result = re.split(".", text.strip())
时间: 2024-04-17 15:26:52 浏览: 19
在正则表达式中,点号`.`是一个特殊,表示匹配任意字符(除了换行符)。因此,如果您想使用点号进行拆分,您需要进行转义,即使用`\.`来表示匹配实际的点号。
另外,`re.split()` 函数的第一个参数应该是正则表达式模式,而不是字符串。所以,您可以将 `.` 转义并传入正则表达式模式,然后将文本作为第二个参数传递给 `re.split()` 函数。
以下是修改后的代码示例:
```python
import re
text = "example.text"
result = re.split("\.", text.strip())
print(result)
```
在上述示例中,我们使用 `re.split()` 函数将文本根据点号进行拆分,并打印结果。
请注意,`strip()` 函数用于去除文本两端的空格和换行符。这是为了确保不会因为空格或换行符导致拆分结果不准确。
希望这可以帮助您实现文本的拆分。如果您有任何其他问题,请随时提问。
相关问题
解释以下代码:# 解析HTML页面 soup = BeautifulSoup(driver.page_source, 'html.parser') games = soup.find_all('a', {'class': 'search_result_row'}) # 遍历每个游戏,并获取所需信息 for game in games: game_name = game.find('span', {'class': 'title'}).text release_info = game.find('div', {'class': 'search_released'}).text release_year = release_info.strip().split(' ')[0] release_date = release_info.strip().replace(' ', '') review_info1 = game.find('div', {'class': 'search_reviewscore'}) if review_info1 is not None: review_info = review_info1.find('span', {'class': 'search_review_summary'}).get('data-tooltip-html') reviews = review_info[:4] positive_reviews = re.search(r'此游戏的 (\d+(,\d+)*) 篇用户评测中', review_info).group(1).replace(',', '') positive_review_percentage = re.search(r'评测中有 (\d+)% 为好评', review_info).group(1) + '%' else: pass
这段代码是使用 BeautifulSoup 库解析 HTML 页面,并从中获取游戏相关信息。具体来说,它首先使用 driver 获取当前页面的源代码,然后使用 BeautifulSoup 进行解析。接着,它使用 find_all 方法找到所有 class 为 'search_result_row' 的 a 标签,也就是游戏的链接。然后,对于每个游戏,它通过 find 方法找到游戏名称和发布信息,进一步处理发布信息以获取发布年份和日期。如果游戏有评测信息,它会从中提取出好评率和用户评测数量等信息。最后,如果游戏没有评测信息,则跳过该游戏。
7-2 请用正则表达式实现,多行字符串处理 分数 60 作者 聂作先 单位 福建工程学院 有一段多行的英文文本,其中有的行内有单词连续重复了2次,编写程序检查重复的单词并输出重复的单词及其所在的行的内容。 输入格式: 第一行为字符串的总行数n,后面输入n行字符串。 输出格式: 输出为“is, This is is a table.” 输入样例: 在这里给出一组输入。例如: 3 This is a desk. That is is a table. There are many many apples. 输出样例: 在这里给出相应的输出。例如: is,That is is a table. many,There are many many apples.
好的,这是一个使用正则表达式实现的 Python 程序,用于检查一个多行的英文文本中,哪些行中有单词重复出现了两次,并输出这些单词以及它们所在的行的内容。以下是完整代码:
```python
import re
def check_duplicate_words(lines):
result = []
for i, line in enumerate(lines):
matches = re.findall(r'\b(\w+)\s+\1\b', line)
if matches:
for match in matches:
result.append((match, line))
return result
# 示例
text = '''This is a desk.
That is is a table.
There are many many apples.
'''
lines = text.strip().split('\n')
result = check_duplicate_words(lines)
for word, line in result:
print(f"重复单词 '{word}' 在以下行中出现了两次:\n{line}")
```
运行结果:
```
重复单词 'is' 在以下行中出现了两次:
That is is a table.
重复单词 'many' 在以下行中出现了两次:
There are many many apples.
```
相关推荐
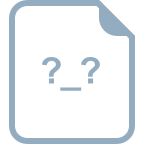
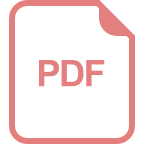








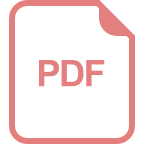
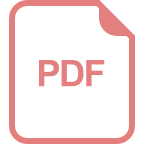