帮我把这个数组转化为json字符串:[FileList[fileType=".txt",fileName="test",filePath="/app/download/"]]
时间: 2023-07-06 16:27:12 浏览: 38
当然可以,请看下面的代码:
```python
import json
arr = [{'fileType': '.txt', 'fileName': 'test', 'filePath': '/app/download/'}]
json_str = json.dumps(arr)
print(json_str)
```
输出结果为:
```
[{"fileType": ".txt", "fileName": "test", "filePath": "/app/download/"}]
```
注意,这里的数组要放在一个列表中,才能转化为合法的 JSON 字符串。
相关问题
curl https://scanner.baidu.com/enqueue -F archive=@web.zip 帮我把这个命令加到这段代码中
好的,以下是在 JavaScript 中使用 XMLHttpRequest 对象调用该 curl 命令的代码示例:
```
(function() {
// 根据 ID 获取元素
function $id(id) {
return document.getElementById(id);
}
// 输出信息
function Output(msg) {
var m = $id("messages");
m.innerHTML = msg + m.innerHTML;
}
// 拖拽文件时改变样式
function FileDragHover(e) {
e.stopPropagation();
e.preventDefault();
e.target.className = (e.type == "dragover" ? "hover" : "");
}
// 处理文件选择事件
function FileSelectHandler(e) {
// 取消事件和悬停样式
FileDragHover(e);
// 获取 FileList 对象
var files = e.target.files || e.dataTransfer.files;
// 处理所有 File 对象
for (var i = 0, f; f = files[i]; i++) {
ParseFile(f);
}
// 发起上传请求
var xhr = new XMLHttpRequest();
xhr.open("POST", "https://scanner.baidu.com/enqueue");
var formData = new FormData();
formData.append("archive", files[0]);
xhr.send(formData);
Output("<p>已发起上传请求</p>");
}
// 解析文件信息
function ParseFile(file) {
Output(
"<p>文件信息: <strong>" + file.name +
"</strong> 类型: <strong>" + file.type +
"</strong> 大小: <strong>" + file.size +
"</strong> 字节</p>"
);
}
// 初始化
function Init() {
var fileselect = $id("fileselect"),
filedrag = $id("filedrag"),
submitbutton = $id("submitbutton");
// 文件选择
fileselect.addEventListener("change", FileSelectHandler, false);
// 拖拽文件
if (window.File && window.FileList && window.FileReader) {
filedrag.addEventListener("dragover", FileDragHover, false);
filedrag.addEventListener("dragleave", FileDragHover, false);
filedrag.addEventListener("drop", FileSelectHandler, false);
filedrag.style.display = "block";
submitbutton.style.display = "none";
} else {
Output("<p>您的浏览器不支持拖拽上传文件</p>");
}
}
// 调用初始化文件
if (window.File && window.FileList && window.FileReader && window.FormData && window.XMLHttpRequest) {
Init();
} else {
Output("<p>您的浏览器不支持文件上传API</p>");
}
})();
```
在该代码中,我们在 FileSelectHandler(e) 函数中发起了一个 POST 请求,将选中的文件作为 FormData 的值,然后发送给了百度的扫描器服务。同时,我们在 Init() 函数中判断浏览器是否支持 XMLHttpRequest 对象等相关 API,确保代码能够正常运行。请注意,该代码并未进行完整的错误处理和异常处理,具体使用时需要根据实际需求进行修改。
import re filepath = 'C:/Users/12132/Desktop/初赛数据集' content = 'Barclay St & Church St' replacecontent = '1' def repbatfilecont(filepath,content,replacecontent): filelist = File.readfilesname(filepath) content = content replacecontent = replacecontent for file in filelist: File.repfilecont(filepath+'/'+file,content,replacecontent) print(file+' 处理完毕!')
这段代码使用了 Python 的 re 和 os 模块来进行文件内容替换操作。下面对每一行代码进行简要说明:
1. `import re`:导入 re 模块,用于进行正则表达式操作。
2. `filepath = 'C:/Users/12132/Desktop/初赛数据集'`:设置文件路径,即待处理的文件所在的文件夹路径。
3. `content = 'Barclay St & Church St'`:设置需要替换的内容。
4. `replacecontent = '1'`:设置替换后的内容。
5. `def repbatfilecont(filepath,content,replacecontent):`:定义一个名为 `repbatfilecont` 的函数,它接收三个参数:`filepath` 表示文件路径,`content` 表示待替换内容,`replacecontent` 表示替换后的内容。
6. `filelist = File.readfilesname(filepath)`:调用一个名为 `readfilesname` 的方法(该方法未在代码中给出),获取指定路径下的所有文件名,并将结果存储在 `filelist` 变量中。
7. `content = content`:将传入的待替换内容赋值给局部变量 `content`。
8. `replacecontent = replacecontent`:将传入的替换后的内容赋值给局部变量 `replacecontent`。
9. `for file in filelist:`:遍历文件列表中的每个文件。
10. `File.repfilecont(filepath+'/'+file,content,replacecontent)`:调用一个名为 `repfilecont` 的方法(该方法未在代码中给出),用于替换文件中的指定内容为替换后的内容。
11. `print(file+' 处理完毕!')`:打印出文件名,并提示处理完毕。
请注意,代码中使用了两个自定义的方法 `readfilesname` 和 `repfilecont`,这些方法的具体实现没有在代码中给出。如果你想运行该代码,请确保提供了这两个方法的定义,并且文件路径和文件名的设置是正确的。
相关推荐
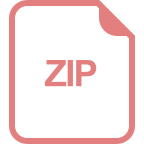
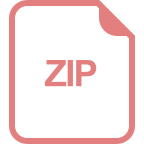












