优化这段代码import javax.swing.*; import java.awt.*; import java.awt.event.*; public class TemperatureConverter implements ActionListener { private JFrame frame; private JPanel panel; private JLabel l; private JTextField; private JButton; public TemperatureConverter() { frame = new JFrame("温度转换"); f.setSize ( 400,200 ) ; f.setVisible ( true ) ; f.setLocationRelativeTo ( null ) ; panel = new JPanel(); Label 1= new JLabel("摄氏度:"); Label 2= new JLabel("华氏度:"); Field 1= new JTextField(10); Field 2= new JTextField(10); outputField.setEditable(false); Button1= new Button("华氏度 → 摄氏度"); Button2= new Button("摄氏度 → 华氏度"); Button3= new Button("清空"); panel.add(Label1); panel.add(Label2); panel.add(Field1); panel.add(Field2); panel.add(Button1); panel.add(Button2); panel.add(Button3); public void actionPerformed(ActionEvent e) { if (e.getSource() == Button1) {
时间: 2023-12-06 18:42:48 浏览: 104
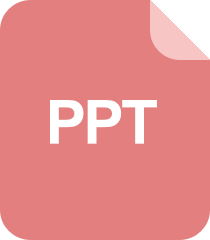
Swing包-JAVA图形界面
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class TemperatureConverter implements ActionListener {
private JFrame frame;
private JPanel panel;
private JLabel celsiusLabel;
private JLabel fahrenheitLabel;
private JTextField celsiusField;
private JTextField fahrenheitField;
private JButton convertToFahrenheitButton;
private JButton convertToCelsiusButton;
private JButton clearButton;
public TemperatureConverter() {
frame = new JFrame("温度转换");
frame.setSize(400, 200);
frame.setVisible(true);
frame.setLocationRelativeTo(null);
panel = new JPanel();
celsiusLabel = new JLabel("摄氏度:");
fahrenheitLabel = new JLabel("华氏度:");
celsiusField = new JTextField(10);
fahrenheitField = new JTextField(10);
fahrenheitField.setEditable(false);
convertToFahrenheitButton = new JButton("华氏度 → 摄氏度");
convertToCelsiusButton = new JButton("摄氏度 → 华氏度");
clearButton = new JButton("清空");
convertToFahrenheitButton.addActionListener(this);
convertToCelsiusButton.addActionListener(this);
clearButton.addActionListener(this);
panel.add(celsiusLabel);
panel.add(celsiusField);
panel.add(fahrenheitLabel);
panel.add(fahrenheitField);
panel.add(convertToFahrenheitButton);
panel.add(convertToCelsiusButton);
panel.add(clearButton);
frame.add(panel);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == convertToFahrenheitButton) {
try {
double celsius = Double.parseDouble(celsiusField.getText());
double fahrenheit = celsius * 1.8 + 32;
fahrenheitField.setText(String.format("%.2f", fahrenheit));
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(frame, "请输入有效的摄氏度");
}
} else if (e.getSource() == convertToCelsiusButton) {
try {
double fahrenheit = Double.parseDouble(fahrenheitField.getText());
double celsius = (fahrenheit - 32) / 1.8;
celsiusField.setText(String.format("%.2f", celsius));
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(frame, "请输入有效的华氏度");
}
} else if (e.getSource() == clearButton) {
celsiusField.setText("");
fahrenheitField.setText("");
}
}
public static void main(String[] args) {
new TemperatureConverter();
}
}
改进思路:
1. 更改变量和组件命名,使其更有意义和易读。
2. 添加异常处理,确保用户输入有效的数字。
3. 重新排版代码,使其更易读和维护。
阅读全文
相关推荐
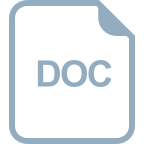
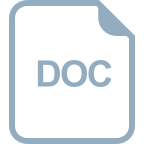















