多线程——使用Thread类创建子线程,并在子线程中显示“thread is running”,使用sleep()方法挂起5s,使用notify()方法唤醒进程,然后使用stop()方法撤销。
时间: 2024-09-20 12:06:38 浏览: 62
在Java中,多线程是通过`Thread`类或实现`Runnable`接口来创建新线程的一种机制。以下是使用`Thread`类创建子线程的一个示例:
```java
public class WorkerThread extends Thread {
private String threadName;
public WorkerThread(String name) {
this.threadName = name;
}
@Override
public void run() {
System.out.println("Thread " + threadName + " is running.");
try {
// 模拟工作并暂停5秒
Thread.sleep(5000);
System.out.println("Thread " + threadName + " has finished its work and is now paused.");
} catch (InterruptedException e) {
e.printStackTrace();
}
// 使用notify()唤醒主线程或其他等待的线程
notify();
}
// 主线程可以调用此方法取消子线程
public void stopThread() {
if (this.isAlive()) {
interrupt(); // 调用Thread.interrupt()停止线程
try {
join(); // 等待子线程结束
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread " + threadName + " has been stopped.");
}
}
}
// 使用示例
public class Main {
public static void main(String[] args) {
WorkerThread worker = new WorkerThread("Main Thread");
worker.start();
// 线程A等待worker线程完成
synchronized (worker) {
while (!worker.isInterrupted()) {
try {
worker.wait(); // 当前线程进入wait状态
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
worker.stopThread(); // 如果需要,从主线程停止子线程
}
}
```
在这个例子中,子线程(`WorkerThread`)开始运行并打印出"thread is running",接着睡眠5秒。当它被中断或通知后,主线程会检查子线程是否还在运行,如果不在,就使用`stop()`方法(已废弃,这里用`interrupt()`替代)终止线程。注意,`stop()`方法在现代Java编程中通常不推荐使用,因为它可能会导致未预期的结果。
阅读全文
相关推荐







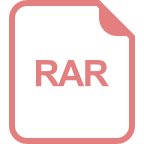


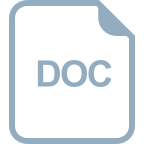

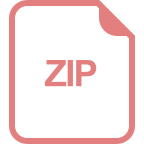


