编写一段c代码实现读入ppm文件
时间: 2024-02-12 17:08:28 浏览: 130
以下是一个简单的C程序,用于读取PPM文件并将其存储在PPM结构体中:
```
#include <stdio.h>
#include <stdlib.h>
struct Pixel {
int red;
int green;
int blue;
};
struct PPM {
int width;
int height;
int max;
struct Pixel *pixels;
};
struct PPM *readPPM(char *filename) {
FILE *f = fopen(filename, "r");
if (f == NULL) {
fprintf(stderr, "Failed to open file %s.\n", filename);
return NULL;
}
// Read the PPM header
char magic[3];
fscanf(f, "%2s\n", magic);
if (magic[0] != 'P' || magic[1] != '3') {
fprintf(stderr, "Invalid PPM file format.\n");
fclose(f);
return NULL;
}
int width, height, max;
fscanf(f, "%d %d\n%d\n", &width, &height, &max);
// Allocate memory for the pixel data
int numPixels = width * height;
struct Pixel *pixels = (struct Pixel *) malloc(numPixels * sizeof(struct Pixel));
if (pixels == NULL) {
fprintf(stderr, "Failed to allocate memory for pixel data.\n");
fclose(f);
return NULL;
}
// Read the pixel data
for (int i = 0; i < numPixels; i++) {
fscanf(f, "%d %d %d", &pixels[i].red, &pixels[i].green, &pixels[i].blue);
}
// Create a new PPM struct and fill it with data
struct PPM *img = (struct PPM *) malloc(sizeof(struct PPM));
if (img == NULL) {
fprintf(stderr, "Failed to allocate memory for PPM image.\n");
fclose(f);
free(pixels);
return NULL;
}
img->width = width;
img->height = height;
img->max = max;
img->pixels = pixels;
fclose(f);
return img;
}
int main() {
struct PPM *img = readPPM("example.ppm");
if (img == NULL) {
return 1;
}
// TODO: do something with the PPM image data
free(img->pixels);
free(img);
return 0;
}
```
在上面的代码中,我们首先定义了一个Pixel结构体和一个PPM结构体,用于存储像素和PPM图像数据。然后我们定义了一个名为`readPPM`的函数,该函数接受一个PPM文件的文件名作为参数,并返回一个指向PPM结构体的指针。该函数首先打开给定的文件,并读取PPM文件头信息。如果文件格式无效,函数将在标准错误输出中打印错误信息,并返回NULL。接着,该函数分配内存空间来存储像素数据,并在读取像素数据后将其存储在Pixel数组中。最后,该函数创建一个新的PPM结构体,并将读取的数据填充进去。如果内存分配失败,函数将在标准错误输出中打印错误信息,并返回NULL。最后,该函数返回一个指向PPM结构体的指针。
在main函数中,我们调用`readPPM`函数来读取PPM文件,并检查函数返回值是否为NULL。如果是,我们将返回1表示程序出错。否则,我们可以对读取出来的PPM图像数据进行操作。最后,我们释放分配的内存空间,以避免内存泄漏。
阅读全文
相关推荐
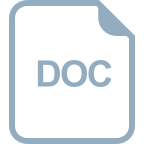
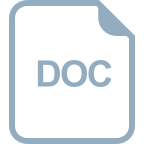
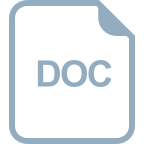
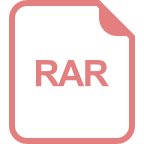
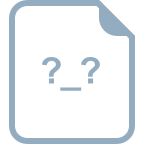
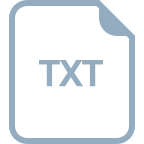





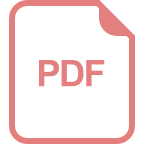
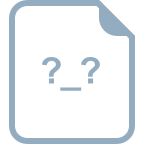
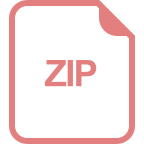
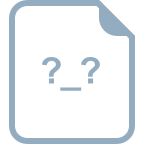
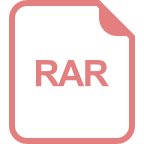