Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/attendance", "root", "password");具体用法
时间: 2023-11-30 22:03:07 浏览: 90
这行代码是用来获取一个 MySQL 数据库连接的,具体用法解释如下:
1. DriverManager 是 Java 中用于管理 JDBC 驱动程序的类,它提供了一组静态方法来获取数据库连接。
2. getConnection 方法是 DriverManager 类提供的获取数据库连接的静态方法,它需要三个参数:
- 第一个参数是数据库的 URL,它包含了数据库的地址、端口号和数据库名称等信息。在这个例子中,数据库 URL 是 "jdbc:mysql://localhost:3306/attendance",表示连接到本地主机上端口号为 3306 的 MySQL 数据库中的名为 attendance 的数据库。
- 第二个参数是数据库的用户名,它表示连接数据库时使用的用户名。在这个例子中,用户名是 "root"。
- 第三个参数是数据库的密码,它表示连接数据库时使用的密码。在这个例子中,密码是 "password"。
3. 这行代码将返回一个 Connection 对象,它表示与 MySQL 数据库的连接。可以使用这个 Connection 对象来执行 SQL 语句并处理查询结果。
需要注意的是,这行代码只是获取了一个数据库连接,还需要根据具体的业务需求使用 Connection 对象来执行相应的 SQL 语句。同时,密码应该保密,不要在代码中明文显示密码。
相关问题
您需要编写Java程序来连接MySQL数据库,并实现各种操作,如添加、删除、修改和查询学生信息、课程信息和考勤信息等等。
对的,连接MySQL数据库和实现各种操作可以使用Java语言中的JDBC(Java DataBase Connectivity)API。下面是一个简单的示例代码,以连接MySQL数据库并查询学生信息为例:
```java
import java.sql.*;
public class StudentInfo {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 加载JDBC驱动
Class.forName("com.mysql.jdbc.Driver");
// 连接MySQL数据库
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/student_attendance", "username", "password");
// 创建Statement对象
stmt = conn.createStatement();
// 查询学生信息
rs = stmt.executeQuery("SELECT * FROM student");
// 打印查询结果
while (rs.next()) {
System.out.println("学号:" + rs.getString("student_id") + ",姓名:" + rs.getString("name") + ",性别:" + rs.getString("gender"));
}
} catch (SQLException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} finally {
// 关闭连接、Statement和ResultSet对象
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
此代码可以连接到名为student_attendance的数据库,并查询其中的学生信息表student。您可以根据需要修改代码以实现添加、删除、修改和查询课程信息和考勤信息等操作。
用myeclipse创建一个简单班级考勤管理系统网页,给出具体部署过程和代码
好的,我可以提供一些代码和部署过程的指导。首先,我们需要安装MyEclipse和Tomcat服务器。然后,我们可以按照以下步骤进行操作:
1. 创建一个Web项目
在MyEclipse中选择“File” -> “New” -> “Web Project”,填写项目名称和目标运行服务器(Tomcat),并选择Web应用程序版本(3.1或更高版本)。
2. 添加依赖库
在项目上单击鼠标右键,选择“Properties” -> “Java Build Path” -> “Libraries” -> “Add Library”,添加所需的JAR包,例如JDBC驱动程序。
3. 创建数据库
我们可以使用MySQL数据库,创建一个名为“attendance”的数据库和三个表,分别为“student”、“teacher”和“attendance_record”。表结构如下:
```
CREATE TABLE student (
id int(11) NOT NULL AUTO_INCREMENT,
name varchar(50) NOT NULL,
gender varchar(10) NOT NULL,
PRIMARY KEY (id)
);
CREATE TABLE teacher (
id int(11) NOT NULL AUTO_INCREMENT,
name varchar(50) NOT NULL,
PRIMARY KEY (id)
);
CREATE TABLE attendance_record (
id int(11) NOT NULL AUTO_INCREMENT,
student_id int(11) NOT NULL,
teacher_id int(11) NOT NULL,
record_date date NOT NULL,
is_present tinyint(1) NOT NULL,
PRIMARY KEY (id),
FOREIGN KEY (student_id) REFERENCES student(id),
FOREIGN KEY (teacher_id) REFERENCES teacher(id)
);
```
4. 编写JSP页面
我们可以创建以下JSP页面:
- index.jsp:显示“学生考勤”和“教师考勤”两个链接。
- student.jsp:显示学生列表和考勤记录。
- teacher.jsp:显示教师列表和考勤记录。
index.jsp代码如下:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>班级考勤管理系统</title>
</head>
<body>
<h1>班级考勤管理系统</h1>
<ul>
<li><a href="student.jsp">学生考勤</a></li>
<li><a href="teacher.jsp">教师考勤</a></li>
</ul>
</body>
</html>
```
student.jsp代码如下:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生考勤</title>
</head>
<body>
<h1>学生考勤</h1>
<table>
<tr>
<th>ID</th>
<th>姓名</th>
<th>性别</th>
<th>考勤记录</th>
</tr>
<%
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/attendance", "root", "password");
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM student");
while(rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String gender = rs.getString("gender");
out.println("<tr>");
out.println("<td>" + id + "</td>");
out.println("<td>" + name + "</td>");
out.println("<td>" + gender + "</td>");
out.println("<td><a href=\"attend.jsp?student_id=" + id + "\">查看考勤记录</a></td>");
out.println("</tr>");
}
rs.close();
stmt.close();
conn.close();
%>
</table>
</body>
</html>
```
