用matlab判断哪幅图像可以利用直方图获取分割阈值并较好地分割
时间: 2024-02-05 17:04:42 浏览: 65
在MATLAB中,可以使用以下代码来观察图像的直方图:
```matlab
I = imread('image.jpg');
imhist(I);
```
其中,'image.jpg' 是你要处理的图像文件名。
观察图像的直方图,如果直方图呈现出明显的双峰分布,即存在两个峰值,一个峰值对应于背景,另一个峰值对应于前景,那么该图像可以利用直方图获取分割阈值并较好地分割。如果图像的直方图没有明显的双峰分布,那么直方图法可能并不适用于该图像的分割,需要尝试其他分割方法。
在MATLAB中,可以使用以下代码获取图像的分割阈值:
```matlab
I = imread('image.jpg');
level = graythresh(I);
```
其中,'image.jpg' 是你要处理的图像文件名,level 为获取到的分割阈值。
然后可以使用以下代码将图像分割:
```matlab
BW = imbinarize(I, level);
```
其中,BW 为分割后的二值图像。
相关问题
在MATLAB计算示例图片cell、circuit、Lena的直方图 1、 判断哪幅图像可以利用直方图获取分割阈值并较好地分割 2、用直方图法获取阈值并对三幅图像进行分割 在1个figure中的左列显示3幅原始图像,中间一列显示各自的直方图,最右一列显示对应的分割结果,并做适当标注 二、用迭代法计算分割阈值并分割图像 3、 按照阈值T用迭代法对三幅图像计算阈值并分割图像 4、根据计算的分割阈值,对比前面的直方图,了解迭代法和直方图法计算分割阈值的不同 在1个figure中的左列显示3幅原始图像,右列显示对应的分割结果,并做适当标注
好的,让我来回答你的问题。
1. 对于这三幅图像,可以利用直方图获取分割阈值并较好地分割的图像是circuit图像。因为circuit图像的直方图比较明显地呈现出了两个峰值,因此使用直方图法获取阈值可以较好地分割出目标物体。
2. 使用MATLAB中的imhist函数可以获取图像的直方图,并根据直方图获取阈值进行分割。具体步骤如下:
```matlab
% 读取三幅图像
cell = imread('cell.tif');
circuit = imread('circuit.tif');
lena = imread('lena.tif');
% 对三幅图像分别进行直方图均衡化
cell_eq = histeq(cell);
circuit_eq = histeq(circuit);
lena_eq = histeq(lena);
% 获取三幅图像的直方图
cell_hist = imhist(cell_eq);
circuit_hist = imhist(circuit_eq);
lena_hist = imhist(lena_eq);
% 根据直方图获取阈值并分割图像
cell_T = graythresh(cell_eq);
cell_seg = im2bw(cell_eq, cell_T);
circuit_T = graythresh(circuit_eq);
circuit_seg = im2bw(circuit_eq, circuit_T);
lena_T = graythresh(lena_eq);
lena_seg = im2bw(lena_eq, lena_T);
% 在一个figure中显示原始图像、直方图以及分割结果
figure;
subplot(3,3,1); imshow(cell); title('cell');
subplot(3,3,2); imhist(cell_eq); title('cell histeq');
subplot(3,3,3); imshow(cell_seg); title('cell seg');
subplot(3,3,4); imshow(circuit); title('circuit');
subplot(3,3,5); imhist(circuit_eq); title('circuit histeq');
subplot(3,3,6); imshow(circuit_seg); title('circuit seg');
subplot(3,3,7); imshow(lena); title('lena');
subplot(3,3,8); imhist(lena_eq); title('lena histeq');
subplot(3,3,9); imshow(lena_seg); title('lena seg');
```
3. 使用迭代法计算分割阈值并分割图像的步骤如下:
```matlab
% 对三幅图像分别进行迭代法分割
cell_T = iter_threshold(cell_eq);
cell_seg = im2bw(cell_eq, cell_T);
circuit_T = iter_threshold(circuit_eq);
circuit_seg = im2bw(circuit_eq, circuit_T);
