qt c++,判断文件夹容量,超出设定的限值,则文件夹改名,然后新建一个同名的文件夹
时间: 2024-12-19 21:18:41 浏览: 10
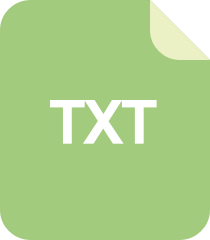
QT文件夹绝对路径定位

在Qt C++中,如果你需要检查一个文件夹的容量并根据预设的大小限制对文件夹进行操作,你可以使用QDir类以及QFile类来进行文件和目录的操作。以下是一个简单的步骤概述:
1. 引入所需的库头文件:
```cpp
#include <QDir>
#include <QFileInfo>
```
2. 定义一个函数来计算文件夹容量:
```cpp
qint64 calculateFolderSize(const QString &folderPath) {
QDir dir(folderPath);
qint64 size = 0;
foreach (const QFileInfo &fileInfo, dir.entryInfoList(QDir::Files | QDir::Dirs)) {
if (!fileInfo.isSymLink()) { // 避免计算链接
size += fileInfo.size();
}
}
return size;
}
```
3. 检查文件夹容量并与设定的阈值比较:
```cpp
bool exceedsLimit(qint64 currentSize, qint64 limit) {
return currentSize > limit;
}
// 示例中假设limit变量存储了文件夹容量的上限
bool shouldRename(QString folderPath, qint64 limit) {
qint64 size = calculateFolderSize(folderPath);
return exceedsLimit(size, limit);
}
```
4. 实现文件夹的改名和新建:
```cpp
void handleExceedance(QString sourceFolderPath, QString targetFolderPath) {
if (shouldRename(sourceFolderPath, /*limit*/)) {
// 改名
QDir().removeRecursively(targetFolderPath); // 先删除旧的同名文件夹,如果存在
QDir().rename(sourceFolderPath, targetFolderPath);
// 新建同名文件夹
QDir().mkpath(targetFolderPath);
} else {
qDebug() << "文件夹容量未超过限制,无需操作";
}
}
```
记得在实际应用中替换`/*limit*/`为实际的容量限制。
阅读全文
相关推荐
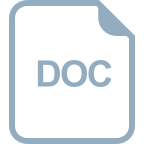
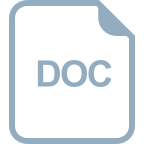















