编写一个程序,在自定义函数中完成下列功能并在主函数中进行测试:(1) 建立一个空的单链表,并初始化: (2)用尾插法往链表里插入n个数据(数据类型及个数需自己定义),然后打印出来验证。 (3) 删除单链表中元素值最大的结点(假设这样的结点唯一),然后打印出来验证。
时间: 2024-09-27 13:06:37 浏览: 56
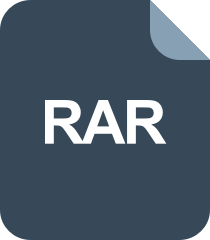
写自定义函数stringLower()实现将一个字符串中所有大写字母变为小写字母。在主函数中输入一含有大写字母的字符串,调用该函数并输出改变后的字符串。

在Python中,我们可以使用`ListNode`类来创建一个简单的单链表结构,并提供相应的操作方法,如`init_list`, `insert`, 和 `delete_max`. 首先,我们需要定义节点类和一些辅助函数:
```python
class ListNode:
def __init__(self, data=None):
self.data = data
self.next = None
def init_list(n, data_type=int):
if n <= 0:
return None
head = ListNode(data_type(0))
current = head
for _ in range(1, n):
new_node = ListNode(data_type(_))
current.next = new_node
current = new_node
return head
def insert_value(head, value, data_type=int):
new_node = ListNode(value)
current = head
while current.next is not None:
current = current.next
current.next = new_node
return head
def delete_max(head, data_type=int):
max_val = float('-inf')
max_node = None
current = head
while current is not None:
if current.data > max_val:
max_val = current.data
max_node = current
current = current.next
if max_node is not None:
max_node.data = float('-inf') # Replace with None or remove the node here
return head
else:
return head # If no max value exists, the list remains unchanged
def print_list(head):
current = head
while current is not None:
print(current.data, end=' -> ')
current = current.next
print("None")
# 测试
if __name__ == "__main__":
n = 5
custom_data_type = lambda x: chr(x + ord('a')) # 使用字符作为数据类型示例
head = init_list(n, custom_data_type)
print("Original List:")
print_list(head)
inserted_value = 'e'
head = insert_value(head, inserted_value, custom_data_type)
print("\nList after inserting:", inserted_value)
print_list(head)
deleted_head = delete_max(head, custom_data_type)
print("\nList after deleting maximum:", "None" if deleted_head.data == float('-inf') else deleted_head.data)
print_list(deleted_head)
```
这个程序首先创建一个空链表,接着插入指定数量的数据,然后删除最大值并打印链表。请注意,上述代码中的`delete_max`函数假设链表中有一个唯一的最大值,如果没有,则不会删除任何节点。
阅读全文
相关推荐
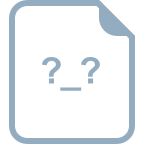
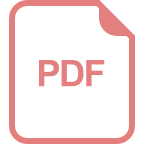















