用c语言建立一个可以多次输入学生信息的线性表
时间: 2024-10-25 10:02:50 浏览: 15
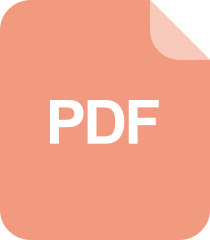
数据结构(c语言版)习题答案严蔚敏李冬梅吴伟民23490数据结构习题答案
在 C 语言中,我们可以使用结构体(struct)来创建一个表示学生的信息的数据结构,然后通过动态内存分配(如`malloc`和`realloc`)来构建可以存储多个学生数据的线性表,即链表。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
typedef struct {
char name[50];
int age;
float score; // 成绩
} Student;
// 动态内存管理函数
Student* create_student(char* name, int age, float score) {
Student* new_student = (Student*) malloc(sizeof(Student));
if (new_student != NULL) {
strcpy(new_student->name, name);
new_student->age = age;
new_student->score = score;
return new_student;
}
printf("Memory allocation failed.\n");
return NULL;
}
void add_student(Student** students_list, Student* new_student) {
*students_list = realloc(*students_list, sizeof(Student) + sizeof(Student*));
if (*students_list == NULL) {
printf("Memory reallocation failed.\n");
free(new_student);
return;
}
(*students_list)[*(strlen(*students_list) / sizeof(Student))++] = new_student;
}
// 添加学生信息并显示链表内容
int main() {
Student* students = NULL; // 初始化链表头
char name[50];
int age;
float score;
while (1) {
printf("Enter student information (type 'quit' to exit): \n");
fgets(name, sizeof(name), stdin); // 防止换行符
if (strcmp(name, "quit") == 0) break;
age = atoi(strtok(name, ","));
score = atof(strtok(NULL, ","));
Student* new_student = create_student(name, age, score);
if (new_student != NULL) {
add_student(&students, new_student);
}
}
printf("Students in the list:\n");
for (size_t i = 0; students[i] != NULL; i++) {
printf("%s, Age: %d, Score: %.2f\n", students[i]->name, students[i]->age, students[i]->score);
}
for (Student* student = students; student != NULL; student = student->next) {
free(student);
}
free(students);
return 0;
}
```
在这个程序中,用户可以不断输入学生的信息直到指定“quit”,然后程序会将每个新学生添加到链表中,并在退出时遍历整个列表显示所有学生的详情。
阅读全文
相关推荐
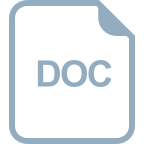
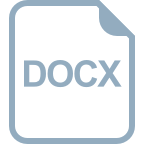
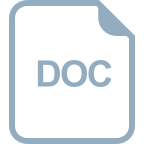
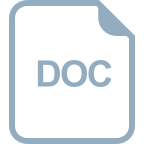
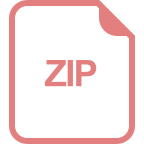
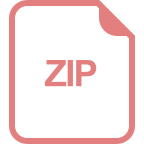
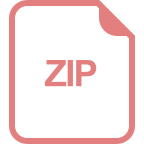
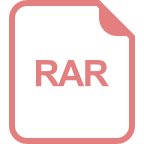
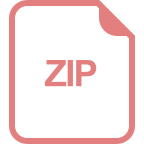
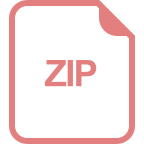
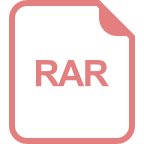
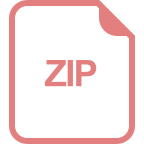
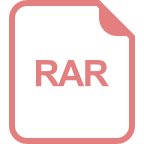