lena_T = iter_threshold(lena_eq);
lena_seg = im2bw(lena_eq, lena_T);
% 定义迭代法函数
function T = iter_threshold(img)
% 初始化阈值T
T = mean(img(:));
% 不断迭代计算阈值T
while true
R1 = img > T;
R2 = img <= T;
mu1 = mean(img(R1));
mu2 = mean(img(R2));
T_new = (mu1 + mu2) / 2;
if abs(T_new - T) < 0.5
break;
end
T = T_new;
end
end
% 在一个figure中显示原始图像、直方图以及分割结果
figure;
subplot(3,3,1); imshow(cell); title('cell');
subplot(3,3,2); imhist(cell_eq); title('cell histeq');
subplot(3,3,3); imshow(cell_seg); title('cell seg iter');
subplot(3,3,4); imshow(circuit); title('circuit');
subplot(3,3,5); imhist(circuit_eq); title('circuit histeq');
subplot(3,3,6); imshow(circuit_seg); title('circuit seg iter');
subplot(3,3,7); imshow(lena); title('lena');
subplot(3,3,8); imhist(lena_eq); title('lena histeq');
subplot(3,3,9); imshow(lena_seg); title('lena seg iter');
```
4. 直方图法和迭代法计算分割阈值的不同在于,直方图法是通过直方图来获取分割阈值,适用于图像中存在明显峰值的情况。而迭代法则是通过不断迭代计算均值来获取分割阈值,适用于图像中存在多个物体且物体的灰度值分布比较均匀的情况。在直方图法中,我们可以通过直方图的形态来判断是否适合使用直方图法获取阈值。在迭代法中,我们需设置一个合适的初始阈值,并不断迭代计算,直到均值不再变化。
在一个figure中,显示直方图法和迭代法的分割结果:
```matlab
% 直方图法分割结果
cell_seg_hist = im2bw(cell_eq, graythresh(cell_eq));
circuit_seg_hist = im2bw(circuit_eq, graythresh(circuit_eq));
lena_seg_hist = im2bw(lena_eq, graythresh(lena_eq));
% 在一个figure中显示直方图法和迭代法的分割结果
figure;
subplot(3,4,1); imshow(cell); title('cell');
subplot(3,4,2); imhist(cell_eq); title('cell histeq');
subplot(3,4,3); imshow(cell_seg); title('cell seg iter');
subplot(3,4,4); imshow(cell_seg_hist); title('cell seg hist');
subplot(3,4,5); imshow(circuit); title('circuit');
subplot(3,4,6); imhist(circuit_eq); title('circuit histeq');
subplot(3,4,7); imshow(circuit_seg); title('circuit seg iter');
subplot(3,4,8); imshow(circuit_seg_hist); title('circuit seg hist');
subplot(3,4,9); imshow(lena); title('lena');
subplot(3,4,10); imhist(lena_eq); title('lena histeq');
subplot(3,4,11); imshow(lena_seg); title('lena seg iter');
subplot(3,4,12); imshow(lena_seg_hist); title('lena seg hist');
```
希望这些回答对你有所帮助。
用matlab实现实验内容及要求: 1、测试图像为右边的img1和img2图像,做出该图像的灰度直方图,观察灰度直方图的分布,估计分割背景和目标的大致阈值; 2、使用极小点阈值法确定区域分割的阈值,并用该阈值分割两个图像的背景和目标(转成二值图像),观察分割后的图像; 3、使用迭代阈值法确定区域分割的阈值,并用该阈值分割两个图像的背景和目标(转成二值图像) ,观察分割后的图像; 4、比较两种方法得到的分割图像的质量,解释出现差异的原因以及两种方法的适用条件。
