如何在Java项目中使用HttpClientUtil工具类实现RESTful API的GET和POST请求调用?请提供示例代码。
时间: 2024-11-02 20:27:26 浏览: 11
在Java项目开发中,调用RESTful API的GET和POST请求是一种常见的网络交互方式,尤其在需要获取天气预报等第三方服务数据时。使用HttpClientUtil工具类可以简化HTTP请求的发送和处理流程。以下是使用HttpClientUtil实现GET和POST请求的具体示例代码,以及相关的技术细节和操作步骤。
参考资源链接:[Java调用第三方接口实战教程](https://wenku.csdn.net/doc/6401ad20cce7214c316ee652?spm=1055.2569.3001.10343)
首先,确保你的项目中已经集成了Apache HttpClient库。接下来,你可以创建一个HttpClientUtil工具类,用于封装HTTP请求的发送逻辑。
对于GET请求,可以创建一个方法如下:
```java
public String sendGetRequest(String url, Map<String, String> queryParams) throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet(url);
URIBuilder builder = new URIBuilder(httpGet.getURI());
for (Map.Entry<String, String> entry : queryParams.entrySet()) {
builder.addParameter(entry.getKey(), entry.getValue());
}
httpGet.setURI(builder.build());
CloseableHttpResponse response = httpClient.execute(httpGet);
try {
return EntityUtils.toString(response.getEntity(), ContentType.APPLICATION_JSON.getCharset());
} finally {
response.close();
}
}
```
调用此方法时,传入API的基础URL和查询参数,然后获取返回的JSON字符串。
对于POST请求,可以创建另一个方法:
```java
public String sendPostRequest(String url, String jsonPayload) throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
StringEntity entity = new StringEntity(jsonPayload, ContentType.APPLICATION_JSON);
httpPost.setEntity(entity);
CloseableHttpResponse response = httpClient.execute(httpPost);
try {
return EntityUtils.toString(response.getEntity(), ContentType.APPLICATION_JSON.getCharset());
} finally {
response.close();
}
}
```
调用此方法时,传入API的基础URL和要发送的JSON数据负载。
使用这两个方法,你可以轻松地在Java项目中调用RESTful API,获取数据或执行操作。例如,获取天气预报信息:
```java
String weatherUrl =
参考资源链接:[Java调用第三方接口实战教程](https://wenku.csdn.net/doc/6401ad20cce7214c316ee652?spm=1055.2569.3001.10343)
阅读全文
相关推荐
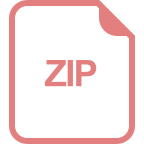
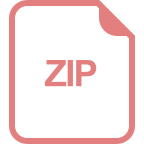
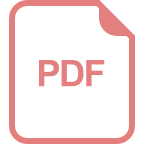
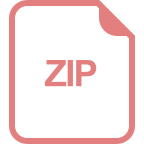
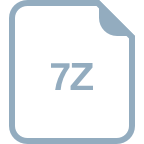
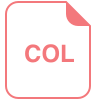
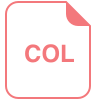
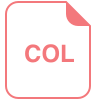
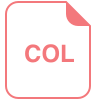
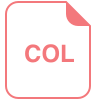
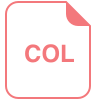
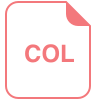
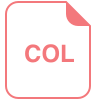
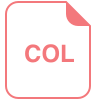
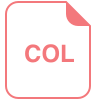
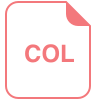
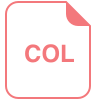
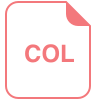