c++怎么用sort对数组内数字实现排序
时间: 2024-05-19 11:15:47 浏览: 20
C语言中可以使用sort函数对数组内数字实现排序,具体步骤如下:
1. 定义一个数组,例如int arr[10] = {3, 5, 1, 6, 8, 2, 9, 4, 7, 0};
2. 调用sort函数,该函数位于stdlib.h头文件中,函数原型为:void qsort(void *base, size_t nitems, size_t size, int (*compar)(const void *, const void*))。
其中,参数base为需要排序的数组的首地址;nitems为数组元素个数;size为每个元素的字节数;compar为比较函数,用于指定数组元素的比较方式。
3. 编写比较函数,该函数的作用是比较两个元素的大小,并返回一个整数值,用于指示它们的大小关系。比较函数的原型为:int cmpfunc(const void * a, const void * b)。
例如,若要按照从小到大的顺序排序,则可以编写如下的比较函数:
int cmpfunc(const void * a, const void * b) {
return ( *(int*)a - *(int*)b );
}
4. 调用sort函数进行排序,代码如下:
sort(arr, 10, sizeof(int), cmpfunc);
其中,第一个参数为需要排序的数组的首地址;第二个参数为数组元素个数;第三个参数为每个元素的字节数;第四个参数为比较函数的名称。
5. 输出排序后的数组,代码如下:
for(int i = 0; i < 10; i++) {
printf("%d ", arr[i]);
}
完整代码如下:
#include <stdio.h>
#include <stdlib.h>
int cmpfunc(const void * a, const void * b) {
return ( *(int*)a - *(int*)b );
}
int main() {
int arr[10] = {3, 5, 1, 6, 8, 2, 9, 4, 7, 0};
sort(arr, 10, sizeof(int), cmpfunc);
for(int i = 0; i < 10; i++) {
printf("%d ", arr[i]);
}
return 0;
}
相关推荐
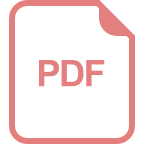
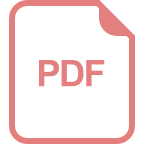
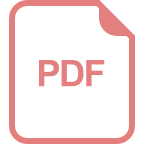














