java实现chatgpt流式接口
时间: 2023-09-11 22:08:32 浏览: 70
Java实现ChatGPT的流式接口可以使用OpenAI GPT-3的REST API进行通信。下面是一个简单的示例代码:
```java
import okhttp3.*;
import org.json.JSONObject;
import java.io.IOException;
public class ChatGPTClient {
private static final String API_ENDPOINT = "https://api.openai.com/v1/engines/davinci/completions";
private final OkHttpClient client;
private final String apiKey;
public ChatGPTClient(String apiKey) {
this.apiKey = apiKey;
this.client = new OkHttpClient();
}
public String sendMessage(String message) throws IOException {
RequestBody requestBody = new FormBody.Builder()
.add("model", "davinci")
.add("prompt", message)
.build();
Request request = new Request.Builder()
.url(API_ENDPOINT)
.header("Authorization", "Bearer " + apiKey)
.post(requestBody)
.build();
try (Response response = client.newCall(request).execute()) {
JSONObject json = new JSONObject(response.body().string());
String completions = json.getJSONArray("choices").getJSONObject(0).getString("text");
return completions;
}
}
public static void main(String[] args) {
ChatGPTClient client = new ChatGPTClient("YOUR_API_KEY");
try {
String message = "Hello!";
while (!message.equals("bye")) {
String response = client.sendMessage(message);
System.out.println("AI: " + response);
// 接收用户输入
// ...
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
上述代码使用了OkHttp库进行HTTP请求,并使用JSONObject解析响应结果。请确保你替换`YOUR_API_KEY`为你的OpenAI API密钥。
这个示例代码只是一个基本的实现,你可以根据自己的需求进行扩展和优化。
相关推荐
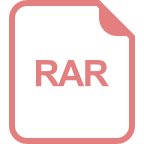
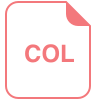
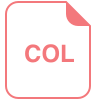











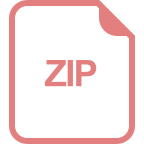