编写一个矩阵类Matrix,实现矩阵加法,减法,乘法和数乘功能。 本题中数乘用于将矩阵中的每个元素扩大整除倍。 输入: 输入为三个矩阵 每个矩阵输入的第一行为M,N两个整数,表示矩阵的行数和列数 ; 接下来M行为矩阵的内容 。 输出: 矩阵求和、差值和乘积
时间: 2024-12-05 20:09:05 浏览: 28
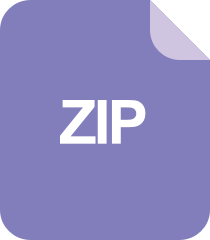
EigenDemon_matrix_矩阵运算库_线性代数_数学函数库_eigen_

为了实现这个Matrix类,我们可以首先定义一个内部数据结构来存储矩阵,然后分别添加加法、减法、乘法和数乘的方法。这里是一个简单的Python实现示例:
```python
class Matrix:
def __init__(self, rows, cols):
self.rows = rows
self.cols = cols
self.data = []
def input_matrix(self, matrix_string):
self.data = [[int(num) for num in row.split()] for row in matrix_string.strip().split("\n")]
def add_matrix(self, other):
if self.rows != other.rows or self.cols != other.cols:
raise ValueError("Matrices must have the same dimensions.")
result = Matrix(self.rows, self.cols)
result.data = [list(map(sum, zip(a, b))) for a, b in zip(self.data, other.data)]
return result
def subtract_matrix(self, other):
if self.rows != other.rows or self.cols != other.cols:
raise ValueError("Matrices must have the same dimensions.")
result = Matrix(self.rows, self.cols)
result.data = [list(map(lambda x, y: x - y, a, b)) for a, b in zip(self.data, other.data)]
return result
def multiply_matrix(self, other):
if self.cols != other.rows:
raise ValueError("The number of columns in the first matrix must be equal to the number of rows in the second matrix.")
result = Matrix(other.rows, self.cols)
result.data = [[sum(row * col for row in other.data[i]) for col in self.data[j]] for i, j in enumerate(result.data)]
return result
def scalar_multiply(self, scalar):
new_data = [[cell * scalar for cell in row] for row in self.data]
return Matrix(self.rows, self.cols, new_data)
# 示例:
matrix_a = Matrix(2, 2).input_matrix("1 2\n3 4")
matrix_b = Matrix(2, 2).input_matrix("5 6\n7 8")
matrix_sum = matrix_a.add_matrix(matrix_b)
matrix_diff = matrix_a.subtract_matrix(matrix_b)
matrix_prod = matrix_a.multiply_matrix(matrix_b)
scaled_matrix = matrix_a.scalar_multiply(2)
print("Sum:\n", matrix_sum.data)
print("Difference:\n", matrix_diff.data)
print("Product:\n", matrix_prod.data)
print("Scalar Multiply (2x):\n", scaled_matrix.data)
```
阅读全文
相关推荐
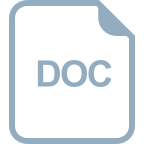
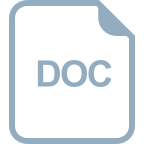
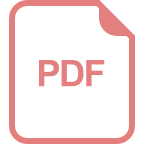
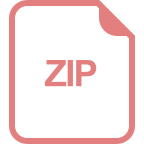
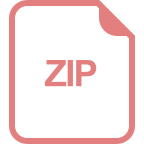
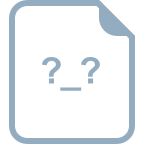
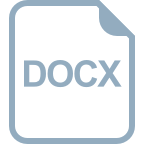
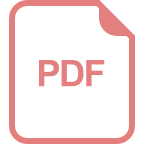
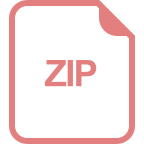
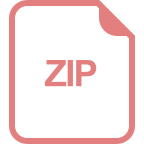
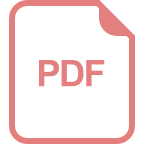
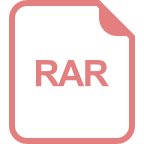
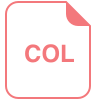
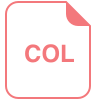
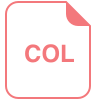
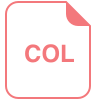
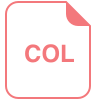
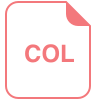