yolov5s核心代码及核心代码解释
时间: 2024-06-07 10:11:52 浏览: 104
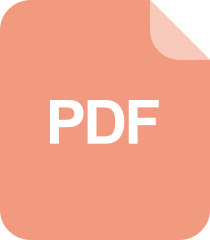
YOLOV5源码的详细解读
以下是 YOLOv5s 的核心代码及其解释:
```
class Detect(nn.Module):
stride = None # strides computed during build
export = False # onnx export
def __init__(self, nc=80, anchors=(), ch=()):
super(Detect, self).__init__()
self.nc = nc # number of classes
self.no = nc + 5 # number of outputs per anchor
self.nl = len(anchors) # number of detection layers
self.na = len(anchors[0]) // 2 # number of anchors
self.grid = [torch.zeros(1)] * self.nl # init grid
a = torch.tensor(anchors).float().view(self.nl, -1, 2)
self.register_buffer('anchors', a)
self.register_buffer('anchor_grid', a.clone().view(self.nl, 1, -1, 1, 1, 2))
self.m = nn.ModuleList(nn.Conv2d(x, self.no * self.na, 1) for x in ch) # output conv
def forward(self, x):
# x(bs,channels,width,height) -> y(bs,anchors,nc+5,width,height)
z = [] # inference output
for i in range(self.nl):
x[i] = self.m[i](x[i]) # conv
bs, _, ny, nx = x[i].shape # x(bs,anchors*(nc+5),width,height) to x(bs,anchors,nc+5,width,height)
x[i] = x[i].view(bs, self.na, self.no, ny, nx).permute(0, 1, 3, 4, 2).contiguous()
if not self.training:
if self.grid[i].shape[2:4] != x[i].shape[2:4]:
self.grid[i] = self._make_grid(nx, ny).to(x[i].device)
y = x[i].sigmoid()
y[..., 0:2] = (y[..., 0:2] * 2. - 0.5 + self.grid[i]) * self.stride[i] # xy
y[..., 2:4] = (y[..., 2:4] * 2) ** 2 * self.anchor_grid[i] # wh
z.append(y.view(bs, -1, self.no))
return x if self.training else (torch.cat(z, 1), x)
def _make_grid(self, nx=20, ny=20):
xv, yv = torch.meshgrid([torch.arange(ny), torch.arange(nx)])
return torch.stack((xv, yv), 2).view((1, 1, ny, nx, 2)).float()
def _initialize_biases(self, cf):
# cf is class frequency
pi = 3.141592653589793
b = [-4.0, -3.0, -2.0, -1.0, 0, 1.0, 2.0, 3.0]
w = [2.0 ** i for i in b]
x = [w[i] * pi / 2 for i in range(len(w))]
y = [(1 - cf[i] / sum(cf)) / (self.nc - 1) for i in range(self.nc)]
for j in range(self.nl):
bias = self.m[j].bias.view(self.na, -1) # conv.bias(255) to (3,85)
if bias.shape[1] == self.no:
# yolov3-tiny
# https://github.com/pjreddie/darknet/blob/master/cfg/yolov3-tiny.cfg
bias[:, 4] += math.log(8. / (640. / 13) ** 2) # obj (8 objects per 13x13 image)
bias[:, 5:] += math.log(0.6 / (self.nc - 0.99)) # cls
elif bias.shape[1] == self.no + 1:
# yolov3 and yolov5
# https://github.com/pjreddie/darknet/blob/master/cfg/yolov3.cfg
# https://github.com/ultralytics/yolov5/blob/master/models/yolo.py
bias[:, 4] += math.log(8. / (640. / 32) ** 2) # obj (8 objects per 32x32 feature map)
bias[:, 5:-1] += math.log(0.6 / (self.nc - 0.99)) # cls
bias[:, -1] += -math.log(1. / (self.na * 0.05)) # ratio
for i in range(self.na):
# if self.na == 1:
# b = bias
# else:
# b = bias[i:i+1]
b = bias[i:i+1]
b[:, 0] += x # x
b[:, 1] += y * 2*pi # y
b[:, 2] += x # w
b[:, 3] += y * 2*pi # h
bias[i:i+1] = b
self.m[j].bias = torch.nn.Parameter(bias.view(-1), requires_grad=True)
```
这个代码实现了 YOLOv5s 中的检测模块,它的主要作用是将输入的特征图进行处理,输出包含物体类别、位置、置信度等信息的检测结果。
- `nc`: 表示物体的类别数;
- `anchors`: 表示锚框的大小和位置;
- `ch`: 表示特征图的通道数;
- `self.grid`: 表示特征图上的网格;
- `self.na`: 表示锚框的数量;
- `y`: 表示检测结果。
在 forward 函数中,首先将输入的特征图通过卷积层进行卷积操作,然后对卷积结果进行变形,得到检测结果。在训练时,会输出特征图,而在测试时,会输出检测结果。
_make_grid 函数将特征图上的每个点映射到原图上,用于计算物体的位置。
_initialize_biases 函数用于初始化偏置,以便更好地检测不同类别的物体。
阅读全文
相关推荐
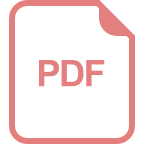
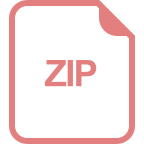
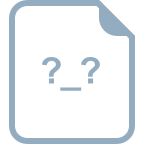
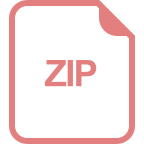
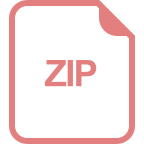
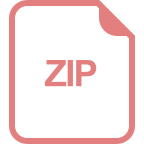
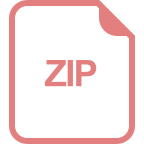
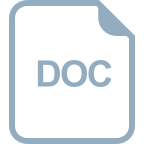
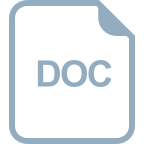
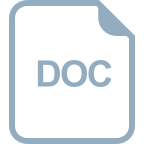
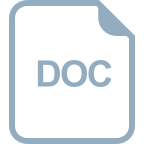
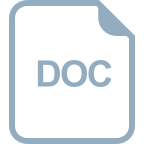
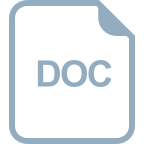
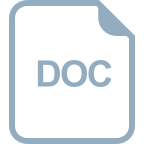

