用python写什么代码可以下载一首歌曲
时间: 2024-02-01 08:01:19 浏览: 12
要用Python写一个可以下载一首歌曲的代码,可以使用Python的requests库来实现。以下是一个简单的实现:
```python
import requests
def download_song(url, saving_path):
response = requests.get(url)
if response.status_code == 200:
with open(saving_path, 'wb') as file:
file.write(response.content)
print("歌曲下载成功!")
else:
print("无法下载歌曲。")
# Example usage
url = "https://example.com/song.mp3" # 歌曲的URL链接
saving_path = "path/to/save/song.mp3" # 歌曲保存的路径
download_song(url, saving_path)
```
在这个例子中,我们定义了一个名为`download_song`的函数,它接收歌曲的URL链接和保存路径作为参数。函数使用requests库发送GET请求来下载歌曲。如果响应状态码为200,表示请求成功,我们把响应的内容写入指定的保存路径,从而完成歌曲的下载。
你只需要将`url`和`saving_path`参数替换为实际的URL链接和保存路径,就能成功下载歌曲。请确保你有合法的许可来下载该歌曲。
相关问题
python写一个可以爬取音乐的代码
### 回答1:
这是一段爬取音乐的简单 Python 代码:
```python
import requests
from bs4 import BeautifulSoup
# 请求网页
url = "https://music.163.com/playlist?id=3778678"
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
res = requests.get(url, headers=headers)
# 解析网页
soup = BeautifulSoup(res.text, 'html.parser')
# 查找音乐信息
music_list = soup.select('.f-hide a')
for music in music_list:
print(music.getText())
```
此代码爬取的是网易云音乐中某个歌单的信息,使用了requests库和beautifulsoup库,需要在终端中运行。
请注意使用爬虫爬取网站的数据时需要遵循网站的相关协议和规定。
### 回答2:
以下是一个使用Python编写的爬取音乐的代码示例:
```python
import requests
from bs4 import BeautifulSoup
def get_music_list(keyword):
url = f"https://www.example.com/search?keywords={keyword}"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
music_list = []
results = soup.find_all('div', {'class': 'music-item'})
for result in results:
title = result.find('h2', {'class': 'title'}).text.strip()
artist = result.find('p', {'class': 'artist'}).text.strip()
music_list.append({'title': title, 'artist': artist})
return music_list
def download_music(url, file_path):
response = requests.get(url)
with open(file_path, 'wb') as file:
file.write(response.content)
if __name__ == "__main__":
keyword = input("请输入要搜索的音乐关键字:")
music_list = get_music_list(keyword)
print(f"共找到{len(music_list)}首符合条件的音乐:")
for index, music in enumerate(music_list):
print(f"{index + 1}. 标题:{music['title']},艺术家:{music['artist']}")
# 假设下载链接在music字典的'download_url'键中保存
download_url = music['download_url']
file_path = f"music{index + 1}.mp3"
download_music(download_url, file_path)
print(f"音乐已下载保存为:{file_path}")
```
这段代码通过向音乐搜索网站发送GET请求,获取搜索结果页面的HTML内容,并使用BeautifulSoup解析HTML,提取出音乐的标题和艺术家信息。然后,根据用户输入的关键字,获取音乐列表。接下来,代码迭代音乐列表,并下载每首歌曲的音乐文件到本地。在代码中,假设下载链接保存在音乐字典的`download_url`键中,下载的音乐文件将保存为`music1.mp3`、`music2.mp3`等。
### 回答3:
import requests
from bs4 import BeautifulSoup
# 定义爬取音乐的函数
def crawl_music(keyword):
url = f"https://music.example.com/search?keyword={keyword}" # 替换为实际的音乐网站链接
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) "
"Chrome/58.0.3029.110 Safari/537.3"
} # 添加User-Agent以伪装浏览器请求
response = requests.get(url, headers=headers) # 发送GET请求
soup = BeautifulSoup(response.text, "html.parser") # 解析网页
music_list = soup.find_all("a", class_="music") # 根据实际网页结构找到音乐链接标签
