答辩问使用spring boot和,vue和mysql如何实现商品分类
时间: 2023-07-14 11:13:14 浏览: 48
使用 Spring Boot 框架可以提供后端服务,Vue 框架可以提供前端展示,MySQL 数据库可以存储商品分类信息。下面简单介绍实现的步骤:
1. 定义商品分类的数据模型,例如:
```
public class Category {
private Long id;
private String name;
private Long parentId;
// getter/setter 略
}
```
2. 创建商品分类的数据库表,并在 Spring Boot 中配置数据库连接。
3. 实现商品分类的增删改查接口,例如:
```
@RestController
@RequestMapping("/categories")
public class CategoryController {
@Autowired
private CategoryService categoryService;
@GetMapping("/{id}")
public Category getCategory(@PathVariable("id") Long id) {
return categoryService.getCategoryById(id);
}
@PostMapping
public Category addCategory(@RequestBody Category category) {
return categoryService.addCategory(category);
}
@PutMapping("/{id}")
public Category updateCategory(@PathVariable("id") Long id, @RequestBody Category category) {
category.setId(id);
return categoryService.updateCategory(category);
}
@DeleteMapping("/{id}")
public void deleteCategory(@PathVariable("id") Long id) {
categoryService.deleteCategory(id);
}
}
```
4. 在 Vue 中编写商品分类管理页面,可以使用 Element UI 组件库提供的树形控件展示商品分类结构,例如:
```
<template>
<div>
<el-tree :data="categories" :props="treeProps">
<span class="custom-tree-node" slot-scope="{ node, data }">
{{ data.name }}
</span>
</el-tree>
</div>
</template>
<script>
import { mapState } from 'vuex';
export default {
computed: {
...mapState(['categories']),
treeProps() {
return {
label: 'name',
children: 'children',
};
},
},
};
</script>
```
5. 在 Vue 中调用后端接口获取商品分类数据,并通过 Vuex 存储在全局状态中,例如:
```
import axios from 'axios';
const state = {
categories: [],
};
const mutations = {
setCategories(state, categories) {
state.categories = categories;
},
};
const actions = {
async fetchCategories({ commit }) {
const { data } = await axios.get('/categories');
commit('setCategories', data);
},
};
export default {
namespaced: true,
state,
mutations,
actions,
};
```
综上,使用 Spring Boot 和 Vue 可以轻松实现商品分类的增删改查和展示。
相关推荐
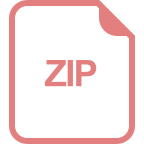
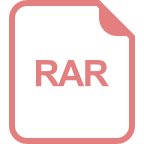
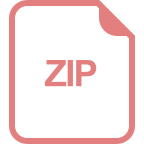














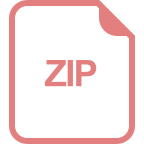