重新用java把全部代码写出来
时间: 2024-03-13 21:43:42 浏览: 17
好的,以下是完整的 Java 代码:
```
import java.math.BigInteger;
import java.nio.charset.StandardCharsets;
import java.util.Random;
public class RSA {
private BigInteger p;
private BigInteger q;
private BigInteger n;
private BigInteger phi;
private BigInteger e;
private BigInteger d;
public RSA(BigInteger p, BigInteger q) {
this.p = p;
this.q = q;
this.n = p.multiply(q);
this.phi = p.subtract(BigInteger.ONE).multiply(q.subtract(BigInteger.ONE));
this.e = generateE(phi);
this.d = e.modInverse(phi);
}
public BigInteger encrypt(String message) {
byte[] bytes = message.getBytes(StandardCharsets.UTF_8);
BigInteger m = new BigInteger(bytes);
return m.modPow(e, n);
}
public String decrypt(BigInteger cipher) {
BigInteger m = cipher.modPow(d, n);
byte[] bytes = m.toByteArray();
return new String(bytes, StandardCharsets.UTF_8);
}
private BigInteger generateE(BigInteger phi) {
BigInteger e = BigInteger.probablePrime(phi.bitLength(), new Random());
while (!phi.gcd(e).equals(BigInteger.ONE)) {
e = BigInteger.probablePrime(phi.bitLength(), new Random());
}
return e;
}
public static void main(String[] args) {
BigInteger p = BigInteger.valueOf(17);
BigInteger q = BigInteger.valueOf(19);
RSA rsa = new RSA(p, q);
String message = "Hello, world!";
System.out.println("Original message: " + message);
BigInteger cipher = rsa.encrypt(message);
System.out.println("Encrypted message: " + cipher);
String decryptedMessage = rsa.decrypt(cipher);
System.out.println("Decrypted message: " + decryptedMessage);
}
}
```
这个程序可以生成一个 RSA 密钥对,加密和解密字符串消息。在 `main` 方法中,我们使用了一个简单的示例消息 "Hello, world!" 进行加密和解密,您可以根据需要修改这个消息。
相关推荐
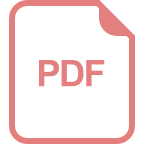















