RandomAccessFile和MappedByteBuffer来实现Java SocketChannel 文件分块随机传输 代码
时间: 2024-04-10 19:35:09 浏览: 104
以下是使用RandomAccessFile和MappedByteBuffer实现Java SocketChannel文件分块随机传输的示例代码:
```java
import java.io.RandomAccessFile;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.channels.SocketChannel;
public class FileTransferExample {
private static final int CHUNK_SIZE = 1024; // 每次传输的块大小
public static void main(String[] args) {
try {
// 创建RandomAccessFile对象
RandomAccessFile file = new RandomAccessFile("path/to/file", "r");
// 获取文件通道
FileChannel fileChannel = file.getChannel();
// 创建SocketChannel对象,连接到目标主机和端口
SocketChannel socketChannel = SocketChannel.open();
socketChannel.connect(serverAddress);
// 设置文件指针的位置,即要传输的文件的起始位置
long position = 0;
while (position < file.length()) {
// 计算当前块的大小
long remaining = file.length() - position;
int chunkSize = (int) Math.min(remaining, CHUNK_SIZE);
// 创建MappedByteBuffer对象,将文件的一部分映射到内存中
MappedByteBuffer buffer = fileChannel.map(FileChannel.MapMode.READ_ONLY, position, chunkSize);
// 通过SocketChannel的write()方法将MappedByteBuffer中的数据写入到网络通道中
while (buffer.hasRemaining()) {
socketChannel.write(buffer);
}
// 更新文件指针的位置
position += chunkSize;
}
// 关闭SocketChannel和RandomAccessFile
socketChannel.close();
file.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
请注意,上述代码中的`path/to/file`需要替换为实际的文件路径,`serverAddress`需要替换为目标主机和端口。此外,还需要处理异常和错误情况,以确保文件传输的稳定性和可靠性。
阅读全文
相关推荐
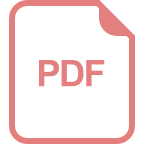





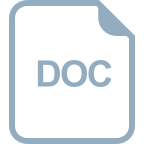
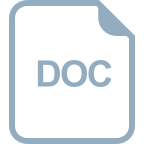








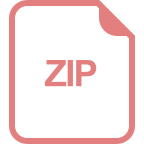