班级考勤管理系统c++链表
时间: 2024-03-13 08:40:10 浏览: 26
班级考勤管理系统是一个用于记录学生考勤情况的,使用C++表可以方便地实现对学生的存储和管理。下面是一个简单的班级考勤系统C++链表实现示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 学生节点
struct Student {
string name;
int id;
bool isPresent;
Student* next;
};
// 班级链表
class ClassList {
private:
Student* head;
public:
ClassList() {
head = nullptr;
}
// 添加学生
void addStudent(string name, int id) {
Student* newStudent = new Student;
newStudent->name = name;
newStudent->id = id;
newStudent->isPresent = false;
newStudent->next = nullptr;
if (head == nullptr) {
head = newStudent;
} else {
Student* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newStudent;
}
}
// 根据学号查找学生
Student* findStudent(int id) {
Student* current = head;
while (current != nullptr) {
if (current->id == id) {
return current;
}
current = current->next;
}
return nullptr;
}
// 标记学生出勤
void markAttendance(int id) {
Student* student = findStudent(id);
if (student != nullptr) {
student->isPresent = true;
cout << "学生 " << student->name << " 出勤已标记" << endl;
} else {
cout << "未找到学号为 " << id << " 的学生" << endl;
}
}
// 打印班级考勤情况
void printAttendance() {
Student* current = head;
while (current != nullptr) {
cout << "学生姓名:" << current->name << ",学号:" << current->id;
if (current->isPresent) {
cout << ",出勤情况:出勤" << endl;
} else {
cout << ",出勤情况:缺勤" << endl;
}
current = current->next;
}
}
};
int main() {
ClassList classList;
// 添加学生
classList.addStudent("张三", 1001);
classList.addStudent("李四", 1002);
classList.addStudent("王五", 1003);
// 标记出勤
classList.markAttendance(1001);
classList.markAttendance(1003);
// 打印考勤情况
classList.printAttendance();
return 0;
}
```
这个示例中,我们使用了一个`Student`结构体来表示学生信息,包括姓名、学号和出勤情况。然后,我们使用`ClassList`类来管理班级的学生信息,包括添加学生、查找学生、标记出勤和打印考勤情况等功能。
希望以上示例对你有所帮助!如果你有任何问题,请随时提问。
相关推荐
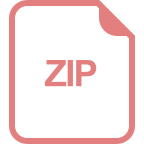
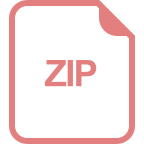














