将这个匿名内部类定义为lambda表达式:thread = new Thread(new Runnable() { @Override public void run() { //服务端要建立两个group,一个负责接收客户端的连接,一个负责处理数据传输 //连接处理group EventLoopGroup boss = new NioEventLoopGroup(); //事件处理group EventLoopGroup worker = new NioEventLoopGroup(); ServerBootstrap bootstrap = new ServerBootstrap(); // 绑定处理group bootstrap.group(boss, worker).channel(NioServerSocketChannel.class) //保持连接数 .option(ChannelOption.SO_BACKLOG, 300) //有数据立即发送 .option(ChannelOption.TCP_NODELAY, true) //保持连接 .childOption(ChannelOption.SO_KEEPALIVE, true) //处理新连接 .childHandler(new ChannelInitializer<SocketChannel>() { @Override protected void initChannel(SocketChannel sc) throws Exception { // 增加任务处理 ChannelPipeline p = sc.pipeline(); p.addLast( // //使用了netty自带的编码器和解码器 // new StringDecoder(), // new StringEncoder(), //心跳检测,读超时,写超时,读写超时 new IdleStateHandler(0, 0, 3, TimeUnit.MINUTES), //自定义的处理器 new ServerHandler()); } }); //绑定端口,同步等待成功 ChannelFuture future; try { future = bootstrap.bind(port).sync(); if (future.isSuccess()) { serverSocketChannel = (ServerSocketChannel) future.channel(); log.debug("服务端启动成功,端口:"+port); } else { log.debug("服务端启动失败!"); } //等待服务监听端口关闭,就是由于这里会将线程阻塞,导致无法发送信息,所以我这里开了线程 future.channel().closeFuture().sync(); }catch (InterruptedException e) { Thread.currentThread().interrupt(); } catch (Exception e) { log.debug(e.getMessage()); } finally { //优雅地退出,释放线程池资源 boss.shutdownGracefully(); worker.shutdownGracefully(); } } });
时间: 2024-04-22 07:28:51 浏览: 85
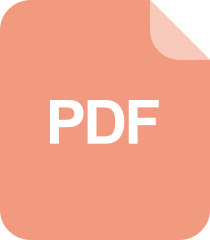
Java Lambda表达式与匿名内部类的联系和区别实例分析

thread = new Thread(() -> {
//服务端要建立两个group,一个负责接收客户端的连接,一个负责处理数据传输
//连接处理group
EventLoopGroup boss = new NioEventLoopGroup();
//事件处理group
EventLoopGroup worker = new NioEventLoopGroup();
ServerBootstrap bootstrap = new ServerBootstrap();
// 绑定处理group
bootstrap.group(boss, worker).channel(NioServerSocketChannel.class)
//保持连接数
.option(ChannelOption.SO_BACKLOG, 300)
//有数据立即发送
.option(ChannelOption.TCP_NODELAY, true)
//保持连接
.childOption(ChannelOption.SO_KEEPALIVE, true)
//处理新连接
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel sc) throws Exception {
// 增加任务处理
ChannelPipeline p = sc.pipeline();
p.addLast(
//使用了netty自带的编码器和解码器
new StringDecoder(),
new StringEncoder(),
//心跳检测,读超时,写超时,读写超时
new IdleStateHandler(0, 0, 3, TimeUnit.MINUTES),
//自定义的处理器
new ServerHandler()
);
}
});
//绑定端口,同步等待成功
ChannelFuture future;
try {
future = bootstrap.bind(port).sync();
if (future.isSuccess()) {
serverSocketChannel = (ServerSocketChannel) future.channel();
log.debug("服务端启动成功,端口:" + port);
} else {
log.debug("服务端启动失败!");
}
//等待服务监听端口关闭,就是由于这里会将线程阻塞,导致无法发送信息,所以我这里开了线程
future.channel().closeFuture().sync();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} catch (Exception e) {
log.debug(e.getMessage());
} finally {
//优雅地退出,释放线程池资源
boss.shutdownGracefully();
worker.shutdownGracefully();
}
});
阅读全文
相关推荐
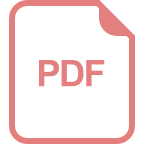
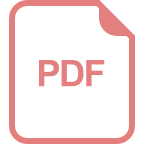


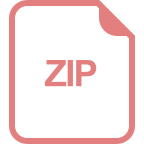
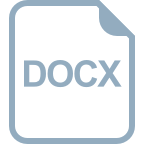
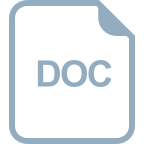
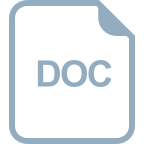
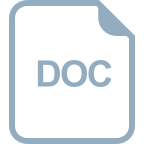
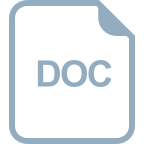
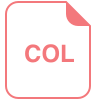
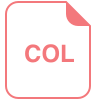
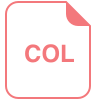





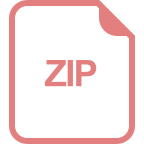