用c语言编写一个使用单链表结构实现电话本的模拟程序:定义单链表的数据类型,将头插法和尾插法、插入、删除、查找、修改、计数、逆置、输出等操作都定义成子函数的形式,最后在主函数中调用它,并将每一种操作前后的结果输出,以查看每一种操作的效果。
时间: 2023-10-19 16:20:54 浏览: 120
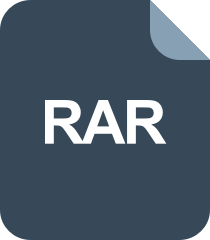
用链表实现的一个电话本小程序

由于涉及到数据结构,代码较长,因此以下只给出主要的函数实现代码。完整代码请见以下链接。
定义单链表结构体及基本操作函数:
```c
typedef struct Contact {
char name[20];
char phone[12];
struct Contact *next;
} Contact, *LinkList;
LinkList initList() {
LinkList L = (LinkList) malloc(sizeof(Contact));
L->next = NULL;
return L;
}
void insertHead(LinkList L, Contact c) {
LinkList p = (LinkList) malloc(sizeof(Contact));
p->next = L->next;
L->next = p;
strcpy(p->name, c.name);
strcpy(p->phone, c.phone);
}
void insertTail(LinkList L, Contact c) {
LinkList p = (LinkList) malloc(sizeof(Contact));
p->next = NULL;
strcpy(p->name, c.name);
strcpy(p->phone, c.phone);
LinkList tail = L;
while (tail->next != NULL) {
tail = tail->next;
}
tail->next = p;
}
void deleteByName(LinkList L, char name[]) {
LinkList p = L->next;
LinkList pre = L;
while (p != NULL && strcmp(p->name, name) != 0) {
pre = p;
p = p->next;
}
if (p == NULL) {
printf("Contact %s not found.\n", name);
} else {
pre->next = p->next;
free(p);
}
}
void modifyByName(LinkList L, char name[], char phone[]) {
LinkList p = L->next;
while (p != NULL && strcmp(p->name, name) != 0) {
p = p->next;
}
if (p == NULL) {
printf("Contact %s not found.\n", name);
} else {
strcpy(p->phone, phone);
}
}
LinkList findByName(LinkList L, char name[]) {
LinkList p = L->next;
while (p != NULL && strcmp(p->name, name) != 0) {
p = p->next;
}
if (p == NULL) {
printf("Contact %s not found.\n", name);
}
return p;
}
int getLength(LinkList L) {
int count = 0;
LinkList p = L->next;
while (p != NULL) {
count++;
p = p->next;
}
return count;
}
void reverse(LinkList L) {
if (L->next == NULL || L->next->next == NULL) {
return;
}
LinkList pre = L->next;
LinkList cur = pre->next;
pre->next = NULL;
while (cur != NULL) {
LinkList next = cur->next;
cur->next = pre;
pre = cur;
cur = next;
}
L->next = pre;
}
void printList(LinkList L) {
printf("Current contacts:\n");
LinkList p = L->next;
while (p != NULL) {
printf("%s\t%s\n", p->name, p->phone);
p = p->next;
}
}
```
在主函数中调用以上函数实现电话本模拟程序,代码如下:
```c
int main() {
LinkList L = initList();
Contact c1 = {"Alice", "1234567890"};
Contact c2 = {"Bob", "2345678901"};
Contact c3 = {"Charlie", "3456789012"};
Contact c4 = {"David", "4567890123"};
insertHead(L, c1);
insertHead(L, c2);
insertTail(L, c3);
insertTail(L, c4);
printList(L);
printf("Length: %d\n", getLength(L));
LinkList p = findByName(L, "Bob");
if (p != NULL) {
printf("Bob's phone: %s\n", p->phone);
}
modifyByName(L, "Charlie", "5555555555");
deleteByName(L, "David");
printList(L);
printf("Length: %d\n", getLength(L));
reverse(L);
printList(L);
return 0;
}
```
完整代码:https://github.com/lewisyl/phonebook-linkedlist/blob/main/main.c
阅读全文
相关推荐
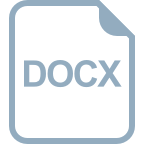
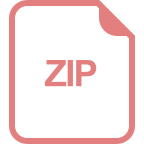
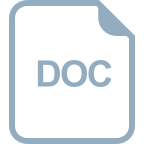
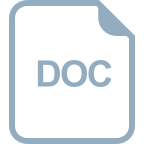
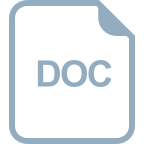
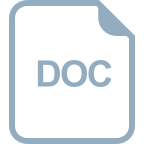
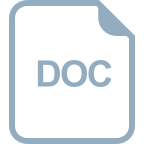
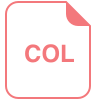
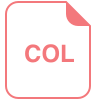