teacher.jsp代码如下:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>教师考勤</title>
</head>
<body>
<h1>教师考勤</h1>
<table>
<tr>
<th>ID</th>
<th>姓名</th>
<th>考勤记录</th>
</tr>
<%
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/attendance", "root", "password");
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM teacher");
while(rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
out.println("<tr>");
out.println("<td>" + id + "</td>");
out.println("<td>" + name + "</td>");
out.println("<td><a href=\"attend.jsp?teacher_id=" + id + "\">查看考勤记录</a></td>");
out.println("</tr>");
}
rs.close();
stmt.close();
conn.close();
%>
</table>
</body>
</html>
```
attend.jsp代码如下:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>考勤记录</title>
</head>
<body>
<h1>考勤记录</h1>
<table>
<tr>
<th>日期</th>
<th>是否出席</th>
</tr>
<%
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/attendance", "root", "password");
PreparedStatement pstmt = null;
ResultSet rs = null;
if(request.getParameter("student_id") != null) {
int student_id = Integer.parseInt(request.getParameter("student_id"));
pstmt = conn.prepareStatement("SELECT * FROM attendance_record WHERE student_id=?");
pstmt.setInt(1, student_id);
rs = pstmt.executeQuery();
} else if(request.getParameter("teacher_id") != null) {
int teacher_id = Integer.parseInt(request.getParameter("teacher_id"));
pstmt = conn.prepareStatement("SELECT * FROM attendance_record WHERE teacher_id=?");
pstmt.setInt(1, teacher_id);
rs = pstmt.executeQuery();
}
while(rs.next()) {
Date record_date = rs.getDate("record_date");
boolean is_present = rs.getBoolean("is_present");
out.println("<tr>");
out.println("<td>" + record_date + "</td>");
out.println("<td>" + (is_present ? "出席" : "缺席") + "</td>");
out.println("</tr>");
}
rs.close();
pstmt.close();
conn.close();
%>
</table>
</body>
</html>
```
其中,上述JSP页面中的数据库连接信息需要根据实际情况修改。
5. 编写Java代码
我们可以创建以下Java类:
- Student:表示学生,包括id、name和gender等属性,以及获取和设置这些属性的方法。
- Teacher:表示教师,包括id和name等属性,以及获取和设置这些属性的方法。
- AttendanceRecord:表示考勤记录,包括id、student、teacher、recordDate和isPresent等属性,以及获取和设置这些属性的方法。
- AttendanceDao:提供访问数据库的方法,包括获取学生列表、教师列表、考勤记录等操作。
Student代码如下:
```
public class Student {
private int id;
private String name;
private String gender;
public Student(int id, String name, String gender) {
this.id = id;
this.name = name;
this.gender = gender;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
}
```
Teacher代码如下:
```
public class Teacher {
private int id;
private String name;
public Teacher(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
```
AttendanceRecord代码如下:
```
public class AttendanceRecord {
private int id;
private Student student;
private Teacher teacher;
private Date recordDate;
private boolean isPresent;
public AttendanceRecord(int id, Student student, Teacher teacher, Date recordDate, boolean isPresent) {
this.id = id;
this.student = student;
this.teacher = teacher;
this.recordDate = recordDate;
this.isPresent = isPresent;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public Student getStudent() {
return student;
}
public void setStudent(Student student) {
this.student = student;
}
public Teacher getTeacher() {
return teacher;
}
public void setTeacher(Teacher teacher) {
this.teacher = teacher;
}
public Date getRecordDate() {
return recordDate;
}
public void setRecordDate(Date recordDate) {
this.recordDate = recordDate;
}
public boolean isPresent() {
return isPresent;
}
public void setPresent(boolean isPresent) {
this.isPresent = isPresent;
}
}
```
AttendanceDao代码如下:
```
public class AttendanceDao {
private static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
private static final String DB_URL = "jdbc:mysql://localhost:3306/attendance";
private static final String USER = "root";
private static final String PASS = "password";
