用c++写出运用类封装,继承,多态等技术的一个简易的扫雷小游戏的项目开发
时间: 2024-09-09 19:14:48 浏览: 68
设计一个简单的扫雷游戏项目涉及到C++的几个核心特性,包括类封装、继承和多态。以下是简化的步骤和代码示例:
1. **类封装**:
首先创建一个基础的`Cell`类,表示棋盘上的格子,包含状态(是否埋雷、已翻开还是未知)、周围有多少颗雷等属性和初始化方法。
```cpp
class Cell {
private:
bool isMine;
int numAdjacentMines;
public:
Cell(int mineCount);
// getter and setter methods
};
```
2. **继承**:
创建一个`MineCell`类,继承自`Cell`,添加额外处理挖掘雷的方法。
```cpp
class MineCell : public Cell {
public:
MineCell(int mineCount) : Cell(mineCount) {}
virtual void explode();
};
```
3. **多态**:
实现`explode()`方法,在基类中声明虚函数,然后在派生类中提供具体的实现。
```cpp
// Base class definition
virtual void Cell::explode() {
// Default behavior
}
// Derived class implementation
void MineCell::explode() {
// Handle exploding logic for a mine cell
}
```
4. **游戏主循环**:
创建一个`GameBoard`类,包含一个动态数组来存储`Cell`对象,通过指针或引用操作实现多态。
```cpp
class GameBoard {
private:
std::vector<std::unique_ptr<Cell>> board;
public:
void init(int width, int height);
void reveal(int x, int y); // 通用揭示逻辑,调用对应cell的explode方法
};
```
5. **用户交互和游戏控制**:
主程序中提供用户输入,更新`GameBoard`的状态并触发相应的行为。
```cpp
int main() {
GameBoard game(10, 10); // 初始化一个10x10的游戏板
// Main game loop...
return 0;
}
```
阅读全文
相关推荐
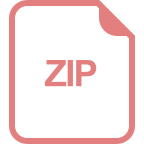
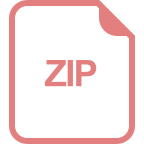
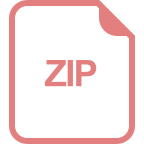

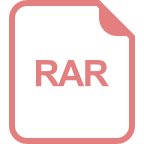
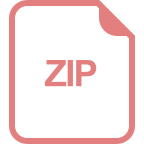
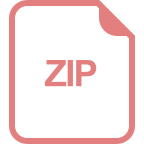
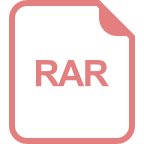
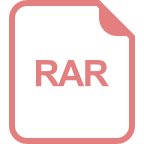
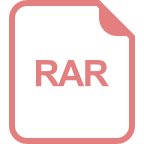
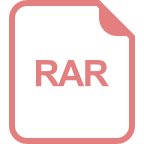
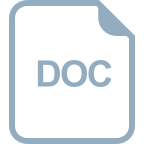
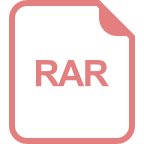
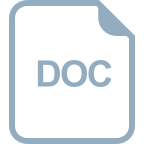
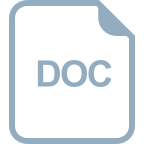
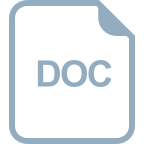
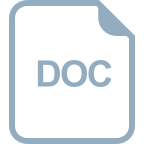
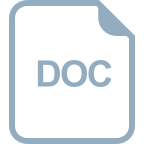
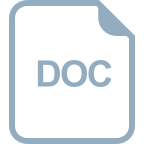