请给出一个完整的WPF示例代码,要求在WPF设计中只有一个DataGrid控件,用来让用户填写数据,CanUserAddRows="False",要求实现DataGrid表格中最后一行的所有数据都输入完毕且为非空时,触发事件自动新增一行空的模板行。
时间: 2024-01-21 12:16:32 浏览: 20
以下是一个完整的WPF示例代码,实现了在DataGrid中填写数据时自动新增一行空的模板行的功能:
XAML代码:
```xml
<Window x:Class="DataGridAutoAddRow.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<DataGrid Name="dataGrid" CanUserAddRows="False" AutoGenerateColumns="False"
ItemsSource="{Binding}">
<DataGrid.Columns>
<DataGridTextColumn Header="Name" Binding="{Binding Name}" />
<DataGridTextColumn Header="Age" Binding="{Binding Age}" />
</DataGrid.Columns>
</DataGrid>
</Grid>
</Window>
```
C#代码:
```csharp
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Windows;
namespace DataGridAutoAddRow
{
public partial class MainWindow : Window
{
private ObservableCollection<Person> persons;
public MainWindow()
{
InitializeComponent();
// 初始化数据源
persons = new ObservableCollection<Person>();
persons.Add(new Person() { Name = "", Age = "" });
dataGrid.ItemsSource = persons;
// 添加事件处理程序
dataGrid.CellEditEnding += DataGrid_CellEditEnding;
}
private void DataGrid_CellEditEnding(object sender, DataGridCellEditEndingEventArgs e)
{
// 检查最后一行的数据是否都已输入
if (e.Row.Item == persons[persons.Count - 1] &&
!string.IsNullOrEmpty(persons[persons.Count - 1].Name) &&
!string.IsNullOrEmpty(persons[persons.Count - 1].Age))
{
// 新增一行空的模板行
persons.Add(new Person() { Name = "", Age = "" });
}
}
}
public class Person : INotifyPropertyChanged
{
private string name;
private string age;
public string Name
{
get { return name; }
set
{
name = value;
OnPropertyChanged(nameof(Name));
}
}
public string Age
{
get { return age; }
set
{
age = value;
OnPropertyChanged(nameof(Age));
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
```
在这个示例中,我们首先在窗口的构造函数中初始化了DataGrid控件的数据源,将其绑定到一个ObservableCollection中。然后,我们添加了一个CellEditEnding事件处理程序,在用户编辑完最后一行的单元格时检查最后一行的数据是否都已输入,如果是,则新增一行空的模板行。注意,在Person类中我们实现了INotifyPropertyChanged接口,以便在数据发生变化时通知DataGrid控件进行更新。
相关推荐
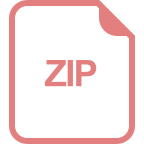
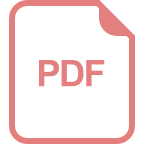














