mplayer 播放全部歌曲
时间: 2023-09-11 08:12:08 浏览: 133
你可以使用 mplayer 的循环播放功能来播放全部歌曲,具体操作如下:
1. 打开终端,进入存放歌曲的文件夹。
2. 执行以下命令:
```
mplayer -loop 0 *.mp3
```
其中 `-loop 0` 表示循环播放,`*.mp3` 表示播放所有以 `.mp3` 结尾的文件。
3. 按下 Enter 键开始播放。
这样就可以播放该文件夹下所有的 MP3 文件,循环播放直到手动停止。
相关问题
使用popen()函数实现对mplayer播放歌曲的歌曲时长获取和当前歌曲进度获取
可以使用 `popen()` 函数打开一个进程并与其进行通信。对于 `mplayer` 播放器,可以使用 `-identify` 参数获取歌曲时长和其他信息,使用 `-slave` 参数启用控制模式,然后通过管道向 `mplayer` 发送命令获取当前歌曲进度。
以下是一个示例程序,该程序通过 `popen()` 打开 `mplayer` 进程并获取歌曲时长和当前进度。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#define BUF_SIZE 512
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: %s <filename>\n", argv[0]);
return -1;
}
char cmd[BUF_SIZE];
sprintf(cmd, "mplayer -identify -slave %s 2>&1", argv[1]);
FILE *fp = popen(cmd, "r");
if (fp == NULL) {
printf("Failed to open pipe\n");
return -1;
}
int duration = 0;
int position = 0;
while (1) {
char buf[BUF_SIZE];
if (fgets(buf, BUF_SIZE, fp) == NULL) {
break;
}
// 获取歌曲总时长
char *p = strstr(buf, "ID_LENGTH=");
if (p != NULL) {
duration = atoi(p + 10);
}
// 获取当前播放进度
p = strstr(buf, "ANS_TIME_POSITION=");
if (p != NULL) {
position = atoi(p + 17);
}
printf("%s", buf);
fflush(stdout);
}
pclose(fp);
printf("Duration: %d seconds\n", duration);
printf("Position: %d seconds\n", position);
return 0;
}
```
在命令行中运行该程序,例如:
```shell
./get_song_info /path/to/song.mp3
```
该程序将打印 `mplayer` 的输出并输出歌曲总时长和当前进度。
、 编写程序,在程序中创建一个子进程,使子进程通过exec更改代码段,执行mplayer命令来播放歌曲;并且在父进程中同步显式歌曲对应的歌词。
在C语言中,可以使用fork()函数创建一个子进程,然后使用exec()函数来更改代码段并执行mplayer命令。在父进程中,可以使用wait()函数来等待子进程执行完毕,然后同步显式歌曲对应的歌词。
下面是一个示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main()
{
pid_t pid;
int status;
pid = fork();
if (pid == -1) {
printf("Failed to create child process\n"); exit(1);
} else if (pid == 0) {
// Child process
execl("/usr/bin/mplayer", "mplayer", "song.mp3", NULL);
printf("Failed to execute mplayer\n");
exit(1);
} else {
// Parent process
wait(&status);
printf("Song finished playing\n");
printf("Lyrics: ...\n");
}
return 0;
}
```
在上面的代码中,子进程使用execl()函数来执行mplayer命令,并播放名为"song.mp3"的歌曲。在父进程中,使用wait()函数等待子进程执行完毕,并在歌曲播放完毕后同步显式歌曲对应的歌词。
阅读全文
相关推荐








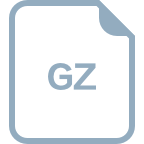