for music in music_list:
music_name = music.text # 获取音乐名称
music_url = music["href"] # 获取音乐链接
print("音乐名称:" + music_name)
print("音乐链接:" + music_url)
print("--------")
# 调用函数进行音乐爬取
crawl_music("周杰伦") # 以"周杰伦"为关键词爬取音乐链接
写一个python爬虫爬取QQ音乐歌曲的代码
好的,以下是一个简单的Python爬虫爬取QQ音乐歌曲的代码:
```python
import requests
import json
def get_song_list(keyword, page):
"""
获取歌曲列表
:param keyword: 搜索关键词
:param page: 页码
:return: 歌曲列表
"""
url = 'https://c.y.qq.com/soso/fcgi-bin/client_search_cp'
headers = {
'referer': 'https://y.qq.com/portal/search.html',
'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
params = {
'ct': '24',
'qqmusic_ver': '1298',
'new_json': '1',
'remoteplace': 'sizer.yqq.song_next',
'searchid': '64405487069162918',
't': '0',
'aggr': '1',
'cr': '1',
'catZhida': '1',
'lossless': '0',
'flag_qc': '0',
'p': page,
'n': '20',
'w': keyword,
'g_tk': '5381',
'loginUin': '0',
'hostUin': '0',
'format': 'json',
'inCharset': 'utf8',
'outCharset': 'utf-8',
'notice': '0',
'platform': 'yqq.json',
'needNewCode': '0'
}
response = requests.get(url, headers=headers, params=params)
if response.status_code == 200:
song_list = []
data = json.loads(response.text)
for song in data['data']['song']['list']:
song_info = {
'song_name': song['name'],
'singer': song['singer'][0]['name'],
'album': song['album']['name'],
'interval': song['interval'],
'song_id': song['mid']
}
song_list.append(song_info)
return song_list
else:
return None
def get_song_url(song_id):
"""
获取歌曲播放链接
:param song_id: 歌曲ID
:return: 歌曲播放链接
"""
url = 'https://u.y.qq.com/cgi-bin/musicu.fcg'
headers = {
'referer': 'https://y.qq.com/',
'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
params = {
'-': 'getplaysongvkey' + str(int(round(time.time() * 1000))),
'g_tk': '5381',
'loginUin': '0',
'hostUin': '0',
'format': 'json',
'inCharset': 'utf8',
'outCharset': 'utf-8',
'notice': '0',
'platform': 'yqq.json',
'needNewCode': '0',
'data': '{"req":{"module":"CDN.SrfCdnDispatchServer","method":"GetCdnDispatch","param":{"guid":"1535846080","calltype":0,"userip":""}},"req_0":{"module":"vkey.GetVkeyServer","method":"CgiGetVkey","param":{"guid":"1535846080","songmid":["' + song_id + '"],"songtype":[0],"uin":"0","loginflag":1,"platform":"20"}},"comm":{"uin":0,"format":"json","ct":24,"cv":0}}'
}
response = requests.get(url, headers=headers, params=params)
if response.status_code == 200:
data = json.loads(response.text)
if data['code'] == 0:
return data['req_0']['data']['midurlinfo'][0]['purl']
else:
return None
else:
return None
if __name__ == '__main__':
keyword = '周杰伦'
page = 1
song_list = get_song_list(keyword, page)
for song in song_list:
song_url = get_song_url(song['song_id'])
print(song['song_name'], song['singer'], song['album'], song_url)
```
通过以上代码,我们可以获取到指定关键词下的歌曲列表,并且获取每首歌曲的播放链接。
相关推荐
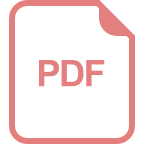
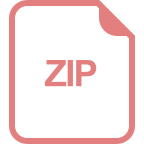
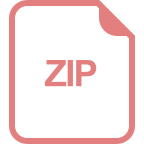












