C++ 灰度图bmp存入二维数组
时间: 2025-01-07 09:04:18 浏览: 9
### 将灰度图 BMP 文件读取到 C++ 中的二维数组
为了实现这一目标,可以利用 OpenCV 库来简化图像处理流程。以下是具体方法:
#### 使用 OpenCV 读取图像并转换为二维数组
OpenCV 提供了一个强大的接口用于图像操作。通过 `imread` 函数可以从磁盘加载图像,并将其作为矩阵对象返回。对于灰度图像而言,该函数会自动将彩色通道压缩成单个亮度值。
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
// 定义全局变量表示图像的高度和宽度
const int Height = 480;
const int Width = 640;
int main() {
// 加载灰度模式下的测试图片
Mat image_gray = imread("./test.bmp", IMREAD_GRAYSCALE);
if (image_gray.empty()) {
printf("Could not open or find the image\n");
return -1;
}
// 创建一个unsigned char类型的二维数组用来保存灰度值
unsigned char img_gray[Height][Width];
// 自定义函数Mat2array完成从cv::Mat至二维数组之间的转换
void Mat2array(const Mat& mat, unsigned char array[][Width], int height, int width);
// 调用自定义函数进行实际的数据复制工作
Mat2array(image_gray, img_gray, Height, Width);
// 显示原始图像以便验证正确性
imshow("Gray Image", image_gray);
waitKey(0);
return 0;
}
```
上述代码展示了如何使用 OpenCV 的 `imread()` 方法读入一幅灰度图像,并创建了一个名为 `img_gray` 的二维字符型数组来容纳这些像素数据[^2]。
接下来是一个辅助性的成员函数原型声明及其简单的逻辑框架,它负责执行由 `cv::Mat` 至标准 C/C++ 数组间的映射关系建立:
```cpp
void Mat2array(const Mat& mat, unsigned char array[][Width], int height, int width){
for(int i=0;i<height;++i){
for(int j=0;j<width;++j){
array[i][j]=mat.at<unsigned char>(i,j);
}
}
}
```
这段代码实现了遍历整个输入矩阵并将每个元素赋给对应的二维数组位置的操作。
阅读全文
相关推荐




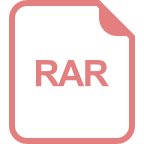



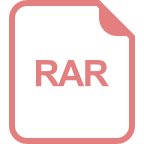






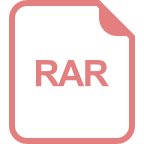
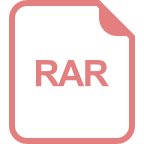
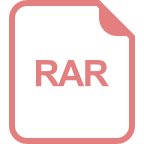
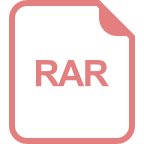