你作为一个Java领域的专家,请完成以下任务:一个大文件中,每一行有一个整数,怎么找第100大的数?
时间: 2023-07-14 18:12:39 浏览: 61
我们可以使用堆排序来解决这个问题。首先,我们可以创建一个大小为100的小根堆,然后将文件中的前100个整数插入堆中。接下来,我们遍历剩余的整数,如果当前整数比堆顶元素大,就将堆顶元素替换为当前整数,并重新调整堆,使其保持小根堆的性质。最后,堆顶元素即为第100大的数。
以下是Java代码实现:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.PriorityQueue;
import java.util.Scanner;
public class Find100thLargestNumber {
public static void main(String[] args) {
File file = new File("file.txt");
int k = 100;
int count = 0;
PriorityQueue<Integer> heap = new PriorityQueue<>(k);
try (Scanner scanner = new Scanner(file)) {
while (scanner.hasNextInt()) {
int num = scanner.nextInt();
if (count < k) {
heap.offer(num);
} else {
if (num > heap.peek()) {
heap.poll();
heap.offer(num);
}
}
count++;
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
System.out.println("The 100th largest number is: " + heap.peek());
}
}
```
这段代码首先创建一个大小为100的小根堆,然后遍历文件中的整数,如果当前整数比堆顶元素大,则将堆顶元素替换为当前整数,并重新调整堆。最后,堆顶元素即为第100大的数。注意,这里假设文件中至少有100个整数。如果文件中的整数不足100个,则需要进行特殊处理。
相关推荐
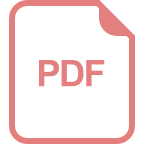