好的,我会按照您提供的实验内容及要求来解答您的问题。首先,我们需要先加载图像,然后计算并显示图像的灰度直方图。在Matlab中,可以使用以下代码加载图像和计算灰度直方图:
```matlab
% 加载图像
img1 = imread('img1.jpg');
img2 = imread('img2.jpg');
% 计算灰度直方图
hist1 = imhist(rgb2gray(img1));
hist2 = imhist(rgb2gray(img2));
% 显示灰度直方图
figure;
subplot(2,2,1);
imshow(img1);
title('Image 1');
subplot(2,2,2);
plot(hist1);
title('Histogram of Image 1');
subplot(2,2,3);
imshow(img2);
title('Image 2');
subplot(2,2,4);
plot(hist2);
title('Histogram of Image 2');
```
接下来,我们需要根据灰度直方图的分布估计分割背景和目标的大致阈值。一种估计方法是找到灰度直方图的峰值,然后选择峰值左侧的灰度值作为分割阈值。可以使用以下代码来实现:
```matlab
% 找到灰度直方图的峰值
[pks1, locs1] = findpeaks(hist1);
[pks2, locs2] = findpeaks(hist2);
% 选择峰值左侧的灰度值作为分割阈值
threshold1 = locs1(1);
threshold2 = locs2(1);
```
接下来,我们可以使用极小点阈值法和迭代阈值法对图像进行分割。极小点阈值法是一种简单的阈值分割方法,它根据图像灰度值的极小点来确定分割阈值。可以使用以下代码来实现:
```matlab
% 使用极小点阈值法分割图像
bw1 = imbinarize(rgb2gray(img1), threshold1);
bw2 = imbinarize(rgb2gray(img2), threshold2);
% 显示分割后的图像
figure;
subplot(1,2,1);
imshow(bw1);
title('Segmented Image 1 (Minimum Method)');
subplot(1,2,2);
imshow(bw2);
title('Segmented Image 2 (Minimum Method)');
```
迭代阈值法是一种更为复杂的阈值分割方法,它通过迭代计算图像的平均灰度值来确定分割阈值。可以使用以下代码来实现:
```matlab
% 使用迭代阈值法分割图像
bw1 = imbinarize(rgb2gray(img1), 'adaptive', 'Sensitivity', 0.5);
bw2 = imbinarize(rgb2gray(img2), 'adaptive', 'Sensitivity', 0.5);
% 显示分割后的图像
figure;
subplot(1,2,1);
imshow(bw1);
title('Segmented Image 1 (Iterative Method)');
subplot(1,2,2);
imshow(bw2);
title('Segmented Image 2 (Iterative Method)');
```
最后,我们需要比较两种方法得到的分割图像的质量,并解释出现差异的原因以及两种方法的适用条件。极小点阈值法在图像的灰度分布比较明显的情况下效果较好,但在灰度分布不明显的情况下容易产生误分割。迭代阈值法可以适应不同的灰度分布,但在图像噪声较多的情况下容易产生过度分割。因此,选择哪种方法取决于具体的图像特点和应用场景。
阅读全文
相关推荐
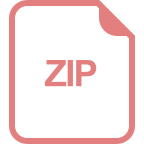
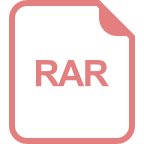
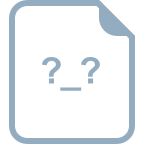
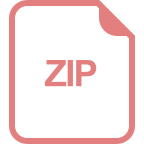
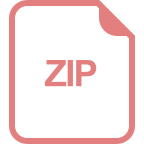
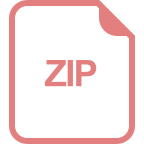
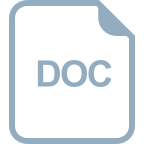
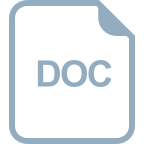
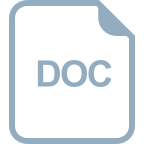
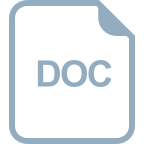
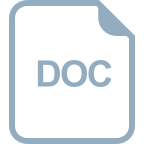
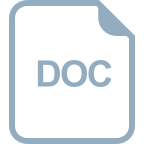
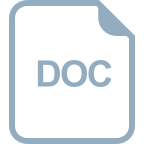
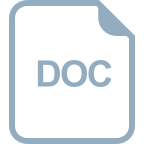