在Aside添加预约挂号按钮,点击后跳转AppointForm界面,并将其中的预约挂号改到头顶居中。提交预约挂号后跳转至医生列表选择医生
时间: 2024-12-13 21:24:00 浏览: 7
为了实现您的需求,您需要对现有代码进行以下修改:
### 1. 修改 Aside 部分
在 `Src/App.vue` 文件中的 `<el-aside>` 标签内添加一个新的菜单项,用于跳转到预约挂号表单页面。
```vue
<template>
<div id="app">
<el-container>
<el-header>预约挂号</el-header>
<el-container>
<el-aside width="200px" style="position: fixed;z-index: 1;">
<el-menu>
<el-menu-item index="1" @click="$router.push('/home')">首页</el-menu-item>
<el-menu-item index="2" @click="$router.push('/login')">用户登录</el-menu-item>
<el-menu-item index="3" @click="$router.push('/register')">用户注册</el-menu-item>
<el-menu-item index="4" @click="$router.push('/appointment')">预约挂号</el-menu-item>
<el-menu-item index="5" @click="$router.push('/doctors')">医生列表</el-menu-item>
<!-- 新增预约挂号按钮 -->
<el-menu-item index="6" @click="$router.push('/appointment/form')">预约挂号</el-menu-item>
</el-menu>
</el-aside>
<el-main>
<router-view></router-view>
</el-main>
</el-container>
<el-footer>
<div style="display: flex; justify-content: space-between;">
<span>地址:x市南泉路</span>
<span>联系电话:123456780</span>
<span id="dynamic-clock"></span>
</div>
</el-footer>
</el-container>
</div>
</template>
<script>
export default {
name: 'App',
mounted() {
this.updateClock();
setInterval(this.updateClock, 1000);
},
methods: {
updateClock() {
const now = new Date();
const timeString = now.toLocaleTimeString();
const dateString = now.toDateString();
document.getElementById('dynamic-clock').innerText = `${dateString} ${timeString}`;
}
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
}
.el-header, .el-footer {
background-color: #B3C0D1;
color: #333;
text-align: center;
line-height: 60px;
}
.el-aside {
position: fixed;
top: 60px;
bottom: 60px;
left: 0;
width: 250px;
height: 100%;
overflow-y: auto;
background-color: #D3DCE6;
color: #333;
text-align: center;
z-index: 1;
}
.el-main {
background-color: #E9EEF3;
color: #333;
text-align: center;
padding: 20px;
margin-left: 200px;
}
body > .el-container {
margin-bottom: 40px;
}
.el-container:nth-child(5) .el-aside,
.el-container:nth-child(6) .el-aside {
line-height: 260px;
}
.el-container:nth-child(7) .el-aside {
line-height: 320px;
}
</style>
```
### 2. 修改路由配置
在 `Src/router/index.js` 文件中添加新的路由规则,以便能够访问预约挂号表单页面。
```javascript
import Vue from 'vue';
import Router from 'vue-router';
import Home from '../components/Home.vue';
import Login from '../components/Login.vue';
import Register from '../components/Register.vue';
import DoctorList from '../components/DoctorList.vue';
import AppointmentForm from '../components/AppointmentForm.vue';
import AppointmentList from '../components/AppointmentList.vue';
Vue.use(Router);
export default new Router({
routes: [
{ path: '/', redirect: '/login' },
{ path: '/home', component: Home },
{ path: '/login', component: Login },
{ path: '/register', component: Register },
{ path: '/doctors', component: DoctorList },
{ path: '/appointment/:doctorId', component: AppointmentForm },
{ path: '/appointments', component: AppointmentList },
// 新增预约挂号表单路由
{ path: '/appointment/form', component: AppointmentForm }
]
});
```
### 3. 修改预约挂号表单页面
在 `Src/components/AppointmentForm.vue` 中,修改表单标题的位置,使其居中显示在顶部。
```vue
<template>
<div class="appointment-form-container">
<h2 style="text-align: center;">预约挂号</h2>
<el-form @submit.native.prevent="handleSubmit">
<el-form-item label="姓名">
<el-input v-model="form.name"></el-input>
</el-form-item>
<el-form-item label="性别">
<el-select v-model="form.gender" placeholder="请选择性别">
<el-option label="男" value="male"></el-option>
<el-option label="女" value="female"></el-option>
</el-select>
</el-form-item>
<el-form-item label="年龄">
<el-input v-model.number="form.age"></el-input>
</el-form-item>
<el-form-item label="联系电话">
<el-input v-model="form.phone"></el-input>
</el-form-item>
<el-form-item label="预约时间">
<el-date-picker v-model="form.date" type="datetime" placeholder="选择日期时间"></el-date-picker>
</el-form-item>
<el-button type="primary" native-type="submit">提交</el-button>
</el-form>
</div>
</template>
<script>
export default {
data() {
return {
form: {
name: '',
gender: '',
age: '',
phone: '',
date: ''
}
};
},
methods: {
handleSubmit() {
const appointment = { ...this.form, doctorId: this.$route.params.doctorId };
this.$store.dispatch('createAppointment', appointment);
this.$router.push('/doctors');
}
}
};
</script>
<style scoped>
.appointment-form-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.box-card {
width: 400px;
}
</style>
```
### 4. 修改提交后的跳转路径
在 `Src/components/AppointmentForm.vue` 的 `handleSubmit` 方法中,将提交成功后的跳转路径改为 `/doctors`,即医生列表页面。
```javascript
methods: {
handleSubmit() {
const appointment = { ...this.form, doctorId: this.$route.params.doctorId };
this.$store.dispatch('createAppointment', appointment);
this.$router.push('/doctors');
}
}
```
通过以上步骤,您可以实现新增预约挂号按钮并将其放在侧边栏中,点击后跳转到预约挂号表单页面,同时将表单标题居中显示在顶部,并在提交预约挂号后跳转到医生列表页面。
阅读全文
相关推荐
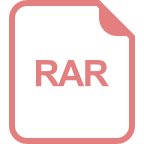
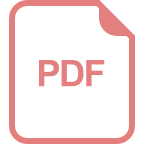
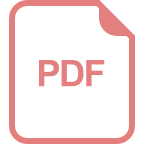
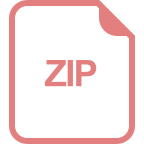
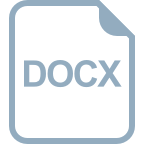
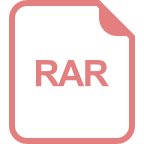
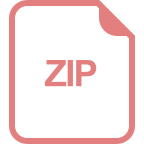
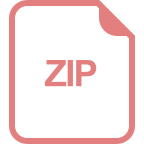
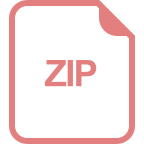
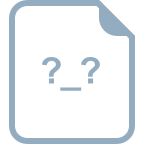
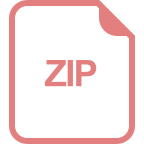
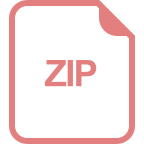
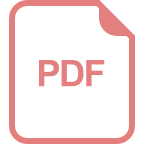
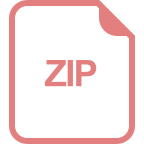
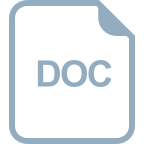