使用springboot+mysql实现积分系统
时间: 2023-09-23 18:06:27 浏览: 272
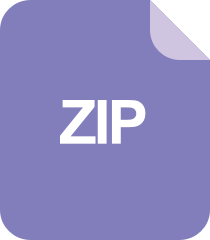
使用SpringBoot + Vue实现的DDD架构会员管理系统源码.zip

### 回答1:
好的,下面是一个简单的积分系统的实现思路:
1. 创建一个MySQL数据库,包含两个表:用户表和积分表。用户表包含用户ID、用户名等基本信息,积分表包含用户ID、积分值等字段。
2. 使用SpringBoot框架来搭建Web应用程序,包含用户登录、积分查询、积分增加、积分减少等功能。
3. 在用户登录时,根据用户名和密码验证用户身份,并将用户ID保存到Session中。
4. 在积分查询时,根据Session中的用户ID查询积分表中的积分值。
5. 在积分增加或减少时,根据Session中的用户ID更新积分表中的积分值。
下面是一个简单的代码示例:
1. 创建MySQL数据库
```sql
CREATE DATABASE IF NOT EXISTS `score_system` DEFAULT CHARSET utf8 COLLATE utf8_general_ci;
USE `score_system`;
CREATE TABLE IF NOT EXISTS `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(64) NOT NULL DEFAULT '',
`password` varchar(64) NOT NULL DEFAULT '',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
CREATE TABLE IF NOT EXISTS `score` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) NOT NULL DEFAULT '0',
`score_value` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
2. 创建SpringBoot应用程序
首先,我们需要在pom.xml文件中添加依赖:
```xml
<!-- SpringBoot -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- MySQL -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!-- MyBatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.4</version>
</dependency>
```
接着,我们需要在application.properties文件中配置MySQL数据库连接信息:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/score_system?useSSL=false&allowPublicKeyRetrieval=true&serverTimezone=Asia/Shanghai
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
mybatis.mapper-locations=classpath:mapper/*.xml
mybatis.configuration.map-underscore-to-camel-case=true
```
然后,我们需要创建User和Score两个实体类:
```java
public class User {
private int id;
private String username;
private String password;
// getters and setters
}
public class Score {
private int id;
private int userId;
private int scoreValue;
// getters and setters
}
```
接着,我们需要创建UserMapper和ScoreMapper两个Mapper接口:
```java
@Mapper
public interface UserMapper {
User selectUserByUsernameAndPassword(@Param("username") String username, @Param("password") String password);
}
@Mapper
public interface ScoreMapper {
int selectScoreByUserId(@Param("userId") int userId);
int insertScore(Score score);
int updateScoreByUserId(@Param("userId") int userId, @Param("scoreValue") int scoreValue);
}
```
最后,我们需要创建UserController和ScoreController两个Controller类,提供用户登录和积分查询、增加、减少等接口:
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserMapper userMapper;
@PostMapping("/login")
public String login(@RequestParam("username") String username, @RequestParam("password") String password, HttpSession session) {
User user = userMapper.selectUserByUsernameAndPassword(username, password);
if (user == null) {
return "login failed";
}
session.setAttribute("userId", user.getId());
return "login success";
}
}
@RestController
@RequestMapping("/score")
public class ScoreController {
@Autowired
private ScoreMapper scoreMapper;
@GetMapping("/query")
public int query(HttpSession session) {
int userId = (int) session.getAttribute("userId");
return scoreMapper.selectScoreByUserId(userId);
}
@PostMapping("/add")
public int add(@RequestParam("scoreValue") int scoreValue, HttpSession session) {
int userId = (int) session.getAttribute("userId");
Score score = new Score();
score.setUserId(userId);
score.setScoreValue(scoreValue);
scoreMapper.insertScore(score);
return scoreMapper.selectScoreByUserId(userId);
}
@PostMapping("/reduce")
public int reduce(@RequestParam("scoreValue") int scoreValue, HttpSession session) {
int userId = (int) session.getAttribute("userId");
int oldScore = scoreMapper.selectScoreByUserId(userId);
int newScore = Math.max(oldScore - scoreValue, 0);
scoreMapper.updateScoreByUserId(userId, newScore);
return newScore;
}
}
```
以上就是一个简单的积分系统的实现思路,具体的实现细节还需要根据实际需求进行调整。
### 回答2:
使用Spring Boot和MySQL实现积分系统有以下步骤:
1. 创建Spring Boot项目:可以使用Spring Initializr来创建一个基本的Spring Boot项目。
2. 配置MySQL数据库:在application.properties或application.yml文件中添加MySQL数据库的配置信息,包括url、username、password等。
3. 创建积分数据表:使用MySQL的DDL语句创建积分数据表,可以包括用户ID、积分数额等字段。
4. 创建实体类和数据访问层:使用JPA或MyBatis等技术创建与数据库中积分表对应的实体类,并提供相应的数据访问方法。
5. 创建积分服务层:创建积分服务类,处理与积分相关的业务逻辑,包括积分增加、减少、查询等操作。
6. 创建控制器:创建积分控制器,处理与积分相关的HTTP请求,包括增加积分、减少积分、查询积分等接口。
7. 配置Spring Boot启动类:在Spring Boot的启动类上添加相应的注解、扫描和配置信息。
通过上述步骤,可以完成使用Spring Boot和MySQL实现积分系统的搭建和基本功能的实现。根据具体系统需求,可以进行额外的功能拓展,例如积分记录的管理、积分兑换等功能。同时,可以利用Spring Boot的自动配置、简化开发和部署的特性,提高开发效率。
### 回答3:
使用Spring Boot和MySQL可以实现积分系统。
首先,我们需要创建一个Spring Boot项目并配置MySQL数据库连接。可以通过在application.properties文件中设置数据库相关属性来实现,如数据库连接URL、用户名和密码。
接下来,我们需要设计数据模型来存储用户信息和积分数据。可以创建两个实体类,一个是User,用于存储用户的基本信息,如用户名、密码等;另一个是Point,用于存储用户的积分信息,如用户ID、积分数等。通过配置实体类的注释和关系注解,可以实现数据库表的创建。
然后,我们需要实现相应的服务层和持久层逻辑。可以创建一个UserService和一个PointService来处理用户和积分的相关操作,如用户注册、登录和积分增减等。这些服务可以通过注入相应的Repository来实现数据库交互,并使用相应的方法来完成操作。
接着,我们可以创建相应的控制器来处理用户请求。可以创建一个UserController来处理用户注册和登录请求,并调用UserService的方法来完成相应的操作。同样地,可以创建一个PointController来处理积分相关的请求,并调用PointService的方法来进行相应的处理。
最后,我们可以编写相应的测试用例来验证系统的功能。可以使用JUnit或者SpringBootTest来编写相应的测试代码。这些测试用例可以验证用户注册和登录功能的正确性,并验证积分增减功能的准确性。
综上所述,使用Spring Boot和MySQL可以实现积分系统。
阅读全文
相关推荐
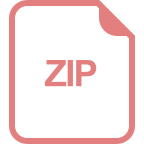
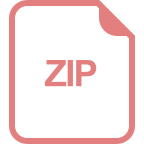











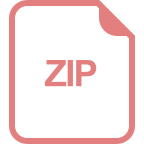
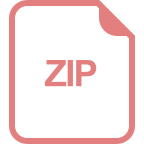
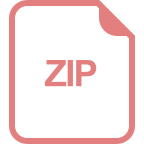
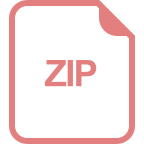
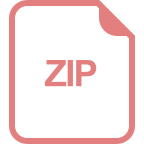