c++用户注册和登录功能
时间: 2024-12-31 13:42:47 浏览: 7
### 实现C++程序中的用户注册和登录功能
#### 创建必要的头文件与类定义
为了实现用户注册和登录的功能,首先需要设计`User`类以及管理这些操作的服务类。这里假设有一个名为`UserService`的类负责处理所有的认证逻辑。
```cpp
// user.h
#ifndef USER_H_
#define USER_H_
#include <string>
using namespace std;
class User {
public:
string username;
string password; // In practice, store hashed passwords instead of plain text.
User(const string& name, const string& pwd);
};
#endif /* USER_H_ */
```
对于实际应用而言,在保存密码时应当采用哈希算法而不是明文存储[^2]。
#### 设计服务层接口
接下来定义一个服务类来封装业务逻辑:
```cpp
// userService.h
#ifndef USERSERVICE_H_
#define USERSERVICE_H_
#include "user.h"
#include <unordered_map>
class UserService {
private:
unordered_map<string, User> usersDatabase_; // Simple in-memory storage.
public:
bool registerUser(const string&, const string&);
bool loginUser(const string&, const string&);
void clear(); // For testing purposes only.
};
#endif /* USERSERVICE_H_ */
```
此部分展示了基本的数据结构和服务方法声明。注意这里的数据库模拟使用了一个简单的内存映射表;在真实环境中应该替换为持久化解决方案如SQL或NoSQL数据库[^3]。
#### 编写具体实现
以下是上述两个类的具体实现细节:
```cpp
// user.cpp
#include "user.h"
User::User(const string& name, const string& pwd):username(name),password(pwd){}
// userService.cpp
#include "userService.h"
bool UserService::registerUser(const string& userName, const string& passWord){
auto result = this->usersDatabase_.emplace(userName, User(userName,passWord));
return result.second;
}
bool UserService::loginUser(const string& userName, const string& passWord){
auto it = this->usersDatabase_.find(userName);
if(it != end(this->usersDatabase_) && it->second.password == passWord){
return true;
}
return false;
}
void UserService::clear(){
this->usersDatabase_.clear();
}
```
这段代码实现了用户注册(`registerUser`) 和 登录 (`loginUser`) 的核心逻辑。当尝试注册新账户时会检查是否存在相同名称的记录;而登录则匹配已有的用户名及其对应的密码[^1]。
#### 主函数入口
最后编写测试用例以展示整个流程的工作方式:
```cpp
// main.cpp
#include <iostream>
#include "userService.h"
int main() {
UserService service;
string username, password;
cout << "请输入要注册的用户名:";
cin >> username;
cout << "设置您的密码:";
cin >> password;
if(service.registerUser(username,password)){
cout << "注册成功!"<< endl;
cout << "\n现在请尝试登陆:\n";
cout << "输入您刚才设定的用户名:";
cin >> username;
cout << "输入您刚才设定的密码:";
cin >> password;
if (service.loginUser(username, password)) {
cout << "欢迎回来," << username << "! 登陆成功." << endl;
} else {
cout << "很遗憾, 登陆失败. 请确认您的凭证并重试." << endl;
}
}else{
cout << "该用户名已被占用.";
}
return 0;
}
```
以上就是完整的基于命令行界面(CLI)下的简易版用户注册/登录系统的实现方案。
阅读全文
相关推荐








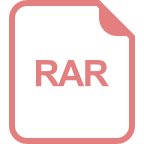









