JAVA中类转换成接口(向下转型)
时间: 2023-11-12 17:05:45 浏览: 84
在JAVA中,将一个类转换成接口称为向下转型。其实现方式如下:
1.定义一个接口,该接口包含了要转换的类中的方法。
2.在要转换的类中实现该接口。
3.在需要进行向下转型的地方,使用强制类型转换将类转换成接口类型,例如:
```
MyClass obj = new MyClass(); // 创建一个MyClass对象
MyInterface intf = (MyInterface) obj; // 将MyClass对象转换成MyInterface接口类型
```
需要注意的是,在进行向下转型时需要确保对象实际上实现了该接口,否则会抛出ClassCastException异常。
相关问题
JAVA中接口转换成实现类(向下转型)
Java中的接口不能直接转换成实现类,因为接口只是一组方法的定义,没有实现。要将接口向下转型成实现类,需要先创建一个实现了该接口的类的对象,然后使用强制类型转换将接口对象转换成该实现类的对象。例如:
```
public interface Animal {
void eat();
}
public class Dog implements Animal {
@Override
public void eat() {
System.out.println("Dog is eating");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog(); // 创建一个实现了Animal接口的Dog类的对象
Dog dog = (Dog) animal; // 强制类型转换将Animal接口对象转换成Dog的对象
dog.eat(); // 调用Dog类的eat方法
}
}
```
接口转换成实现类(向下转型)
接口转换成实现类,也称为向下转型,是将一个接口类型的引用转换为实现该接口的类的引用。这通常是在需要访问实现类特定的属性或方法时使用的。
在 Java 中,使用强制类型转换运算符(即括号)将接口类型的引用转换为实现类类型的引用。例如:
```
interface Animal {
void makeSound();
}
class Dog implements Animal {
public void makeSound() {
System.out.println("Bark!");
}
public void fetch() {
System.out.println("Fetch the ball!");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog();
animal.makeSound(); // Output: Bark!
((Dog) animal).fetch(); // Output: Fetch the ball!
}
}
```
在这个例子中,Animal 接口被实现为 Dog 类。在 main 方法中,我们使用 Animal 接口类型的引用来创建一个 Dog 对象。我们可以调用 Animal 接口中定义的 makeSound 方法,因为它被实现了。然而,我们不能直接调用 Dog 类的 fetch 方法,因为它不是 Animal 接口的一部分。我们需要将 Animal 类型的引用转换为 Dog 类型的引用,以便访问 fetch 方法。这就是向下转型的用途。
阅读全文
相关推荐
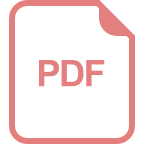
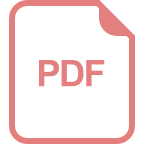
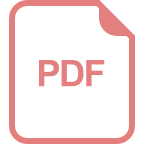













