用ssm写一个分页查询带页面显示
时间: 2023-11-12 21:03:43 浏览: 144
好的,下面是一个简单的使用SSM框架实现分页查询并带页面显示的示例。假设我们要查询一个名为"student"的表中的数据并进行分页显示,具体步骤如下:
1. 在mapper.xml文件中编写SQL语句实现分页查询功能,例如:
```
<!-- 分页查询学生信息,startIndex为起始位置,pageSize为每页显示的记录数 -->
<select id="selectStudentsByPage" parameterType="map" resultMap="studentResultMap">
SELECT * FROM student
LIMIT #{startIndex}, #{pageSize}
</select>
```
2. 在dao层接口中定义selectStudentsByPage方法,如下所示:
```
public interface StudentDao {
List<Student> selectStudentsByPage(Map<String, Object> map);
}
```
3. 在service层中编写代码调用dao层的方法,实现分页查询并返回结果,如下所示:
```
public PageInfo<Student> findStudentsByPage(int pageNum, int pageSize) {
//计算起始位置
int startIndex = (pageNum - 1) * pageSize;
//查询数据
Map<String, Object> map = new HashMap<>();
map.put("startIndex", startIndex);
map.put("pageSize", pageSize);
List<Student> list = studentDao.selectStudentsByPage(map);
//封装分页信息
PageInfo<Student> pageInfo = new PageInfo<>(list);
return pageInfo;
}
```
4. 在controller层中编写代码获取前端传来的pageNum和pageSize参数,并调用service层的方法进行分页查询,如下所示:
```
@RequestMapping("/students")
public ModelAndView findStudents(@RequestParam(defaultValue = "1") int pageNum,
@RequestParam(defaultValue = "10") int pageSize) {
PageInfo<Student> pageInfo = studentService.findStudentsByPage(pageNum, pageSize);
ModelAndView mv = new ModelAndView();
mv.addObject("pageInfo", pageInfo);
mv.setViewName("studentList");
return mv;
}
```
5. 在jsp页面中使用jstl标签库进行页面显示,如下所示:
```
<c:forEach items="${pageInfo.list}" var="student">
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
<td>${student.age}</td>
<td>${student.gender}</td>
</tr>
</c:forEach>
```
以上就是使用SSM框架实现分页查询并带页面显示的简单示例。
阅读全文
相关推荐
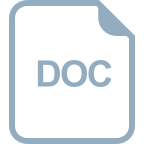
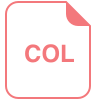

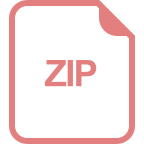
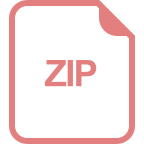
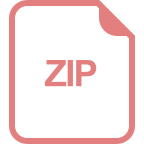
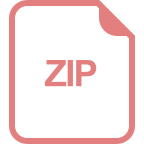
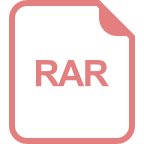
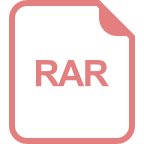
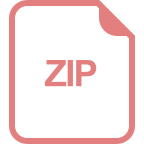
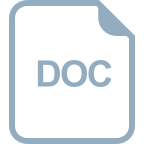




