c语言更新链表中的数据域值
时间: 2023-11-11 11:06:54 浏览: 119
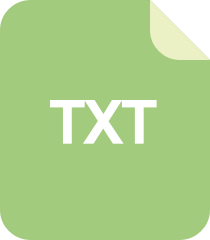
C语言中关于链表
要更新链表中的数据域值,你需要遍历链表,找到要更新的节点,然后修改其数据域的值。下面是一个使用C语言实现的示例代码,演示了如何更新链表中的数据域值。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 在链表末尾插入新节点
void insert(Node** head, int value) {
Node* newNode = createNode(value);
if (*head == NULL) {
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 更新链表中的数据域值
void updateData(Node* head, int oldValue, int newValue) {
Node* current = head;
while (current != NULL) {
if (current->data == oldValue) {
current->data = newValue;
}
current = current->next;
}
}
// 打印链表
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 释放链表内存
void freeList(Node* head) {
Node* current = head;
Node* next;
while (current != NULL) {
next = current->next;
free(current);
current = next;
}
}
int main() {
Node* head = NULL;
// 在链表末尾插入节点
insert(&head, 10);
insert(&head, 20);
insert(&head, 30);
insert(&head, 40);
insert(&head, 50);
printf("原始链表:");
printList(head);
// 更新链表中的数据域值
updateData(head, 30, 35);
printf("更新后的链表:");
printList(head);
// 释放链表内存
freeList(head);
return 0;
}
```
在这个示例中,我们定义了一个链表节点结构 `Node`,并实现了创建节点、插入节点、更新数据域值、打印链表和释放链表内存的函数。
在 `main` 函数中,我们先创建一个空链表,然后插入一些节点。接着,我们打印原始链表,然后使用 `updateData` 函数更新数据域值。最后,我们再次打印链表,然后释放链表内存。
希望这段代码能满足你的需求。如果你还有其他问题,请随时提问!
阅读全文
相关推荐
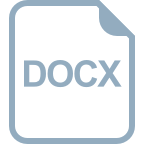
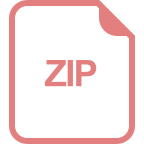
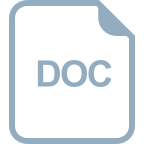

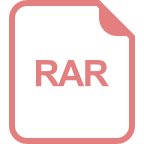
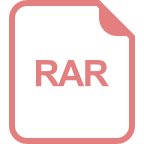
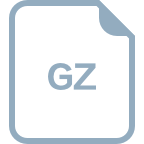
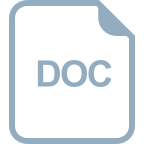
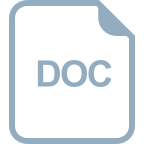
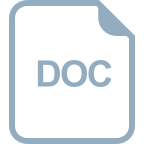







