嵌入式Linux多线程管道怎么使用
时间: 2024-05-06 12:21:57 浏览: 48
嵌入式Linux多线程管道的使用步骤如下:
1. 创建管道:使用pipe()函数创建一个管道。该函数返回两个文件描述符,一个用于读取管道中的数据,一个用于写入管道中的数据。
2. 创建多个线程:使用pthread_create()函数创建多个线程。每个线程都可以使用管道的写入文件描述符向管道中写入数据。
3. 在主线程中读取管道中的数据:使用管道的读取文件描述符从管道中读取数据。可以使用select()或poll()函数来检查管道是否有数据可读,以避免阻塞主线程。
4. 在多个线程中向管道中写入数据:在每个线程中,使用管道的写入文件描述符向管道中写入数据。可以使用write()函数向管道中写入数据。
示例代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
#include <errno.h>
#define PIPE_SIZE 4096
int pipe_fd[2];
void *thread_func(void *arg)
{
char buf[PIPE_SIZE];
int ret;
sprintf(buf, "Data from thread %d", *(int *)arg);
ret = write(pipe_fd[1], buf, PIPE_SIZE);
if (ret == -1) {
perror("write() failed");
exit(EXIT_FAILURE);
}
pthread_exit(NULL);
}
int main()
{
pthread_t tid[5];
int i, ret;
char buf[PIPE_SIZE];
ret = pipe(pipe_fd);
if (ret == -1) {
perror("pipe() failed");
exit(EXIT_FAILURE);
}
for (i = 0; i < 5; i++) {
ret = pthread_create(&tid[i], NULL, thread_func, &i);
if (ret != 0) {
perror("pthread_create() failed");
exit(EXIT_FAILURE);
}
}
for (i = 0; i < 5; i++) {
ret = read(pipe_fd[0], buf, PIPE_SIZE);
if (ret == -1) {
perror("read() failed");
exit(EXIT_FAILURE);
}
printf("%s\n", buf);
}
return 0;
}
```
该示例代码创建了一个管道,然后创建了5个线程,每个线程向管道中写入一条数据。最后,在主线程中从管道中读取5条数据并输出。
阅读全文
相关推荐
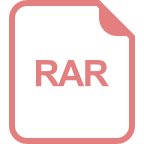
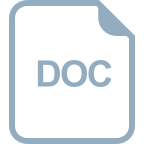
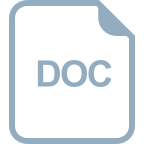

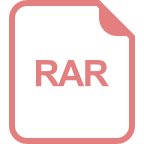
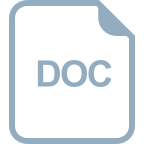
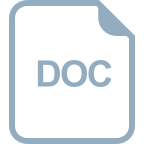
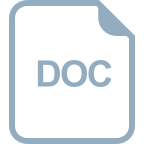
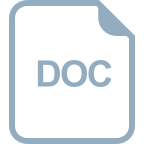
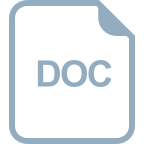
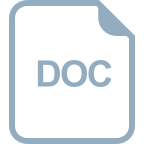
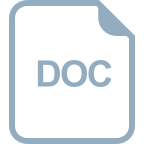

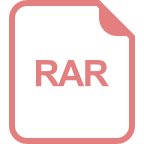
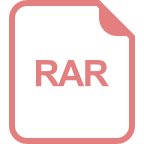
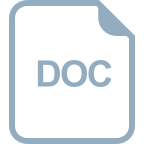