c# 根据日期计算年龄
时间: 2023-07-27 09:23:08 浏览: 287
可以使用以下代码来计算年龄:
```csharp
DateTime birthdate = new DateTime(1990, 1, 1); // 假设出生日期为1990年1月1日
DateTime today = DateTime.Today;
int age = today.Year - birthdate.Year;
if (birthdate > today.AddYears(-age))
{
age--;
}
Console.WriteLine("年龄为:" + age); // 输出年龄
```
在这里,我们首先定义了出生日期和当前日期,然后通过计算两个日期之间的年份差,得到年龄。但是,由于我们只知道出生日期的年月日,而不知道时分秒,因此我们需要进行一些额外的处理,以确保计算结果正确。具体来说,如果出生日期在当前日期之后,那么我们需要将年龄减1,以确保计算结果正确。
相关问题
c#根据出生日期计算年龄精确到日
可以使用 DateTime 类来计算年龄,然后再通过 TimeSpan 类获取精确到日的时间差。下面是一个示例代码:
```csharp
DateTime birthdate = new DateTime(1990, 10, 15);
DateTime today = DateTime.Today;
int age = today.Year - birthdate.Year;
if (birthdate > today.AddYears(-age)) age--;
TimeSpan ageInDays = today - birthdate.AddDays(age * 365);
Console.WriteLine($"年龄:{age}岁,{ageInDays.Days}天");
```
这个代码使用了 DateTime.Today 获取当前日期,然后计算出生日期与今天之间的年龄差。最后通过 TimeSpan 类获取时间差,并输出年龄和天数。注意,这个计算方法不考虑闰年,所以可能会有一定的误差。
C# 最新法定退休年龄日期计算
C# 中最新的法定退休年龄通常依据中国的《中华人民共和国社会保险法》规定,对于职工的基本养老保险,男性的法定退休年龄一般为60岁,女干部为55岁,女工人则为50岁。不过,自2018年起,国家实施渐进式延迟退休政策,意味着这一年龄可能会逐年逐步提高,具体到哪一年达到新的退休年龄,需要查阅官方发布的最新通知。
在C#程序中,如果你需要计算某人的退休年龄,你可以创建一个函数,接收出生年份作为输入,然后通过当前年份减去出生年份来得到大致的退休年龄。例如:
```csharp
DateTime currentYear = DateTime.Now.Year;
int retirementAgeForMale = 60; // 假设男性默认退休年龄
int retirementAgeForFemale = 55; // 女性默认退休年龄
DateTime birthDate = new DateTime(1970); // 示例出生年份,替换为你需要的实际值
DateTime retirementDate = new DateTime(currentYear, 1, 1).AddYears(birthDate.Year - currentYear);
// 计算退休年份
int retirementYear = retirementDate.Year;
if (birthDate.IsFemale()) { // 检查性别
if (retirementYear < currentYear + retirementAgeForFemale)
Console.WriteLine($"女性出生于{birthDate.Year}的人将在{retirementYear}年退休");
else
Console.WriteLine("尚未达到法定退休年龄");
}
else {
if (retirementYear < currentYear + retirementAgeForMale)
Console.WriteLine($"男性出生于{birthDate.Year}的人将在{retirementYear}年退休");
else
Console.WriteLine("尚未达到法定退休年龄");
}
阅读全文
相关推荐






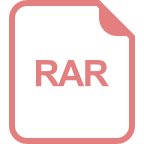



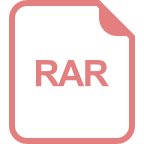






