stm32f10x系列,设定极坐标以确定风摆位置实例
时间: 2024-06-12 16:08:17 浏览: 26
下面是一个基于STM32F10x系列的风摆位置测量的实例:
1.硬件配置
在STM32F10x系列中,使用定时器模块可以实现PWM输出和捕获功能。因此,我们需要将风摆的位置传感器连接到定时器捕获通道上。
2.软件实现
在软件中,我们需要实现以下步骤:
1)初始化定时器模块和GPIO引脚。
2)启动定时器,开始PWM输出。
3)通过定时器捕获模块,实时获取风摆位置传感器的角度值。
4)将角度值转换为极坐标,并根据极坐标确定风摆位置。
下面是一个简单的代码示例:
```c
#include "stm32f10x.h"
#include <math.h>
#define PI 3.14159265358979323846
uint16_t capture1 = 0;
uint16_t capture2 = 0;
uint16_t period = 0;
uint8_t direction = 0;
void TIM2_IRQHandler(void)
{
if(TIM_GetITStatus(TIM2, TIM_IT_CC1) == SET)
{
TIM_ClearITPendingBit(TIM2, TIM_IT_CC1);
capture1 = TIM_GetCapture1(TIM2);
if(direction == 0)
{
direction = 1;
period = 0;
TIM_SetCounter(TIM2, 0);
}
else
{
direction = 0;
period = capture1 - capture2;
capture2 = capture1;
}
}
}
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_ICInitTypeDef TIM_ICInitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
TIM_TimeBaseStructure.TIM_Period = 65535;
TIM_TimeBaseStructure.TIM_Prescaler = 71;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
TIM_ICInitStructure.TIM_Channel = TIM_Channel_1;
TIM_ICInitStructure.TIM_ICPolarity = TIM_ICPolarity_Rising;
TIM_ICInitStructure.TIM_ICSelection = TIM_ICSelection_DirectTI;
TIM_ICInitStructure.TIM_ICPrescaler = TIM_ICPSC_DIV1;
TIM_ICInitStructure.TIM_ICFilter = 0x0F;
TIM_ICInit(TIM2, &TIM_ICInitStructure);
TIM_ITConfig(TIM2, TIM_IT_CC1, ENABLE);
NVIC_InitStructure.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
TIM_Cmd(TIM2, ENABLE);
while(1)
{
float angle = period * 360 / 65535.0;
float x = sin(angle * PI / 180);
float y = cos(angle * PI / 180);
// 根据极坐标确定风摆位置
// ...
}
}
```
在上述代码中,我们使用TIM2定时器模块捕获GPIOA0引脚上的信号,并根据捕获到的值计算出风摆的角度值。然后,我们将角度值转换为极坐标,并根据极坐标确定风摆位置。在实际应用中,可以根据风摆位置进行相关的控制操作。
需要注意的是,在实现过程中,需要根据具体的硬件情况进行相应的参数调整和校准,以确保测量的精度和准确性。
阅读全文
相关推荐
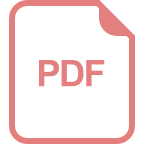
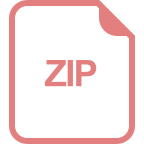
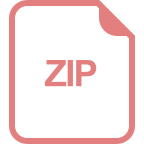
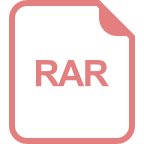
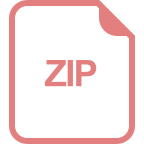
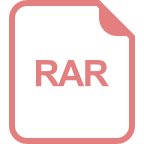
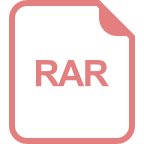
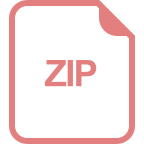
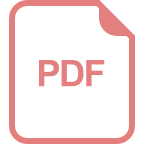
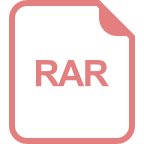
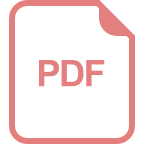
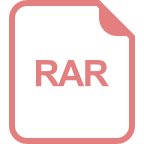
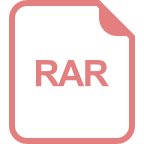
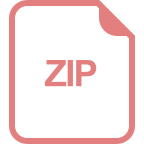
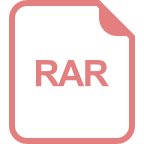
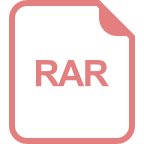
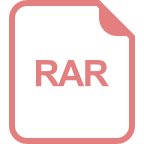
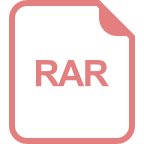
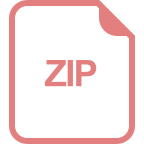