安卓Studio使用SQLite数据库制作备忘录的实验报告
时间: 2023-11-24 20:11:09 浏览: 144
实验目的:
1. 了解SQLite数据库的基本概念和使用方法
2. 掌握Android Studio中SQLite数据库的操作方法
3. 能够使用SQLite数据库存储数据并读取数据
实验步骤:
1. 新建一个Android Studio项目,并创建一个空白Activity。
2. 在项目的build.gradle文件中添加依赖项:implementation 'com.android.support:support-core-utils:28.0.0',用于支持SQLite数据库的操作。
3. 在布局文件中添加一个EditText和两个Button,分别用于输入备忘录内容、保存备忘录和读取备忘录。
4. 在Activity中定义一个SQLiteOpenHelper类,用于创建和更新数据库。
5. 在Activity中定义一个SQLiteOpenHelper对象和一个SQLiteDatabase对象,用于操作数据库。
6. 在Activity的onCreate()方法中,通过SQLiteOpenHelper对象创建或打开数据库,并创建一个备忘录表。
7. 在保存备忘录按钮的点击事件处理方法中,将EditText中的内容插入到备忘录表中。
8. 在读取备忘录按钮的点击事件处理方法中,查询备忘录表中的所有数据,并在EditText中显示。
实验代码:
```java
public class MemoActivity extends AppCompatActivity {
private EditText mMemoEditText;
private Button mSaveButton;
private Button mLoadButton;
private MemoDbHelper mDbHelper;
private SQLiteDatabase mDb;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_memo);
mMemoEditText = findViewById(R.id.memo_edit_text);
mSaveButton = findViewById(R.id.save_button);
mLoadButton = findViewById(R.id.load_button);
mDbHelper = new MemoDbHelper(this);
mDb = mDbHelper.getWritableDatabase();
mDb.execSQL(MemoContract.MemoEntry.SQL_CREATE_ENTRIES);
}
public void onSaveButtonClick(View view) {
ContentValues values = new ContentValues();
values.put(MemoContract.MemoEntry.COLUMN_NAME_CONTENT, mMemoEditText.getText().toString());
mDb.insert(MemoContract.MemoEntry.TABLE_NAME, null, values);
Toast.makeText(this, "Saved", Toast.LENGTH_SHORT).show();
}
public void onLoadButtonClick(View view) {
Cursor cursor = mDb.query(
MemoContract.MemoEntry.TABLE_NAME,
null,
null,
null,
null,
null,
null
);
StringBuilder builder = new StringBuilder();
while (cursor.moveToNext()) {
String content = cursor.getString(cursor.getColumnIndexOrThrow(MemoContract.MemoEntry.COLUMN_NAME_CONTENT));
builder.append(content);
builder.append("\n");
}
mMemoEditText.setText(builder.toString());
}
private static class MemoDbHelper extends SQLiteOpenHelper {
public static final int DATABASE_VERSION = 1;
public static final String DATABASE_NAME = "Memo.db";
public MemoDbHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(MemoContract.MemoEntry.SQL_CREATE_ENTRIES);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL(MemoContract.MemoEntry.SQL_DELETE_ENTRIES);
onCreate(db);
}
}
}
```
实验结果:
经过实验,可以成功地使用SQLite数据库存储备忘录数据,并且能够读取备忘录数据并在EditText中显示。
阅读全文
相关推荐
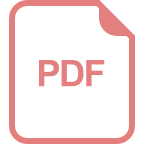
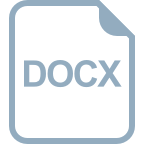
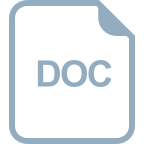