public List<Student> getStudentList() {
List<Student> studentList = new ArrayList<Student>();
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT * FROM student");
while(rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String gender = rs.getString("gender");
Student student = new Student(id, name, gender);
studentList.add(student);
}
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if(rs != null) rs.close();
if(stmt != null) stmt.close();
if(conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
return studentList;
}
public List<Teacher> getTeacherList() {
List<Teacher> teacherList = new ArrayList<Teacher>();
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT * FROM teacher");
while(rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
Teacher teacher = new Teacher(id, name);
teacherList.add(teacher);
}
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if(rs != null) rs.close();
if(stmt != null) stmt.close();
if(conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
return teacherList;
}
public List<AttendanceRecord> getAttendanceRecordList(int studentId, int teacherId) {
List<AttendanceRecord> recordList = new ArrayList<AttendanceRecord>();
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
if(studentId > 0) {
pstmt = conn.prepareStatement("SELECT * FROM attendance_record WHERE student_id=?");
pstmt.setInt(1, studentId);
} else if(teacherId > 0) {
pstmt = conn.prepareStatement("SELECT * FROM attendance_record WHERE teacher_id=?");
pstmt.setInt(1, teacherId);
}
rs = pstmt.executeQuery();
while(rs.next()) {
int id = rs.getInt("id");
int student_id = rs.getInt("student_id");
int teacher_id = rs.getInt("teacher_id");
Date record_date = rs.getDate("record_date");
boolean is_present = rs.getBoolean("is_present");
Student student = getStudentById(student_id);
Teacher teacher = getTeacherById(teacher_id);
AttendanceRecord record = new AttendanceRecord(id, student, teacher, record_date, is_present);
recordList.add(record);
}
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if(rs != null) rs.close();
if(pstmt != null) pstmt.close();
if(conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
return recordList;
}
private Student getStudentById(int id) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
Student student = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
pstmt = conn.prepareStatement("SELECT * FROM student WHERE id=?");
pstmt.setInt(1, id);
rs = pstmt.executeQuery();
if(rs.next()) {
String name = rs.getString("name");
String gender = rs.getString("gender");
student = new Student(id, name, gender);
}
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if(rs != null) rs.close();
if(pstmt != null) pstmt.close();
if(conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
return student;
}
private Teacher getTeacherById(int id) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
Teacher teacher = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
pstmt = conn.prepareStatement("SELECT * FROM teacher WHERE id=?");
pstmt.setInt(1, id);
rs = pstmt.executeQuery();
if(rs.next()) {
String name = rs.getString("name");
teacher = new Teacher(id, name);
}
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if(rs != null) rs.close();
if(pstmt != null) pstmt.close();
if(conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
return teacher;
}
}
```
6. 部署项目
将上述代码保存到相应的文件中,并将JAR包放入WEB-INF/lib目录下。然后,将整个项目打包成WAR文件,并将其部署到Tomcat服务器中。
现在,我们可以访问http://localhost:8080/attendance/index.jsp,进入班级考勤管理系统,浏览学生和教师列表,以及查看他们的考勤记录。
以上是一个简单的班级考勤管理系统的实现过程和代码,你可以根据自己的需要进行修改和优化。
阅读全文
相关推荐
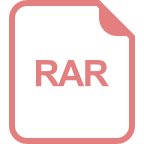


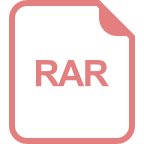
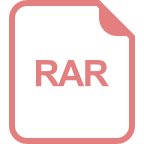
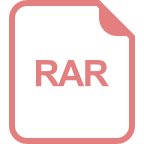