如何使用C++编程语言来获取CPU和GPU的实时温度读数?
时间: 2024-11-20 14:33:46 浏览: 65
在C++编程中获取CPU和GPU的实时温度读数通常需要借助系统API或者第三方库,因为标准C++库本身并不直接提供这样的功能。以下是两个常见平台上的简要说明:
1. **Linux** (使用`libhwmon`库):
- 首先,你需要安装`lm-sensors`包,它包含了`libhwmon`库。然后你可以通过`sensors`命令查看硬件监控信息,或者使用C++编写程序,如下面这个示例(假设已包含`<linux/hwmon.h>`头文件):
```cpp
#include <iostream>
#include <linux/hwmon.h>
int main() {
struct hwm_device *dev;
dev = hwmon_device_open("/sys/class/hwmon/hwmon0"); // 用实际路径替换hwmon0
if (!dev) {
std::cerr << "Failed to open device" << std::endl;
return 1;
}
double temp = get_temp(dev); // 假设有个get_temp函数用于获取温度
std::cout << "CPU temperature: " << temp << "°C" << std::endl;
hwmon_device_close(dev);
return 0;
}
`
2. **Windows** (使用`Win32 API`):
- Windows上可以使用`PerformanceCounter`类来监测性能计数器,比如处理器或显卡的温度。首先需要包含`<windows.h>`,然后创建`PerformanceCounter`实例,并设置正确的计数器ID。例如:
```cpp
#include <windows.h>
#include <intrin.h> // 对于GetTemperature APIs
int main() {
LARGE_INTEGER freq;
QueryPerformanceFrequency(&freq);
double cpuTemp = GetProcessorTemperature();
double gpuTemp = GetGPUTemperature(); // 假设有这两个自定义函数
printf("CPU temperature: %.2f°C\n", cpuTemp / freq.QuadPart);
printf("GPU temperature: %.2f°C\n", gpuTemp / freq.QuadPart);
return 0;
}
```
请注意,上述代码中的`get_temp`、`GetProcessorTemperature`和`GetGPUTemperature`函数是虚构的,实际实现会依赖于操作系统提供的接口或者第三方库。
阅读全文
相关推荐
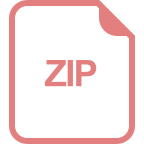
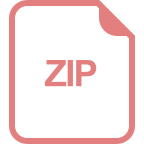
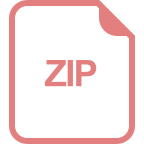
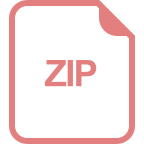
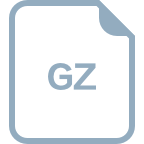
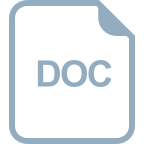
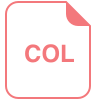
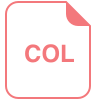
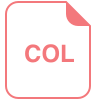
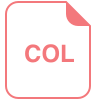
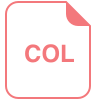
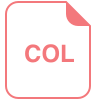
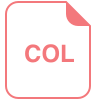
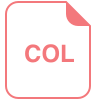
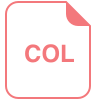
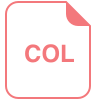
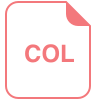
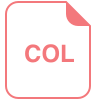