c语言实现(1)实现单链表的创建;(2)实现单链表的插入;(3)实现单链表的删除(4)实现单链表的查找;(5)实现单链表的显示;
时间: 2023-05-25 13:04:31 浏览: 84
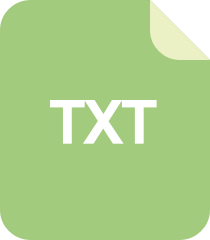
链表的C语言实现之单链表的实现.txt
1. 单链表的创建:
// 定义单链表节点结构体
struct ListNode {
int val; // 存储节点数据
struct ListNode *next; // 指向下一个节点
};
// 创建单链表
struct ListNode* createList(int arr[], int n) {
if (n == 0) {
return NULL;
}
struct ListNode *head = (struct ListNode*)malloc(sizeof(struct ListNode)); // 创建头节点
head->val = arr[0];
head->next = NULL;
struct ListNode *p = head; // p指向最后一个节点
for (int i = 1; i < n; i++) {
struct ListNode *node = (struct ListNode*)malloc(sizeof(struct ListNode)); // 创建新节点
node->val = arr[i];
node->next = NULL;
p->next = node; // 将新节点连接到链表末尾
p = node; // 更新p指针
}
return head;
}
2. 单链表的插入:
// 在单链表中插入节点
struct ListNode* insert(struct ListNode *head, int val, int pos) {
struct ListNode *node = (struct ListNode*)malloc(sizeof(struct ListNode)); // 创建新节点
node->val = val;
if (pos == 1) { // 在头节点前插入
node->next = head;
head = node; // 更新头节点
return head;
}
int k = 1;
struct ListNode *p = head;
while (p != NULL && k < pos - 1) { // 找到插入位置的前一个节点
p = p->next;
k++;
}
if (p == NULL) { // 插入位置大于链表长度
printf("Error: position exceeds the length of the list!\n");
free(node);
return head;
}
node->next = p->next; // 将新节点插入到链表中
p->next = node;
return head;
}
3. 单链表的删除:
// 在单链表中删除节点
struct ListNode* delete(struct ListNode *head, int pos) {
if (head == NULL) { // 链表为空
printf("Error: the list is empty!\n");
return head;
}
if (pos == 1) { // 删除头节点
struct ListNode *p = head;
head = head->next;
free(p);
return head;
}
int k = 1;
struct ListNode *p = head;
while (p != NULL && k < pos - 1) { // 找到待删除节点的前一个节点
p = p->next;
k++;
}
if (p == NULL || p->next == NULL) { // 删除位置大于链表长度
printf("Error: position exceeds the length of the list!\n");
return head;
}
struct ListNode *q = p->next; // 删除节点
p->next = q->next;
free(q);
return head;
}
4. 单链表的查找:
// 在单链表中查找元素
int search(struct ListNode *head, int val) {
if (head == NULL) { // 链表为空
return -1;
}
int pos = 1;
struct ListNode *p = head;
while (p != NULL && p->val != val) { // 顺序遍历链表
p = p->next;
pos++;
}
if (p == NULL) { // 链表中不存在该元素
return -1;
}
return pos;
}
5. 单链表的显示:
// 显示单链表
void display(struct ListNode *head) {
if (head == NULL) { // 链表为空
printf("The list is empty!\n");
return;
}
struct ListNode *p = head;
while (p != NULL) { // 顺序遍历链表
printf("%d ", p->val);
p = p->next;
}
printf("\n");
}
阅读全文
相关推荐
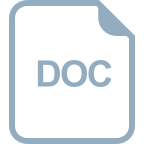
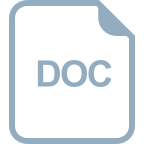
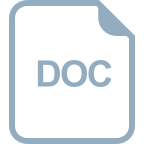
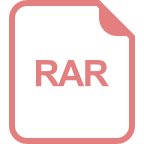
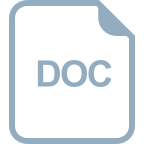
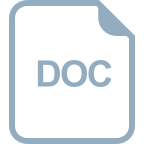
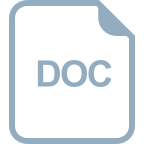
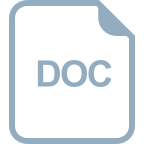
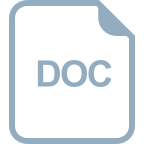
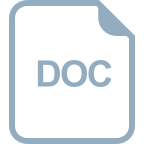
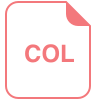






