c++编程 类的练习题以及答案
时间: 2023-06-27 14:01:00 浏览: 194
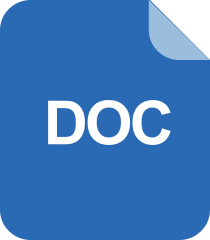
钱能\钱能c++习题答案
以下是一些C++编程练习题及其答案:
1. 编写一个程序,要求用户输入两个整数,然后输出它们的和、差、积和商。
```c++
#include<iostream>
using namespace std;
int main() {
int num1, num2;
cout << "Please enter two integers: ";
cin >> num1 >> num2;
cout << num1 << " + " << num2 << " = " << num1 + num2 << endl;
cout << num1 << " - " << num2 << " = " << num1 - num2 << endl;
cout << num1 << " * " << num2 << " = " << num1 * num2 << endl;
cout << num1 << " / " << num2 << " = " << num1 / num2 << endl;
return 0;
}
```
2. 编写一个程序,要求用户输入以兆位每秒(Mb/s)为单位的下载速度和文件大小,然后计算并输出下载该文件所需的时间。
```c++
#include<iostream>
using namespace std;
int main() {
double downloadSpeed, fileSize;
cout << "Please enter the download speed (in Mb/s): ";
cin >> downloadSpeed;
cout << "Please enter the file size (in MB): ";
cin >> fileSize;
double downloadTime = fileSize * 8 / downloadSpeed;
cout << "The download time is " << downloadTime << " seconds." << endl;
return 0;
}
```
3. 编写一个程序,要求用户输入一个整数,然后输出从1到该整数的所有整数的和。
```c++
#include<iostream>
using namespace std;
int main() {
int num, sum = 0;
cout << "Please enter an integer: ";
cin >> num;
for(int i=1; i<=num; i++) {
sum += i;
}
cout << "The sum of the integers from 1 to " << num << " is " << sum << endl;
return 0;
}
```
4. 编写一个程序,要求用户输入一个整数n,然后输出n的阶乘。
```c++
#include<iostream>
using namespace std;
int main() {
int num, factorial = 1;
cout << "Please enter an integer: ";
cin >> num;
for(int i=1; i<=num; i++) {
factorial *= i;
}
cout << num << "! = " << factorial << endl;
return 0;
}
```
5. 编写一个程序,要求用户输入一个整数n,然后输出从1到n的所有奇数的和和所有偶数的和。
```c++
#include<iostream>
using namespace std;
int main() {
int num, oddSum = 0, evenSum = 0;
cout << "Please enter an integer: ";
cin >> num;
for(int i=1; i<=num; i++) {
if(i % 2 == 0) {
evenSum += i;
} else {
oddSum += i;
}
}
cout << "The sum of odd integers from 1 to " << num << " is " << oddSum << endl;
cout << "The sum of even integers from 1 to " << num << " is " << evenSum << endl;
return 0;
}
```
阅读全文
相关推荐
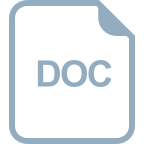
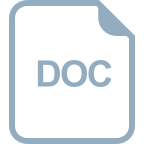
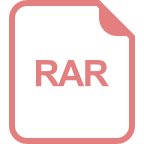
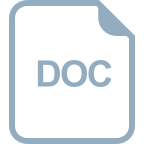
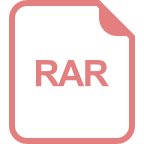
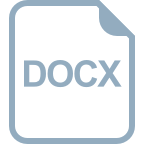
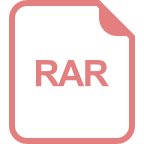
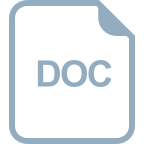
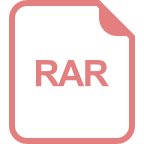
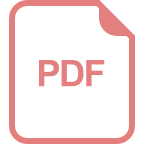
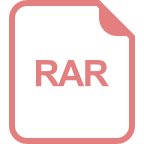
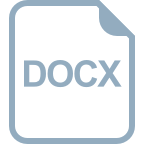
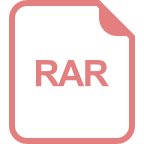
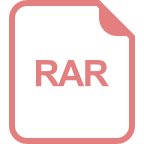
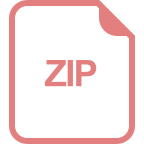
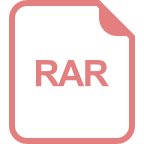