Springboot 每周一8点发邮件代码
时间: 2023-08-31 12:31:49 浏览: 131
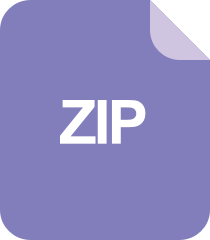
SpringBoot发送邮件代码
### 回答1:
以下是一个示例的 Spring Boot 代码,可用于每周一 8 点发送邮件:
```
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class EmailScheduler {
@Scheduled(cron = "0 0 8 ? * MON")
public void sendEmail() {
// 这里写发送邮件的代码
System.out.println("已发送邮件");
}
}
```
这个代码片段使用 Spring Boot 的定时任务功能 `@Scheduled` 和 Cron 表达式来实现每周一 8 点执行一次发送邮件的任务。在 `sendEmail()` 方法中,可以编写实际的发送邮件逻辑。这个示例代码中,我只是简单地输出了一行文本来模拟发送邮件的过程。
### 回答2:
下面是一个使用Spring Boot框架每周一8点发送邮件的代码示例:
1. 创建一个Spring Boot项目,并添加所需的依赖。
2. 创建一个发送邮件的Service类,例如EmailService。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Service;
@Service
public class EmailService {
private JavaMailSender javaMailSender;
@Autowired
public EmailService(JavaMailSender javaMailSender) {
this.javaMailSender = javaMailSender;
}
public void sendEmail(String to, String subject, String text) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(to);
message.setSubject(subject);
message.setText(text);
javaMailSender.send(message);
}
}
```
3. 创建一个定时任务类,例如EmailScheduler。
```java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class EmailScheduler {
private EmailService emailService;
public EmailScheduler(EmailService emailService) {
this.emailService = emailService;
}
@Scheduled(cron = "0 0 8 ? * MON") // 每周一8点触发
public void sendEmail() {
String to = "your_email@example.com";
String subject = "每周一8点的邮件";
String text = "这是一封测试邮件。";
emailService.sendEmail(to, subject, text);
}
}
```
4. 配置邮件服务器信息,在application.properties或application.yml文件中添加以下配置:
```yaml
spring.mail.host=your_email_host
spring.mail.port=your_email_port
spring.mail.username=your_email_username
spring.mail.password=your_email_password
```
将"your_email_host"、"your_email_port"、"your_email_username"和"your_email_password"替换为你的邮件服务器信息。
5. 运行Spring Boot应用程序,每周一8点时将会触发sendEmail方法发送一封邮件到指定的邮箱地址。
注意:在实际使用中,需要根据自己的需求进行一些修改,例如邮箱地址、邮件内容等。
### 回答3:
要实现每周一8点发邮件的功能,可以使用Spring Boot框架和JavaMailSender来发送邮件。
首先,我们需要在pom.xml文件中添加Spring Boot Starter Mail依赖项。这可以通过在<dependencies>标签中添加以下代码来完成:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
```
接下来,我们需要配置邮件相关的属性。在application.properties文件中添加以下代码:
```
spring.mail.host=your_smtp_host
spring.mail.port=your_smtp_port
spring.mail.username=your_email_username
spring.mail.password=your_email_password
```
然后,我们可以编写发送邮件的代码。以下是一个简单的示例:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.mail.MailProperties;
import org.springframework.boot.autoconfigure.mail.MailSenderAutoConfiguration;
import org.springframework.context.annotation.Bean;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.JavaMailSenderImpl;
import org.springframework.scheduling.annotation.EnableScheduling;
import org.springframework.scheduling.annotation.Scheduled;
@SpringBootApplication(exclude = MailSenderAutoConfiguration.class)
@EnableScheduling
public class EmailSenderApplication {
@Autowired
private MailProperties mailProperties;
@Autowired
private JavaMailSender javaMailSender;
public static void main(String[] args) {
SpringApplication.run(EmailSenderApplication.class, args);
}
@Scheduled(cron = "0 0 8 * * MON") // 每周一的8:00执行
public void sendEmail() {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo("recipient@example.com");
message.setSubject("邮件主题");
message.setText("邮件内容");
javaMailSender.send(message);
}
@Bean
public JavaMailSender javaMailSender() {
JavaMailSenderImpl mailSender = new JavaMailSenderImpl();
mailSender.setHost(mailProperties.getHost());
mailSender.setPort(mailProperties.getPort());
mailSender.setUsername(mailProperties.getUsername());
mailSender.setPassword(mailProperties.getPassword());
return mailSender;
}
}
```
在上述代码中,我们使用了@EnableScheduling注解来启用定时任务,并通过@Scheduled注解指定了发送邮件的时间。在sendEmail()方法中,我们创建了一个SimpleMailMessage对象来设置邮件的收件人地址、主题和内容,然后通过javaMailSender.send()方法发送邮件。
请注意,以上代码中的"your_smtp_host"、"your_smtp_port"、"your_email_username"和"your_email_password"需要替换为实际的SMTP服务器地址、端口号、邮箱用户名和密码。
通过以上步骤,我们就可以实现每周一8点发邮件的功能了。
阅读全文
相关推荐
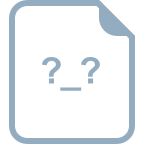
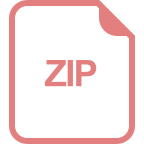
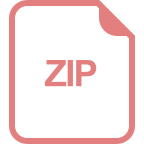
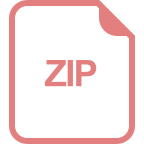
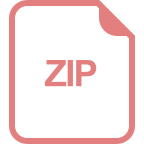
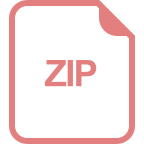
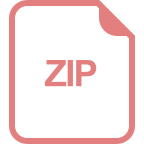
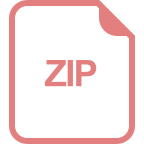
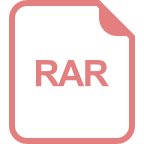
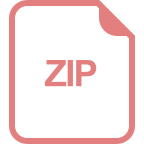
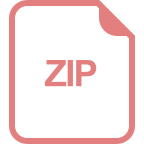
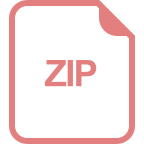
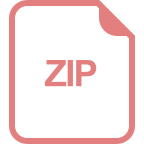
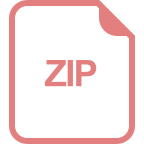