vue computed使用props
时间: 2024-07-12 10:01:41 浏览: 121
在Vue.js中,`computed`属性是一个非常有用的特性,它允许你在组件中定义一些基于数据(包括接收自父组件的`props`)的响应式计算属性。当你依赖的数据改变时,`computed`会自动更新结果,无需手动触发。
当你在子组件中使用`props`时,可以通过`this.$props.propertyName`访问从父组件传递过来的属性。例如:
```html
<template>
<div>
<!-- 在这里使用计算属性 -->
{{ message }}
</div>
</template>
<script>
export default {
name: 'ChildComponent',
computed: {
// 使用prop作为计算的基础
message: function() {
return `Hello, ${this.$props.name}!`;
}
}
}
</script>
```
在这个例子中,`message`是一个`computed`属性,它根据`name` prop动态生成内容。当`name` prop在父组件发生变化时,`childComponent`中的`message`也会相应地更新。
相关问题
vue3.0 computed的使用
在Vue3.0中,可以通过computed属性来定义计算属性。computed属性类似于一个函数,它能够根据其依赖的状态(data、props等)的变化而自动地重新计算。使用computed属性能够简化代码,提高性能。
vue3 中使用 props 数据
在Vue3中,使用props数据的方式与Vue2中有一些不同。在Vue3中,我们可以使用`setup`函数来定义组件,并通过`props`参数接收传递进来的props数据。
首先,在组件的`setup`函数中,我们可以通过参数(通常是`props`)来接收父组件传递的props数据。例如,如果我们的props中有一个名为`message`的属性,我们可以这样接收它:
```javascript
setup(props) {
// 在这里可以使用props.message
// ...
}
```
然后,我们可以在`setup`函数中使用`props`对象中的属性,就像在Vue2中使用`this.$props`一样。例如,我们可以在模板中使用它们:
```html
<template>
<div>{{ props.message }}</div>
</template>
```
或者,我们还可以在计算属性和方法中使用`props`数据:
```javascript
setup(props) {
// 计算属性
const computedData = computed(() => {
return props.message + ' computed';
});
// 方法
function handleClick() {
console.log(props.message + ' clicked');
}
return {
computedData,
handleClick
};
}
```
最后,记得在`setup`函数中返回我们希望在模板中使用的数据、计算属性和方法。
需要注意的是,在Vue3中,`props`不再是组件实例的直接属性,而是作为`setup`函数的参数传入。同时,我们也不再需要使用`data`函数来初始化`props`中的数据,而是直接使用`props`对象来访问这些数据。
希望这个解答对您有帮助!<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [vue3,使用watch监听props中的数据](https://blog.csdn.net/weixin_46683645/article/details/125481381)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [Vue基础之使用props传递数据](https://blog.csdn.net/qq_41720578/article/details/124363836)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
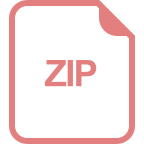
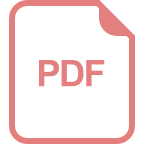
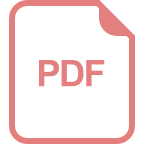












