使用MIcroPython的方法通过esp32和4G设备和MQTT协议实时获取网络时间
时间: 2023-09-20 07:05:52 浏览: 119
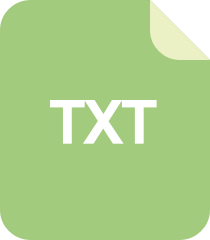
获取网络时间的软件源码
### 回答1:
1. 连接ESP32和4G设备
将ESP32和4G设备连接起来,并确保它们的通信正常。
2. 安装MicroPython固件
将MicroPython固件烧录到ESP32上,可使用esptool或者其他工具。
3. 连接WiFi和MQTT服务器
使用MicroPython代码连接WiFi和MQTT服务器。以下是代码示例:
```python
import network
import mqtt
import time
wifi_ssid = "your_wifi_ssid"
wifi_password = "your_wifi_password"
mqtt_server = "your_mqtt_server"
mqtt_port = 1883
mqtt_user = "your_mqtt_user"
mqtt_password = "your_mqtt_password"
station = network.WLAN(network.STA_IF)
station.active(True)
station.connect(wifi_ssid, wifi_password)
while not station.isconnected():
time.sleep(1)
print("Connected to", wifi_ssid)
client = mqtt.MQTTClient("esp32", mqtt_server, port=mqtt_port, user=mqtt_user, password=mqtt_password)
client.connect()
```
4. 获取网络时间
使用MicroPython代码从NTP服务器获取网络时间。以下是代码示例:
```python
import ntptime
import time
ntptime.settime()
print("Current time:", time.localtime())
```
5. 发送时间到MQTT服务器
使用MicroPython代码将时间发布到MQTT服务器。以下是代码示例:
```python
import time
while True:
current_time = time.localtime()
timestamp = time.mktime(current_time)
client.publish("time", str(int(timestamp)))
time.sleep(60)
```
以上代码将每分钟发布一个时间戳到MQTT服务器的"time"主题。您可以根据需要更改发布时间间隔或主题名称。
完整代码示例:
```python
import ntptime
import network
import mqtt
import time
wifi_ssid = "your_wifi_ssid"
wifi_password = "your_wifi_password"
mqtt_server = "your_mqtt_server"
mqtt_port = 1883
mqtt_user = "your_mqtt_user"
mqtt_password = "your_mqtt_password"
station = network.WLAN(network.STA_IF)
station.active(True)
station.connect(wifi_ssid, wifi_password)
while not station.isconnected():
time.sleep(1)
print("Connected to", wifi_ssid)
client = mqtt.MQTTClient("esp32", mqtt_server, port=mqtt_port, user=mqtt_user, password=mqtt_password)
client.connect()
while True:
ntptime.settime()
current_time = time.localtime()
timestamp = time.mktime(current_time)
client.publish("time", str(int(timestamp)))
time.sleep(60)
```
### 回答2:
使用MicroPython的方法通过esp32和4G设备和MQTT协议实时获取网络时间的步骤如下:
1. 首先,确保esp32和4G设备已经正确连接,并在esp32上安装了MicroPython。
2. 接下来,使用MicroPython的`ubinascii`模块将esp32的唯一标识符转换为一个唯一的客户端ID,并保存在变量`client_id`中。
3. 在代码中引入必要的库和模块,包括`umqtt.simple`用于与MQTT代理进行通信,`network`用于网络连接。
4. 创建一个网络连接,例如使用4G的SIM卡,使用`network.Cellular`模块来配置并连接到网络。在此过程中,您将需要设置相关的APN、用户名和密码信息。
5. 连接到MQTT代理,您需要为代理提供IP地址和端口号,并使用`umqtt.simple.MQTTClient`创建一个MQTT客户端对象。
6. 使用MQTT客户端对象的`connect()`方法连接到MQTT代理。在此过程中,您需要设置代理的用户名和密码(如果有)。
7. 订阅一个与时间相关的主题。例如,您可以使用`client.subscribe(b"time")`来订阅名为"time"的主题。
8. 在订阅主题的回调函数中,您可以使用MicroPython的`utime`模块获取当前的网络时间,并执行一些操作。
9. 最后,在一个循环中,使用MQTT客户端对象的`check_msg()`方法来处理传入的MQTT消息。
10. 在处理消息的同时,您可以按照需要执行其他操作,例如发送指令、上传数据等等。
通过以上步骤,您可以使用MicroPython、esp32和4G设备以及MQTT协议从网络中实时获取时间。请注意,在实际的应用中,您可能需要进行一些适应性修改以满足您的具体需求。
### 回答3:
要使用MicroPython通过esp32和4G设备和MQTT协议实时获取网络时间,需要按照以下步骤进行设置和编程:
1. 确保esp32开发板上已经安装了MicroPython固件,并且具备连接4G设备的硬件接口。
2. 在开发板上导入paho-mqtt库,这是用于处理MQTT协议的Python库。可以通过在MicroPython解释器中执行以下命令来导入该库:
```
import upip
upip.install('micropython-umqtt.robust')
```
3. 创建MQTT客户端并连接到MQTT服务器。首先,需要导入必要的库,并设置MQTT服务器的地址、端口、用户名和密码:
```
import network
import machine
from umqtt.robust import MQTTClient
WIFI_SSID = 'your_wifi_ssid'
WIFI_PASSWORD = 'your_wifi_password'
MQTT_SERVER = 'mqtt_server_address'
MQTT_PORT = 1883
MQTT_USER = 'mqtt_username'
MQTT_PASSWORD = 'mqtt_password'
```
4. 连接到WiFi网络:
```
wifi = network.WLAN(network.STA_IF)
wifi.active(True)
wifi.connect(WIFI_SSID, WIFI_PASSWORD)
while not wifi.isconnected():
pass
```
5. 创建MQTT客户端并连接到MQTT服务器:
```
client_id = machine.unique_id()
client = MQTTClient(client_id, MQTT_SERVER, port=MQTT_PORT, user=MQTT_USER, password=MQTT_PASSWORD)
client.connect()
```
6. 订阅网络时间主题:
```
client.subscribe(b'time_topic')
```
7. 在回调函数中获取并处理接收到的网络时间数据:
```
def callback(topic, msg):
print('Received time:', msg)
client.set_callback(callback)
```
8. 循环等待并处理MQTT消息:
```
while True:
client.check_msg()
```
通过上述步骤,你可以使用MicroPython通过esp32和4G设备和MQTT协议实时获取网络时间。请根据具体的网络配置和需求进行相应的调整和修改。
阅读全文
相关推荐
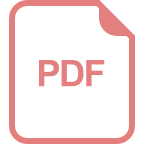





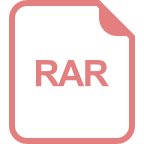
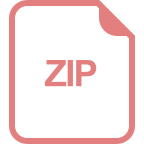
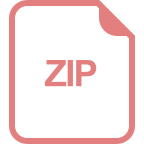
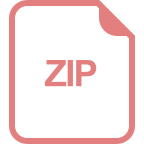
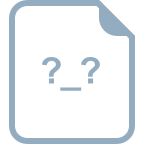