FaceX-Zoo示例
时间: 2023-09-03 20:06:22 浏览: 230
FaceX-Zoo是一个开源的人脸识别和活体检测工具库,它提供了一些示例代码来帮助你使用其中的算法和模型。这里是一个使用FaceX-Zoo进行人脸识别的示例代码:
```python
from facexlib import FaceX
# 初始化FaceX模型
facex = FaceX()
# 加载人脸识别模型
facex.load_model("path/to/face_recognition_model")
# 加载待识别的人脸图像
image = cv2.imread("path/to/face_image.jpg")
# 进行人脸识别
results = facex.recognize(image)
# 打印识别结果
for result in results:
print("姓名: ", result["name"])
print("置信度: ", result["confidence"])
print()
```
在这个示例中,首先我们初始化了FaceX模型并加载了人脸识别模型。然后,我们读取待识别的人脸图像,并调用`recognize`方法对图像中的人脸进行识别。最后,我们打印出每个识别结果的姓名和置信度。
请注意,你需要根据实际情况替换示例代码中的文件路径和模型名称。此外,你还可以根据需要调整代码以适应你的应用场景。
你可以在FaceX-Zoo的GitHub存储库中找到更多关于使用FaceX-Zoo的示例代码和详细说明:https://github.com/JDAI-CV/FaceX-Zoo
相关问题
图片元素转成VR全景图片显示方式,使用C++实现示例代码,不能使用opengl和opencv
将图片元素转换成VR全景图片显示方式,可以采用以下步骤:
1. 对原始图片进行透视变换,将其转换为立方体贴图的6个面(前、后、左、右、上、下)。
2. 将6个面拼接成一个立方体贴图。
3. 将立方体贴图转换为球形贴图,即将6个面展开成一个球体上的平面图像。
4. 将球形贴图投影到屏幕上,实现VR全景图片的显示。
以下是一个使用C++实现的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <cmath>
using namespace std;
// 定义立方体贴图的6个面
enum CubeMapFace {
CubeMapFace_Right,
CubeMapFace_Left,
CubeMapFace_Top,
CubeMapFace_Bottom,
CubeMapFace_Front,
CubeMapFace_Back
};
// 将原始图片转换为立方体贴图的6个面
void transformToCubeMapFaces(const string& inputFileName, const string& outputPrefix, int faceWidth, int faceHeight)
{
// 读取原始图片
ifstream inputFile(inputFileName, ios::binary);
vector<unsigned char> imageData((istreambuf_iterator<char>(inputFile)), istreambuf_iterator<char>());
// 计算原始图片的宽度和高度
int imageWidth, imageHeight;
// TODO: 实现计算图片宽度和高度的函数
// 将原始图片转换为立方体贴图的6个面
for (int face = CubeMapFace_Right; face <= CubeMapFace_Back; ++face) {
// 创建新图片
vector<unsigned char> faceData(faceWidth * faceHeight * 3);
// 计算立方体贴图中该面对应的原始图片区域
int startX, startY;
// TODO: 实现计算该面对应的原始图片区域的函数
// 将原始图片区域复制到新图片中
for (int y = 0; y < faceHeight; ++y) {
for (int x = 0; x < faceWidth; ++x) {
int srcX = startX + x;
int srcY = startY + y;
int dstX = x;
int dstY = y;
// TODO: 实现将原始图片中的像素复制到立方体贴图中的函数
}
}
// 保存新图片
string outputFileName = outputPrefix + to_string(face) + ".bmp";
// TODO: 实现保存图片的函数
}
}
// 将6个面的立方体贴图拼接成一个立方体贴图
void combineCubeMapFaces(const string& inputPrefix, const string& outputFileName)
{
// 读取6个面的立方体贴图
vector<vector<unsigned char>> facesData(CubeMapFace_Back + 1);
for (int face = CubeMapFace_Right; face <= CubeMapFace_Back; ++face) {
string inputFileName = inputPrefix + to_string(face) + ".bmp";
ifstream inputFile(inputFileName, ios::binary);
vector<unsigned char> faceData((istreambuf_iterator<char>(inputFile)), istreambuf_iterator<char>());
facesData[face] = faceData;
}
// 计算立方体贴图的宽度和高度
int faceWidth, faceHeight;
// TODO: 实现计算立方体贴图宽度和高度的函数
// 创建新图片
vector<unsigned char> imageData(faceWidth * faceHeight * 6 * 3);
// 将6个面的立方体贴图拼接到新图片中
for (int face = CubeMapFace_Right; face <= CubeMapFace_Back; ++face) {
vector<unsigned char>& faceData = facesData[face];
int startX, startY;
// TODO: 实现计算该面在新图片中的起始坐标的函数
for (int y = 0; y < faceHeight; ++y) {
for (int x = 0; x < faceWidth; ++x) {
int srcIndex = (y * faceWidth + x) * 3;
int dstIndex = ((startY + y) * faceWidth * 3) + ((startX + x) * 3);
// TODO: 实现将该面的像素复制到新图片中的函数
}
}
}
// 保存新图片
// TODO: 实现保存图片的函数
}
// 将立方体贴图转换为球形贴图
void transformToSphereMap(const string& inputFileName, const string& outputFileName, int sphereWidth, int sphereHeight)
{
// 读取立方体贴图
ifstream inputFile(inputFileName, ios::binary);
