循环神经网络python实例
时间: 2023-09-15 11:21:58 浏览: 62
以下是一个简单的循环神经网络(RNN)的Python示例:
```python
import numpy as np
# 定义sigmoid函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义RNN类
class RNN:
def __init__(self, input_size, hidden_size, output_size):
# 初始化权重矩阵
self.Whh = np.random.randn(hidden_size, hidden_size)
self.Wxh = np.random.randn(hidden_size, input_size)
self.Why = np.random.randn(output_size, hidden_size)
# 初始化偏置向量
self.bh = np.zeros((hidden_size, 1))
self.by = np.zeros((output_size, 1))
def forward(self, inputs):
# 初始化隐藏状态
self.h = np.zeros((self.Whh.shape[0], 1))
# 初始化输出
self.y = np.zeros((self.Why.shape[0], inputs.shape[1]))
# 迭代每一个时间步
for t in range(inputs.shape[1]):
# 计算隐藏状态
self.h = sigmoid(np.dot(self.Whh, self.h) + np.dot(self.Wxh, inputs[:, t].reshape(-1, 1)) + self.bh)
# 计算输出
self.y[:, t] = np.dot(self.Why, self.h).flatten() + self.by.flatten()
return self.y
def backward(self, inputs, targets, learning_rate):
# 初始化梯度
dWhh = np.zeros_like(self.Whh)
dWxh = np.zeros_like(self.Wxh)
dWhy = np.zeros_like(self.Why)
dbh = np.zeros_like(self.bh)
dby = np.zeros_like(self.by)
# 初始化隐藏状态和损失
self.h = np.zeros((self.Whh.shape[0], 1))
loss = 0
# 迭代每一个时间步
for t in reversed(range(inputs.shape[1])):
# 计算输出误差
output_error = targets[:, t].reshape(-1, 1) - self.y[:, t].reshape(-1, 1)
# 更新损失
loss += np.sum(output_error ** 2)
# 计算输出梯度
dWhy += np.dot(output_error, self.h.T)
dby += output_error
# 计算隐藏状态梯度
dh = np.dot(self.Why.T, output_error) + np.dot(self.Whh, self.h) - np.dot(self.Wxh, inputs[:, t].reshape(-1, 1)) - self.bh
dh_raw = self.h * (1 - self.h)
dh = dh * dh_raw
# 更新权重和偏置梯度
dWhh += np.dot(dh, self.h.T)
dWxh += np.dot(dh, inputs[:, t].reshape(1, -1))
dbh += dh
# 更新隐藏状态
self.h = self.h - learning_rate * dh
return loss, dWhh, dWxh, dWhy, dbh, dby
# 定义训练函数
def train(inputs, targets, hidden_size, learning_rate, num_epochs):
# 初始化RNN模型
rnn = RNN(inputs.shape[0], hidden_size, targets.shape[0])
# 迭代训练
for epoch in range(num_epochs):
# 前向传播
outputs = rnn.forward(inputs)
# 反向传播
loss, dWhh, dWxh, dWhy, dbh, dby = rnn.backward(inputs, targets, learning_rate)
# 更新权重和偏置
rnn.Whh -= learning_rate * dWhh
rnn.Wxh -= learning_rate * dWxh
rnn.Why -= learning_rate * dWhy
rnn.bh -= learning_rate * dbh
rnn.by -= learning_rate * dby
# 打印损失
if (epoch+1) % 100 == 0:
print('Epoch:', epoch+1, 'Loss:', loss)
return rnn
# 测试RNN模型
inputs = np.array([[0, 1, 0, 1], [1, 0, 1, 0]])
targets = np.array([[1, 0, 1, 0], [0, 1, 0, 1]])
rnn = train(inputs, targets, hidden_size=3, learning_rate=0.1, num_epochs=1000)
outputs = rnn.forward(inputs)
print('Inputs:', inputs)
print('Outputs:', outputs)
```
这个示例实现了一个简单的RNN,用于学习两个二进制序列之间的映射关系。在训练期间,模型通过反向传播算法更新权重和偏置,以最小化输出误差。在测试期间,模型使用训练后的权重和偏置来预测新的输入序列的输出。
阅读全文
相关推荐



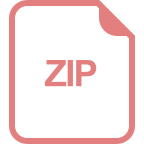
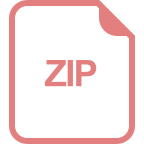
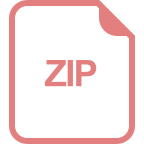
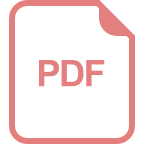
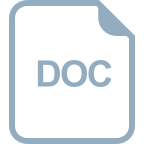
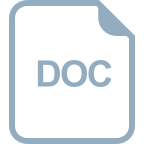
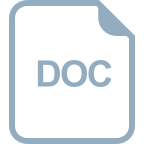

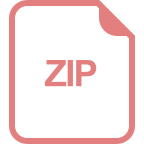
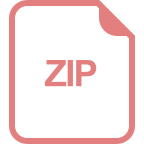
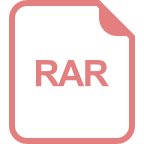
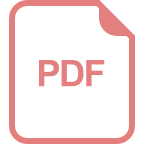
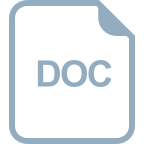