用java调用百度云API写一个查询域名到期时间的接口
时间: 2023-05-22 11:07:23 浏览: 312
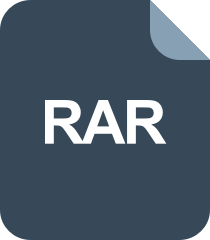
asp到期的域名到期查询
很高兴扮演ChitGPT,接下来我将为您提供有关如何使用Java调用百度云API查询域名到期时间的详细说明。
首先,您需要申请一个百度云账号并在控制台中创建一个新的应用程序,然后在应用程序中启用“域名API”服务。接着,您需要获取您的Access Key和Secret Key,这将用于与百度云API进行身份验证和授权。
在获得这些凭证之后,您可以使用Java中的HttpClient类来向百度API发送GET请求并获取JSON响应。以下为示例代码:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class DomainExpirationQuery {
private static final String API_URL = "https://domainapi.baidu.com/api/v3/domain/expiredate";
public static void main(String[] args) {
String domainName = "example.com";
String accessKeyId = "yourAccessKeyId";
String secretKey = "yourSecretKey";
try {
URL url = new URL(API_URL + "?apikey=" + accessKeyId + "&domain=" + domainName);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Content-Type", "application/json");
conn.setRequestProperty("User-Agent", "Mozilla/5.0");
conn.setRequestProperty("X-bce-date", "20220101T010101Z");
String auth = AuthUtils.generateAuthorization(accessKeyId, secretKey, "GET",
url.toString(), null, conn.getRequestProperty("X-bce-date"));
conn.setRequestProperty("Authorization", auth);
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
```
在上面的示例代码中,您需要将“example.com”替换为您要查询到期时间的域名,将“yourAccessKeyId”和“yourSecretKey”替换为您的百度云凭证。
请注意,您还需要在AuthUtils类中实现generateAuthorization方法以生成用于身份验证的Authorization头。这需要使用HMAC-SHA256算法和UTF-8字符集生成签名,并对其进行Base64编码。以下为示例代码:
```java
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.Locale;
import java.util.SimpleTimeZone;
public class AuthUtils {
private static final String BCE_AUTH_VERSION = "bce-auth-v1";
private static final String BCE_DATE_FORMAT = "yyyyMMdd'T'HHmmss'Z'";
private static final String BCE_TIME_ZONE = "UTC";
public static String generateAuthorization(String accessKeyId, String secretAccessKey, String httpMethod,
String uri, String params, String timestamp) {
try {
String authString = BCE_AUTH_VERSION + "/" + accessKeyId + "/"
+ getFormattedTimestamp(timestamp) + "/1800";
String httpHeaders = "";
String httpQuery = (params != null && !params.isEmpty()) ? "?" + params : "";
String signingKey = generateSigningKey(secretAccessKey, getFormattedTimestamp(timestamp), "bce/auth/v1");
String auth = generateAuthString(authString, httpMethod.toUpperCase(), uri, httpQuery, httpHeaders,
timestamp, signingKey);
return auth;
} catch (Exception e) {
throw new RuntimeException("Unable to generate authorization signature", e);
}
}
private static String generateAuthString(String authString, String httpMethod, String uri, String httpQuery,
String httpHeaders, String timestamp, String signingKey) throws NoSuchAlgorithmException {
String canonicalRequest = httpMethod + "\n" + uri + httpQuery + "\n" + httpQuery + "\n" + httpHeaders + "\n\n" + "content-md5;content-type\n" + timestamp + "\n" + "x-bce-date:" + timestamp + "\n\n" + "content-md5;content-type;x-bce-date\n" + "UNSIGNED-PAYLOAD";
String signing = hmacSha256(signingKey, canonicalRequest);
String auth = authString + "/" + "host" + "/" + signing;
return auth;
}
private static String hmacSha256(String secret, String message) {
try {
byte[] key = secret.getBytes(StandardCharsets.UTF_8);
SecretKeySpec secretKeySpec = new SecretKeySpec(key, "HmacSHA256");
Mac mac = Mac.getInstance("HmacSHA256");
mac.init(secretKeySpec);
byte[] messageBytes = message.getBytes("UTF-8");
byte[] result = mac.doFinal(messageBytes);
return Base64.encodeBase64String(result);
} catch (Exception e) {
throw new RuntimeException("Unable to calculate a request signature: " + e.getMessage(), e);
}
}
private static String generateSigningKey(String secretAccessKey, String dateStamp, String regionName) throws Exception {
byte[] kSecret = ("Baidu" + secretAccessKey).getBytes(StandardCharsets.UTF_8);
byte[] kDate = hmacSha256("Baidu" + dateStamp, kSecret).getBytes(StandardCharsets.UTF_8);
byte[] kRegion = hmacSha256(regionName, kDate).getBytes(StandardCharsets.UTF_8);
byte[] kService = hmacSha256("bce/auth/v1", kRegion).getBytes(StandardCharsets.UTF_8);
byte[] kSigning = hmacSha256("bce/auth/v1", kService).getBytes(StandardCharsets.UTF_8);
return Base64.encodeBase64String(kSigning);
}
private static String getFormattedTimestamp(String timestamp) {
SimpleDateFormat sdf = new SimpleDateFormat(BCE_DATE_FORMAT, Locale.US);
sdf.setTimeZone(new SimpleTimeZone(0, BCE_TIME_ZONE));
if (timestamp == null) {
return sdf.format(new Date());
}
Calendar cd = Calendar.getInstance();
try {
Date date = sdf.parse(timestamp);
cd.setTime(date);
} catch (Throwable ignored) {
}
return sdf.format(cd.getTime());
}
}
```
最后,代码将输出响应的JSON字符串,其中包含查询到域名到期日期的信息。
希望这个例子能帮助您理解如何使用Java调用百度云API来查询域名到期时间!
阅读全文
相关推荐
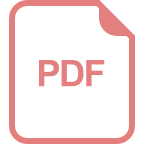
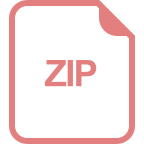
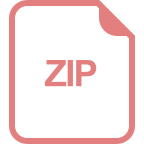
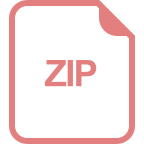
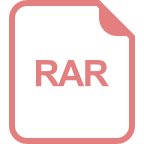
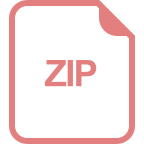
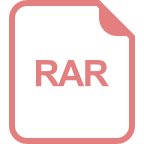
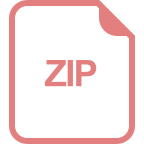
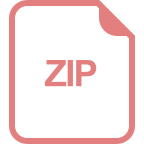
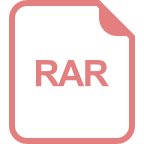
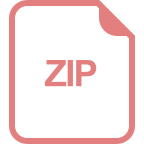
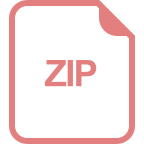
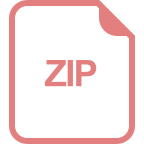
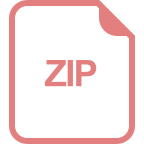
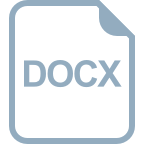
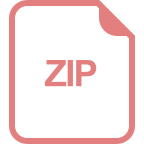