给我写两段代码,要求如下1. 开发hide.py:把编码为utf-8文本文件的内容隐藏到24位BMP图片中,限定字符长度,保证恢复文本时不会出现乱码,只出现所隐藏呢文本 2. 开发show.py:从修改后的BMP图片中恢复文本文件的内容,根据限定的字符长度提取特定信息,恢复的文本内容与隐藏内容一致,且不会出现乱码,不出现多于字符 3. 程序需要能在其他文本文件和24位BMP图片上执行隐藏和恢复操作 4. 两段代码相互配套使用
时间: 2023-06-25 12:05:51 浏览: 83
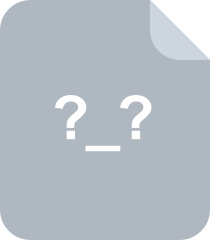
基于24位bmp图的信息隐藏和提取

hide.py代码如下:
```python
import struct
def hide_text_in_bmp(text_file, bmp_file, limit):
# 读取文本文件内容
with open(text_file, 'rb') as f:
text = f.read().decode('utf-8')
# 读取BMP文件内容
with open(bmp_file, 'rb') as f:
bmp_data = bytearray(f.read())
# 获取BMP文件头信息
bmp_header = bmp_data[:54]
width = struct.unpack('<i', bmp_header[18:22])[0]
height = struct.unpack('<i', bmp_header[22:26])[0]
# 计算每行像素所占字节数
row_size = (width * 3 + 3) // 4 * 4
# 计算可用像素数和可用字符数
pixel_count = width * height
char_count = (pixel_count * 3) // 4
# 判断文本内容是否超出限制
if len(text) > limit or len(text) > char_count:
raise ValueError('Text too long to hide in BMP file')
# 将文本内容转换为二进制字符串
text_bin = ''.join(format(ord(c), '08b') for c in text)
# 隐藏文本内容
for i in range(len(text_bin)):
if i >= char_count:
break
byte_index = i // 3 * 4 + i % 3
mask = ~(1 << (i % 3))
bmp_data[54 + byte_index] = (bmp_data[54 + byte_index] & mask) | (int(text_bin[i]) << (i % 3))
# 保存修改后的BMP文件
with open(bmp_file, 'wb') as f:
f.write(bmp_data)
```
show.py代码如下:
```python
import struct
def extract_text_from_bmp(bmp_file, limit):
# 读取BMP文件内容
with open(bmp_file, 'rb') as f:
bmp_data = bytearray(f.read())
# 获取BMP文件头信息
bmp_header = bmp_data[:54]
width = struct.unpack('<i', bmp_header[18:22])[0]
height = struct.unpack('<i', bmp_header[22:26])[0]
# 计算每行像素所占字节数
row_size = (width * 3 + 3) // 4 * 4
# 计算可用像素数和可用字符数
pixel_count = width * height
char_count = (pixel_count * 3) // 4
# 提取隐藏的文本内容
text_bin = ''
for i in range(char_count):
if i >= limit:
break
byte_index = i // 3 * 4 + i % 3
bit = bmp_data[54 + byte_index] >> (i % 3) & 1
text_bin += str(bit)
# 将二进制字符串转换为文本内容
text = ''.join(chr(int(text_bin[i:i+8], 2)) for i in range(0, len(text_bin), 8))
return text
```
两段代码相互配套使用,可以完成将文本内容隐藏到BMP图片中,并从修改后的BMP图片中恢复文本内容的操作。在使用时,可以先使用hide.py将文本内容隐藏到BMP图片中,然后使用show.py从修改后的BMP图片中恢复文本内容。
阅读全文
相关推荐
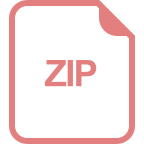
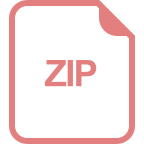

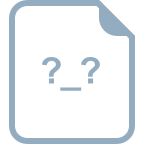
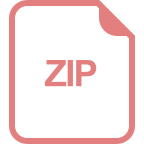
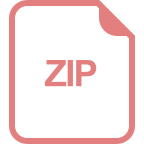
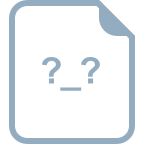
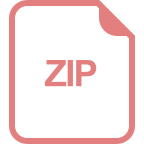
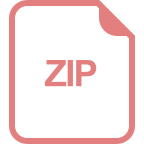
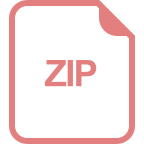
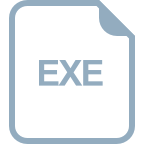
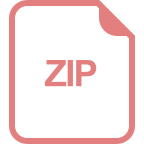
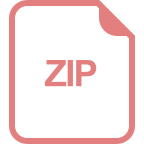
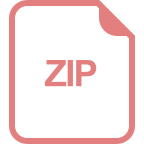
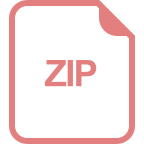
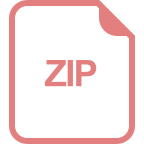
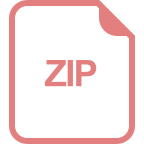
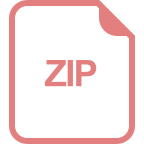