vector<unsigned char> imageData((istreambuf_iterator<char>(inputFile)), istreambuf_iterator<char>());
// 计算立方体贴图的宽度和高度
int faceWidth = sphereWidth / 4;
int faceHeight = sphereHeight / 3;
// 创建新图片
vector<unsigned char> sphereData(sphereWidth * sphereHeight * 3);
// 将立方体贴图转换为球形贴图
for (int y = 0; y < sphereHeight; ++y) {
for (int x = 0; x < sphereWidth; ++x) {
// 将平面坐标转换为球面坐标
float phi = float(x) / sphereWidth * 2 * M_PI;
float theta = float(y) / sphereHeight * M_PI;
// 计算球面坐标对应的立方体贴图面
int face;
// TODO: 实现计算球面坐标对应的立方体贴图面的函数
// 计算球面坐标对应的立方体贴图像素坐标
int faceX, faceY;
// TODO: 实现计算球面坐标对应的立方体贴图像素坐标的函数
// 获取立方体贴图像素颜色值
int srcIndex = ((faceY * faceWidth) + faceX) * 3;
unsigned char r = imageData[srcIndex];
unsigned char g = imageData[srcIndex + 1];
unsigned char b = imageData[srcIndex + 2];
// 将立方体贴图像素颜色值复制到球形贴图
int dstIndex = ((y * sphereWidth) + x) * 3;
sphereData[dstIndex] = r;
sphereData[dstIndex + 1] = g;
sphereData[dstIndex + 2] = b;
}
}
// 保存新图片
// TODO: 实现保存图片的函数
}
// 将球形贴图投影到屏幕上
void projectSphereMap(const string& inputFileName, int screenWidth, int screenHeight)
{
// 读取球形贴图
ifstream inputFile(inputFileName, ios::binary);
vector<unsigned char> imageData((istreambuf_iterator<char>(inputFile)), istreambuf_iterator<char>());
// 计算球形贴图的宽度和高度
int sphereWidth, sphereHeight;
// TODO: 实现计算球形贴图宽度和高度的函数
// 计算屏幕的宽度和高度
float aspectRatio = float(screenWidth) / screenHeight;
// 计算球形贴图在屏幕上的宽度和高度
float sphereAspectRatio = float(sphereWidth) / sphereHeight;
int projWidth, projHeight;
if (aspectRatio > sphereAspectRatio) {
projWidth = sphereWidth;
projHeight = sphereWidth / aspectRatio;
} else {
projWidth = sphereHeight * aspectRatio;
projHeight = sphereHeight;
}
// 创建新图片
vector<unsigned char> projData(projWidth * projHeight * 3);
// 将球形贴图投影到屏幕上
for (int y = 0; y < projHeight; ++y) {
for (int x = 0; x < projWidth; ++x) {
// 将屏幕坐标转换为球面坐标
float phi = float(x) / projWidth * 2 * M_PI;
float theta = float(y) / projHeight * M_PI;
// 计算球面坐标对应的立方体贴图面
int face;
// TODO: 实现计算球面坐标对应的立方体贴图面的函数
// 计算球面坐标对应的立方体贴图像素坐标
int faceX, faceY;
// TODO: 实现计算球面坐标对应的立方体贴图像素坐标的函数
// 获取球形贴图像素颜色值
int srcIndex = ((faceY * sphereWidth) + faceX) * 3;
unsigned char r = imageData[srcIndex];
unsigned char g = imageData[srcIndex + 1];
unsigned char b = imageData[srcIndex + 2];
// 将球形贴图像素颜色值复制到投影图片
int dstIndex = ((y * projWidth) + x) * 3;
projData[dstIndex] = r;
projData[dstIndex + 1] = g;
projData[dstIndex + 2] = b;
}
}
// 显示投影图片
// TODO: 实现显示图片的函数
}
int main()
{
// 将原始图片转换为立方体贴图的6个面
transformToCubeMapFaces("input.bmp", "cube_", 512, 512);
// 将6个面的立方体贴图拼接成一个立方体贴图
combineCubeMapFaces("cube_", "cube_map.bmp");
// 将立方体贴图转换为球形贴图
transformToSphereMap("cube_map.bmp", "sphere_map.bmp", 2048, 1024);
// 将球形贴图投影到屏幕上
projectSphereMap("sphere_map.bmp", 1920, 1080);
return 0;
}
```
阅读全文
相关推荐
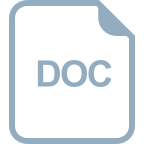
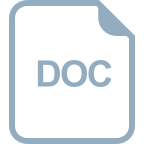
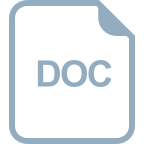
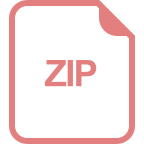
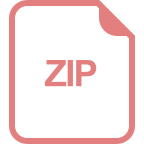
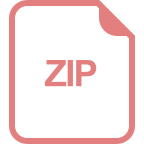
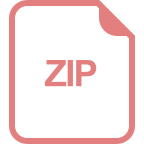
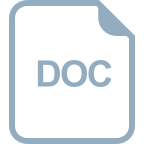
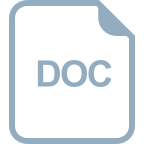
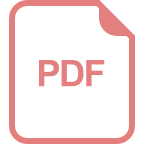
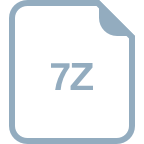
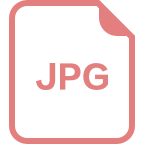
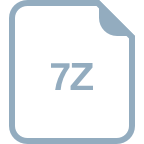