了`+`和`-`运算符,使得可以直接对`Complex`类型的对象进行加减操作。
阅读全文
相关推荐
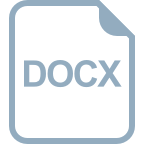
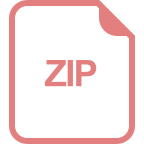
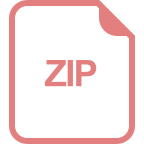

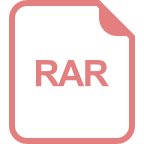
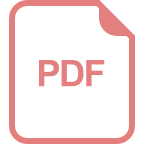
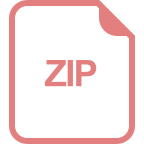
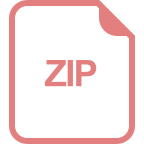
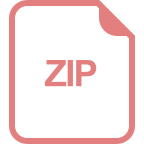
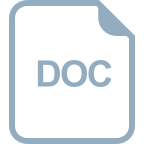
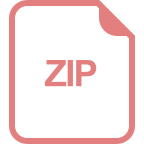
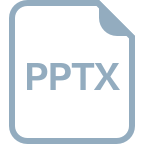
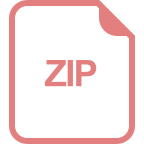
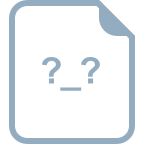
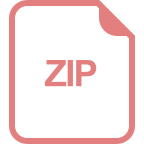
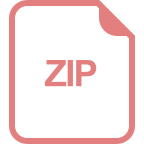
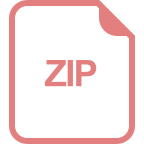
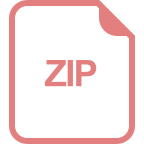
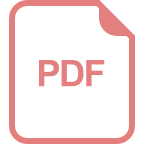