编写一段程序,读入一个包含标点符号的字符串,将标点符号去除后输出字符串剩余的部分。
时间: 2023-05-12 12:03:59 浏览: 62
以下是一段 Python 代码,可以实现这个功能:
```python
import string
def remove_punctuation(text):
# 创建一个包含所有标点符号的字符串
punctuations = string.punctuation
# 创建一个空字符串,用于存储去除标点符号后的文本
no_punct = ""
# 遍历文本中的每个字符
for char in text:
# 如果该字符不是标点符号,则将其添加到 no_punct 字符串中
if char not in punctuations:
no_punct += char
# 返回去除标点符号后的文本
return no_punct
# 读入包含标点符号的字符串
text = input("请输入包含标点符号的字符串:")
# 调用 remove_punctuation 函数,去除标点符号
no_punct_text = remove_punctuation(text)
# 输出去除标点符号后的文本
print("去除标点符号后的文本为:", no_punct_text)
```
如果输入的字符串为:"Hello, world! How are you?"
则输出为:"去除标点符号后的文本为: Hello world How are you"
相关问题
基于C++,编写一段程序,读入一个包含标点符号的字符串,将标点符号去除后输出字符串剩余的部分。
以下是基于C的程序,可以实现读入一个包含标点符号的字符串,将标点符号去除后输出字符串剩余的部分:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[1000];
int i, j;
printf("请输入一个包含标点符号的字符串:\n");
fgets(str, 1000, stdin);
for (i = 0, j = 0; str[i] != '\0'; i++) {
if (!ispunct(str[i])) {
str[j++] = str[i];
}
}
str[j] = '\0';
printf("去除标点符号后的字符串为:\n%s", str);
return 0;
}
```
这个程序使用了 `ispunct()` 函数来判断一个字符是否为标点符号,如果不是标点符号,则将其保存到新的字符串中。最后输出新的字符串即可。
注意,这个程序只能去除 ASCII 码中的标点符号,对于一些中文标点符号可能无法处理。
基于Cpp,编写一段程序,读入一个包含标点符号的字符串,将标点符号去除后输出字符串剩余的部分。
当然可以,以下是代码示例:
```cpp
#include <iostream>
#include <string>
#include <cctype>
using namespace std;
int main() {
string str;
getline(cin, str); // 读入包含标点符号的字符串
string result = "";
for (int i = 0; i < str.length(); i++) {
if (!ispunct(str[i])) { // 判断是否为标点符号
result += str[i]; // 如果不是,则加入结果字符串
}
}
cout << result << endl; // 输出去除标点符号后的字符串
return 0;
}
```
希望这个程序能够帮到你!
相关推荐
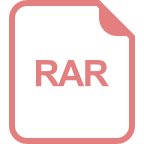












